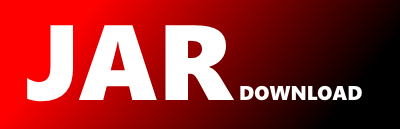
burlap.behavior.singleagent.interfaces.rlglue.RLGlueState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of burlap Show documentation
Show all versions of burlap Show documentation
The Brown-UMBC Reinforcement Learning and Planning (BURLAP) Java code library is for the use and
development of single or multi-agent planning and learning algorithms and domains to accompany them. The library
uses a highly flexible state/observation representation where you define states with your own Java classes, enabling
support for domains that discrete, continuous, relational, or anything else. Planning and learning algorithms range from classic forward search
planning to value-function-based stochastic planning and learning algorithms.
The newest version!
package burlap.behavior.singleagent.interfaces.rlglue;
import burlap.mdp.core.state.State;
import org.rlcommunity.rlglue.codec.types.Observation;
import java.util.ArrayList;
import java.util.List;
/**
* A {@link State} for RLGLue {@link Observation} objects. Each instance contains the inner RLGlue {@link Observation}
* You can set and get the {@link Observation} with standard methods, thereby allowing serialization. The BURLAP
* variable keys are specified with the {@link RLGlueVarKey}, which indicates indices for character, integer, and double
* values that RLGlue supports. You can also get variable values using String keys of the form "cn", "in", "dn" for character
* integer, and double variables respectively, where n is the index into that vector.
* @author James MacGlashan.
*/
public class RLGlueState implements State{
protected Observation obs;
public RLGlueState() {
}
public RLGlueState(Observation obs) {
this.obs = obs;
}
@Override
public List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy