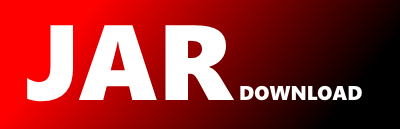
edu.berkeley.nlp.util.MutableInteger Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of berkeleyparser Show documentation
Show all versions of berkeleyparser Show documentation
The Berkeley parser analyzes the grammatical structure of natural language using probabilistic context-free grammars (PCFGs).
The newest version!
package edu.berkeley.nlp.util;
/**
* A class for Integer objects that you can change.
*
* @author Dan Klein
*/
public final class MutableInteger extends Number implements Comparable {
private int i;
// Mutable
public void set(int i) {
this.i = i;
}
public int hashCode() {
return i;
}
/**
* Compares this object to the specified object. The result is
* true
if and only if the argument is not
* null
and is an MutableInteger
object that
* contains the same int
value as this object.
* Note that a MutableInteger isn't and can't be equal to an Integer.
*
* @param obj the object to compare with.
* @return true
if the objects are the same;
* false
otherwise.
*/
public boolean equals(Object obj) {
if (obj instanceof MutableInteger) {
return i == ((MutableInteger) obj).i;
}
return false;
}
public String toString() {
return Integer.toString(i);
}
// Comparable interface
/**
* Compares two MutableInteger
objects numerically.
*
* @param anotherMutableInteger the MutableInteger
to be
* compared.
* @return Tthe value 0
if this MutableInteger
is
* equal to the argument MutableInteger
; a value less than
* 0
if this MutableInteger
is numerically less
* than the argument MutableInteger
; and a value greater
* than 0
if this MutableInteger
is numerically
* greater than the argument MutableInteger
(signed
* comparison).
*/
public int compareTo(MutableInteger anotherMutableInteger) {
int thisVal = this.i;
int anotherVal = anotherMutableInteger.i;
return (thisVal < anotherVal ? -1 : (thisVal == anotherVal ? 0 : 1));
}
/**
* Compares this MutableInteger
object to another object.
* If the object is an MutableInteger
, this function behaves
* like compareTo(MutableInteger)
. Otherwise, it throws a
* ClassCastException
(as MutableInteger
* objects are only comparable to other MutableInteger
* objects).
*
* @param o the Object
to be compared.
* @return 0/-1/1
* @throws ClassCastException
* if the argument is not an
* MutableInteger
.
* @see java.lang.Comparable
*/
public int compareTo(Object o) {
return compareTo((MutableInteger) o);
}
// Number interface
public int intValue() {
return i;
}
public long longValue() {
return (long) i;
}
public short shortValue() {
return (short) i;
}
public byte byteValue() {
return (byte) i;
}
public float floatValue() {
return (float) i;
}
public double doubleValue() {
return (double) i;
}
public MutableInteger() {
this(0);
}
public MutableInteger(int i) {
this.i = i;
}
private static final long serialVersionUID = 624465615824626762L;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy