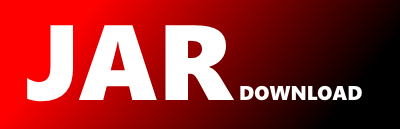
edu.berkeley.nlp.util.T2DoubleMap Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of berkeleyparser Show documentation
Show all versions of berkeleyparser Show documentation
The Berkeley parser analyzes the grammatical structure of natural language using probabilistic context-free grammars (PCFGs).
The newest version!
package edu.berkeley.nlp.util;
import static edu.berkeley.nlp.util.LogInfo.*;
import java.io.*;
import java.util.*;
/** Maps (object, object) pairs to doubles.
* Based on TDoubleMap.
* It's useful when the number of second objects for a fixed first string
* is small.
* Most of the operations in this class parallel that of TDoubleMap,
* but just applied to two keys. The implementation is essentially dispatching
* down to TDoubleMap.
* Typical usage: conditional probability table.
*/
public class T2DoubleMap extends AbstractT2Map implements Iterable>>, Serializable {
protected static final long serialVersionUID = 42;
public T2DoubleMap() {
this.keyFunc = AbstractTMap.defaultFunctionality;
}
public T2DoubleMap(AbstractTMap.Functionality keyFunc) {
this.keyFunc = keyFunc;
}
public void initKeys(AbstractT2Map map) {
this.locked = map.locked;
// HACK: BAD dependencies
if(map instanceof T2DoubleMap) {
for(Map.Entry> e : (T2DoubleMap)map)
put(e.getKey(), new TDoubleMap(e.getValue()));
}
else if(map instanceof T2VMap) { // Not exactly right: need to check type of V
for(Map.Entry> e : ((T2VMap)map))
put(e.getKey(), new TDoubleMap(e.getValue()));
}
else
throw new RuntimeException("");
}
// Main operations
public boolean containsKey(S key1, T key2) {
TDoubleMap map = getMap(key1, false);
return map != null && map.containsKey(key2);
}
public double get(S key1, T key2, double defaultValue) {
TDoubleMap map = getMap(key1, false);
return map == null ? defaultValue : map.get(key2, defaultValue);
}
public double getWithErrorMsg(S key1, T key2, double defaultValue) {
TDoubleMap map = getMap(key1, false);
if(map == null) errors("(%s, %s) not in map, using %f", key1, key2, defaultValue);
return map == null ? defaultValue : map.get(key2, defaultValue);
}
public double getSure(S key1, T key2) {
// Throw exception if key doesn't exist.
TDoubleMap map = getMap(key1, false);
if(map == null) throw new RuntimeException("Missing key: " + key1);
return map.getSure(key2);
}
public void put(S key1, TDoubleMap map) { // Risky
if(locked)
throw new RuntimeException("Cannot make new entry for " + key1 + ", because map is locked");
maps.put(key1, map);
}
public void put(S key1, T key2, double value) {
TDoubleMap map = getMap(key1, true);
map.put(key2, value);
}
public void incr(S key1, T key2, double dValue) {
TDoubleMap map = getMap(key1, true);
map.incr(key2, dValue);
}
public int size() { return maps.size(); }
// Return number of entries
public int totalSize() {
int n = 0;
for(TDoubleMap map : maps.values())
n += map.size();
return n;
}
public void gut() {
for(TDoubleMap map : maps.values())
map.gut();
}
public Iterator>> iterator() {
return maps.entrySet().iterator();
}
public Set>> entrySet() { return maps.entrySet(); }
public Set keySet() { return maps.keySet(); }
public Collection> values() { return maps.values(); }
// If keys are locked, we can share the same keys.
public T2DoubleMap copy() {
return copy(newMap());
}
public T2DoubleMap copy(T2DoubleMap newMap) {
newMap.locked = locked;
for(Map.Entry> e : maps.entrySet())
newMap.maps.put(e.getKey(), e.getValue().copy());
return newMap;
}
public T2DoubleMap restrict(Set set1, Set set2) {
return restrict(newMap(), set1, set2);
}
public T2DoubleMap restrict(T2DoubleMap newMap, Set set1, Set set2) {
newMap.locked = locked;
for(Map.Entry> e : maps.entrySet())
if(set1.contains(e.getKey()))
newMap.maps.put(e.getKey(), e.getValue().restrict(set2));
return newMap;
}
public T2DoubleMap reverse(T2DoubleMap newMap) { // Return a map with (key2, key1) pairs
for(Map.Entry> e1 : maps.entrySet()) {
S key1 = e1.getKey();
TDoubleMap map = e1.getValue();
for(TDoubleMap.Entry e2 : map) {
T key2 = e2.getKey();
double value = e2.getValue();
newMap.put(key2, key1, value);
}
}
return newMap;
}
public void lock() {
for(TDoubleMap map : maps.values())
map.lock();
}
public void switchToSortedList() {
for(TDoubleMap map : maps.values())
map.switchToSortedList();
}
public void switchToHashTable() {
for(TDoubleMap map : maps.values())
map.switchToHashTable();
}
protected T2DoubleMap newMap() { return new T2DoubleMap(keyFunc); }
////////////////////////////////////////////////////////////
public TDoubleMap getMap(S key1, boolean modify) {
if(key1 == lastKey) return lastMap;
TDoubleMap map = maps.get(key1);
if(map != null) return map;
if(modify) {
if(locked)
throw new RuntimeException("Cannot make new entry for " + key1 + ", because map is locked");
maps.put(key1, map = new TDoubleMap(keyFunc));
lastKey = key1;
lastMap = map;
return map;
}
else
return null;
}
////////////////////////////////////////////////////////////
private Map> maps = new HashMap>();
private S lastKey; // Cache last access
private TDoubleMap lastMap; // Cache last access
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy