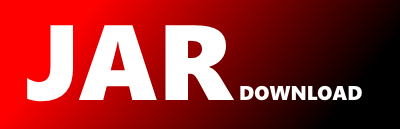
edu.berkeley.nlp.util.functional.Predicates Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of berkeleyparser Show documentation
Show all versions of berkeleyparser Show documentation
The Berkeley parser analyzes the grammatical structure of natural language using probabilistic context-free grammars (PCFGs).
The newest version!
package edu.berkeley.nlp.util.functional;
/**
* Created by IntelliJ IDEA.
* User: aria42
* Date: Oct 9, 2008
* Time: 6:32:13 PM
*/
public class Predicates {
public static Predicate getTruePredicate() {
return new Predicate() {
public Boolean apply(I input) {
return true;
}
};
}
public static Predicate getInversePredicate(final Predicate pred) {
return new Predicate() {
public Boolean apply(I input) {
return !pred.apply(input);
}
};
}
public static Predicate getOrPredicate(final Predicate...preds) {
return new Predicate() {
public Boolean apply(I input) {
for (Predicate pred: preds) {
if (pred.apply(input)) return true;
}
return false;
}
};
}
public static Predicate getNonNullPredicate() {
return new Predicate() {
public Boolean apply(Object input) {
return input != null;
}
};
}
public static Predicate getAndPredicate(final Predicate...preds) {
return new Predicate() {
public Boolean apply(I input) {
for (Predicate pred: preds) {
if (!pred.apply(input)) return false;
}
return true;
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy