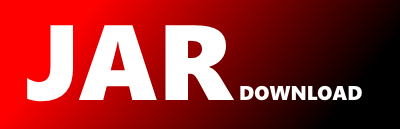
edu.berkeley.nlp.util.functional.FunctionalUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of berkeleyparser Show documentation
Show all versions of berkeleyparser Show documentation
The Berkeley parser analyzes the grammatical structure of natural language using probabilistic context-free grammars (PCFGs).
The newest version!
package edu.berkeley.nlp.util.functional;
import edu.berkeley.nlp.util.CollectionUtils;
import edu.berkeley.nlp.util.Factory;
import edu.berkeley.nlp.util.LazyIterable;
import edu.berkeley.nlp.util.Pair;
import java.lang.reflect.Field;
import java.lang.reflect.Method;
import java.util.*;
/**
* Collection of Functional Utilities you'd
* find in any functional programming language.
* Things like map, filter, reduce, etc..
*
* Created by IntelliJ IDEA.
* User: aria42
* Date: Oct 7, 2008
* Time: 1:06:08 PM
*/
public class FunctionalUtils {
public static List take(Iterator it, int n) {
List result = new ArrayList();
for (int i=0; i < n && it.hasNext(); ++i) {
result.add(it.next());
}
return result;
}
private static Method getMethod(Class c, String field) {
Method[] methods = c.getDeclaredMethods();
String trgMethName = "get" + field;
Method trgMeth = null;
for (Method m: methods) {
if (m.getName().equalsIgnoreCase(trgMethName) || m.getName().equalsIgnoreCase(field)) {
return m;
}
}
return null;
}
private static Field getField(Class c, String fieldName) {
Field[] fields = c.getDeclaredFields();
for (Field f: fields) {
if (f.getName().equalsIgnoreCase(fieldName)) {
return f;
}
}
return null;
}
public static Pair findMax(Iterable xs, Function fn) {
double max = Double.NEGATIVE_INFINITY;
T argMax = null;
for (T x : xs) {
double val = fn.apply(x);
if (val > max) { max = val ; argMax = x; }
}
return Pair.newPair(argMax,max);
}
public static Pair findMin(Iterable xs, Function fn) {
double min= Double.POSITIVE_INFINITY;
T argMin = null;
for (T x : xs) {
double val = fn.apply(x);
if (val < min) { min= val ; argMin = x; }
}
return Pair.newPair(argMin,min);
}
public static Map compose(Map map, Function fn) {
return map(map,fn, (Predicate) Predicates.getTruePredicate(),new HashMap());
}
public static Map compose(Map map, Function fn, Predicate pred) {
return map(map,fn,pred,new HashMap());
}
public static List make(Factory factory, int k ) {
List insts = new ArrayList();
for (int i = 0; i < k; i++) {
insts.add(factory.newInstance());
}
// Fuck you cvs
return insts;
}
public static Map map(Map map, Function fn, Predicate pred, Map resultMap) {
for (Map.Entry entry: map.entrySet()) {
K key = entry.getKey();
I inter = entry.getValue();
if (pred.apply(key)) resultMap.put(key, fn.apply(inter));
}
return resultMap;
}
public static Map mapPairs(Iterable lst, Function fn)
{
return mapPairs(lst,fn,new HashMap());
}
public static Map mapPairs(Iterable lst, Function fn, Map resultMap)
{
for (I input: lst) {
O output = fn.apply(input);
resultMap.put(input,output);
}
return resultMap;
}
public static List map(Iterable lst, Function fn) {
return map(lst,fn,(Predicate) Predicates.getTruePredicate());
}
public static Iterable lazyMap(Iterable lst, Function fn) {
return lazyMap(lst,fn,(Predicate) Predicates.getTruePredicate());
}
public static Iterable lazyMap(Iterable lst, Function fn, Predicate pred) {
return new LazyIterable(lst,fn,pred,20);
}
public static List flatMap(Iterable lst,
Function> fn) {
Predicate> p = Predicates.getTruePredicate();
return flatMap(lst,fn,p);
}
public static List flatMap(Iterable lst,
Function> fn,
Predicate> pred) {
List> lstOfLsts = map(lst,fn,pred);
List init = new ArrayList();
return reduce(lstOfLsts, init,
new Function, List>, List>() {
public List apply(Pair, List> input) {
List result = input.getFirst();
result.addAll(input.getSecond());
return result;
}
});
}
public static O reduce(Iterable inputs,
O initial,
Function,O> fn) {
O output = initial;
for (I input: inputs) {
output = fn.apply(Pair.newPair(output,input));
}
return output;
}
public static List map(Iterable lst, Function fn, Predicate pred) {
List outputs = new ArrayList();
for (I input: lst) {
O output = fn.apply(input);
if (pred.apply(output)) {
outputs.add(output);
}
}
return outputs;
}
public static List filter(final Iterable lst, final Predicate pred) {
List ret = new ArrayList();
for (I input : lst) {
if (pred.apply(input)) ret.add(input);
}
return ret;
}
public static Function getAccessor(String field, Class c) {
final Method trgMeth = getMethod(c, field);
final Field trgField = getField(c, field);
if (trgMeth == null && trgField == null) {
throw new RuntimeException("Couldn't find field or method to access " + field);
}
return new Function() {
public T apply(O input) {
try {
return (T) (trgMeth != null ? trgMeth.invoke(input) : trgField.get(input));
} catch (Exception e) {
e.printStackTrace();
throw new RuntimeException("Error accessing Method or target");
}
}
};
}
public static Map>
groupBy(Iterable objs, Function groupFn) {
return groupBy(objs,groupFn, new Factory>() {
public Collection newInstance(Object... args) {
return new ArrayList();
}
});
}
public static Map> groupBy(Iterable objs, String field) {
return groupBy(objs,getAccessor(field,objs.iterator().next().getClass()));
}
/**
* Groups objs
by the field field
. Tries
* to find public method getField, ignoring case, then to directly
* access the field if that fails.
* @param objs
* @param field
* @return
*/
public static> Map
groupBy(Iterable objs, Function groupFn, final Factory fact) {
Iterator it = objs.iterator();
if (!it.hasNext()) return new HashMap();
Map map = new HashMap();
for (O obj: objs) {
K key = null;
try {
key = (K) groupFn.apply(obj);
} catch (Exception e) {
e.printStackTrace();
return null;
}
CollectionUtils.addToValueCollection(map,key,obj, fact);
}
return map;
}
public static T first(Iterable objs, Predicate pred) {
for (T obj : objs) {
if (pred.apply(obj)) return obj;
}
return null;
}
public static List filter(Iterable coll, final String field, final K value) throws Exception {
Iterator it = coll.iterator();
if (!it.hasNext()) return new ArrayList();
Class c = it.next().getClass();
final Method m = getMethod(c,field);
final Field f = getField(c,field);
return filter(coll, new Predicate() {
public Boolean apply(O input) {
try {
K inputVal = (K)(m != null ? m.invoke(input) : f.get(input));
return inputVal.equals(value);
} catch (Exception e) { }
return false;
}
});
}
public static List range(int n) {
List result = new ArrayList();
for (int i = 0; i < n; i++) {
result.add(i);
}
return result;
}
/**
* Testing Purposes
*/
private static class Person {
public String prefix ;
public String name;
public Person(String name) {
this.name = name;
this.prefix = name.substring(0,3);
}
public String toString() { return "Person(" + name + ")"; }
}
public static T find(Iterable elems, Predicate pred) {
for (T elem : elems) {
if (pred.apply(elem)) return elem;
}
return null;
}
public static void main(String[] args) throws Exception {
List objs = CollectionUtils.makeList(
new Person("david"),
new Person("davs"),
new Person("maria"),
new Person("marshia")
);
Map> grouped = groupBy(objs,getAccessor("prefix",Person.class));
System.out.printf("groupd: %s",grouped);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy