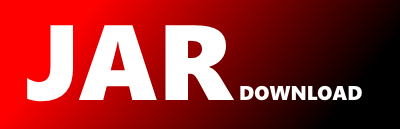
edu.berkeley.nlp.util.MutableDouble Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of berkeleyparser Show documentation
Show all versions of berkeleyparser Show documentation
The Berkeley parser analyzes the grammatical structure of natural language using probabilistic context-free grammars (PCFGs).
The newest version!
package edu.berkeley.nlp.util;
/**
* A class for Double objects that you can change.
*
* @author Dan Klein
*/
public final class MutableDouble extends Number implements Comparable {
private double d;
// Mutable
public void set(double d) {
this.d = d;
}
public int hashCode() {
long bits = Double.doubleToLongBits(d);
return (int) (bits ^ (bits >>> 32));
}
/**
* Compares this object to the specified object. The result is
* true
if and only if the argument is not
* null
and is an MutableDouble
object that
* contains the same double
value as this object.
* Note that a MutableDouble isn't and can't be equal to an Double.
*
* @param obj the object to compare with.
* @return true
if the objects are the same;
* false
otherwise.
*/
public boolean equals(Object obj) {
if (obj instanceof MutableDouble) {
return d == ((MutableDouble) obj).d;
}
return false;
}
public String toString() {
return Double.toString(d);
}
// Comparable interface
/**
* Compares two MutableDouble
objects numerically.
*
* @param anotherMutableDouble the MutableDouble
to be
* compared.
* @return Tthe value 0
if this MutableDouble
is
* equal to the argument MutableDouble
; a value less than
* 0
if this MutableDouble
is numerically less
* than the argument MutableDouble
; and a value greater
* than 0
if this MutableDouble
is numerically
* greater than the argument MutableDouble
(signed
* comparison).
*/
public int compareTo(MutableDouble anotherMutableDouble) {
double thisVal = this.d;
double anotherVal = anotherMutableDouble.d;
return (thisVal < anotherVal ? -1 : (thisVal == anotherVal ? 0 : 1));
}
/**
* Compares this MutableDouble
object to another object.
* If the object is an MutableDouble
, this function behaves
* like compareTo(MutableDouble)
. Otherwise, it throws a
* ClassCastException
(as MutableDouble
* objects are only comparable to other MutableDouble
* objects).
*
* @param o the Object
to be compared.
* @return 0/-1/1
* @throws ClassCastException
* if the argument is not an
* MutableDouble
.
* @see java.lang.Comparable
*/
public int compareTo(Object o) {
return compareTo((MutableDouble) o);
}
// Number interface
public int intValue() {
return (int) d;
}
public long longValue() {
return (long) d;
}
public short shortValue() {
return (short) d;
}
public byte byteValue() {
return (byte) d;
}
public float floatValue() {
return (float) d;
}
public double doubleValue() {
return d;
}
public MutableDouble() {
this(0);
}
public MutableDouble(double d) {
this.d = d;
}
public double increment(double inc) {
this.d += inc;
return d;
}
private static final long serialVersionUID = 624465615824626762L;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy