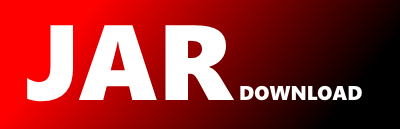
burlap.datastructures.HashedAggregator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of burlap Show documentation
Show all versions of burlap Show documentation
The Brown-UMBC Reinforcement Learning and Planning (BURLAP) Java code library is for the use and
development of single or multi-agent planning and learning algorithms and domains to accompany them. The library
uses a highly flexible state/observation representation where you define states with your own Java classes, enabling
support for domains that discrete, continuous, relational, or anything else. Planning and learning algorithms range from classic forward search
planning to value-function-based stochastic planning and learning algorithms.
The newest version!
package burlap.datastructures;
import java.util.Collection;
import java.util.HashMap;
import java.util.Map;
import java.util.Set;
/**
* Datasturcutre for maintaining numeric aggregated values for different keys. If the value has never been queried or added to before,
* then it assumes an initial value (zero by default). The values are backed by a HashMap.
* @author James MacGlashan
*
* @param the key, which must be hashable.
*/
public class HashedAggregator {
/**
* The backing hash map
*/
protected HashMap storage;
/**
* The initial value for each key
*/
protected double initialValue = 0.;
/**
* Initializes with initial value for each key being 0.0.
*/
public HashedAggregator(){
this.storage = new HashMap();
}
/**
* Initializes with the given initial value for each key.
* @param initialValue the initial value associated with each key.
*/
public HashedAggregator(double initialValue){
this.storage = new HashMap();
this.initialValue = initialValue;
}
/**
* Initializes with the given initial value for each key and the initial capacity for the table.
* @param initialValue the initial value associated with each key.
* @param capacity the initial memory capacity of the storing table
*/
public HashedAggregator(double initialValue, int capacity){
this.storage = new HashMap(capacity);
this.initialValue = initialValue;
}
/**
* Adds a specified value to a key. If the key has not been index previously, its new value is this object's initialValue datamember + v.
* @param ind the key index
* @param v the value to add to the value associated with ind.
*/
public void add(K ind, double v){
Double cur = storage.get(ind);
double c = cur != null ? cur : initialValue;
this.storage.put(ind, c+v);
}
/**
* Forces the value for an entry
* @param ind the key index
* @param v the value to be associated with the key index
*/
public void set(K ind, double v){
this.storage.put(ind, v);
}
/**
* The current value associated with key ind. This object's initialValue datamember is returned if nothing has been added to this value previously.
* @param ind the key index
* @return the value associated with the key index.
*/
public double v(K ind){
Double cur = storage.get(ind);
double c = cur != null ? cur : initialValue;
return c;
}
/**
* Returns the number of keys stored.
* @return the number of keys stored.
*/
public int size(){
return storage.size();
}
/**
* The set of keys stored.
* @return The set of keys stored.
*/
public Set keySet(){
return this.storage.keySet();
}
/**
* The set of values stored.
* @return The set of values stored.
*/
public Collection valueSet(){
return this.storage.values();
}
/**
* The entry set for stored keys and values.
* @return The entry set for stored keys and values.
*/
public Set> entrySet(){
return this.storage.entrySet();
}
/**
* Returns the HashMap that backs this object.
* @return the HashMap that backs this object.
*/
public Map getHashMap(){
return this.storage;
}
/**
* Returns true if this object has a value associated with the specified key, false otherwise.
* @param key the key to check
* @return true if this object has a value associated with the specified key, false otherwise.
*/
public boolean containsKey(K key){
return this.storage.containsKey(key);
}
/**
* Removes the entry for the given key
* @param key the key of the entry to remove
*/
public void remove(K key){
this.storage.remove(key);
}
/**
* Clears the entries
*/
public void clear(){
this.storage.clear();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy