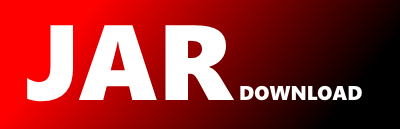
edu.berkeley.nlp.util.StopWatchSet Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of berkeleyparser Show documentation
Show all versions of berkeleyparser Show documentation
The Berkeley parser analyzes the grammatical structure of natural language using probabilistic context-free grammars (PCFGs).
The newest version!
package edu.berkeley.nlp.util;
import java.util.*;
import java.lang.ThreadLocal;
/**
* 4/2/09: StopWatchSet should be re-entrant (can call begin("foo") twice) and thread-safe.
*/
public class StopWatchSet {
// For measuring time of certain types of events.
// Shared across all threads.
private static Map stopWatches = new LinkedHashMap();
// A stack of stop-watches (one per thread)
private static ThreadLocal>> lastStopWatches = new ThreadLocal() {
protected LinkedList> initialValue() { return new LinkedList(); }
};
public synchronized static StopWatch getWatch(String s) {
return MapUtils.getMut(stopWatches, s, new StopWatch());
}
public static void begin(String s) {
// Create a new stop watch for reentrance and thread safety
lastStopWatches.get().addLast(new Pair(s, new StopWatch().start()));
}
public static void end() {
Pair pair = lastStopWatches.get().removeLast();
pair.getSecond().stop();
// Add it
synchronized(stopWatches) {
getWatch(pair.getFirst()).add(pair.getSecond());
}
}
public synchronized static OrderedStringMap getStats() {
OrderedStringMap map = new OrderedStringMap();
for(String key : stopWatches.keySet()) {
StopWatch watch = getWatch(key);
map.put(key, watch + " (" + new StopWatch(watch.n == 0 ? 0 : watch.ms/watch.n) + " x " + watch.n + ")");
}
return map;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy