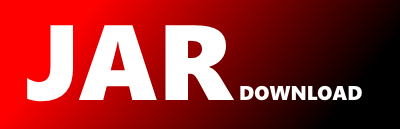
burlap.mdp.core.state.State Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of burlap Show documentation
Show all versions of burlap Show documentation
The Brown-UMBC Reinforcement Learning and Planning (BURLAP) Java code library is for the use and
development of single or multi-agent planning and learning algorithms and domains to accompany them. The library
uses a highly flexible state/observation representation where you define states with your own Java classes, enabling
support for domains that discrete, continuous, relational, or anything else. Planning and learning algorithms range from classic forward search
planning to value-function-based stochastic planning and learning algorithms.
The newest version!
package burlap.mdp.core.state;
import burlap.mdp.core.state.annotations.DeepCopyState;
import burlap.mdp.core.state.annotations.ShallowCopyState;
import java.util.List;
/**
* A State instance is used to define the state of an environment or an observation from the environment. Creating
* a state requires implementing three methods to ensure compatibility with all BURLAP tools. First, the
* {@link #variableKeys()} method should return a list of objects that represent the possible keys that are used
* to reference state variables in your state. In general, a state variable is any value that when changed alters
* the identification of the state. That is, two states with different state variables would not be equal and transition
* dynamics and reward functions from them may be different as a result.
*
* Next the {@link #get(Object)} method should accept any argument that is listed in the {@link #variableKeys()} list
* and should return the value of that variable. You do *not* have to guarantee that changes the client makes to the
* returned value will affect the state.
*
* Finally, the {@link #copy()} method must be implemented so that a copy of this state can be created.
* State copy operations are often performed when generating state transitions, or when a copy of the information
* needs to be held by some other data structure. The copy may be a shallow copy
* or deep copy and is domain/implementation specific. For clarity, the State implementation may indicate its copy mode with the
* {@link DeepCopyState} or {@link ShallowCopyState} annotations. If it is a shallow copy, you should not *directly*
* modify any fields of a copied state without copying the fields first, or it could contaminate the state from
* which the copy was made. Alternatively, use the {@link MutableState#set(Object, Object)} method to modify
* {@link ShallowCopyState} copied states,
* which for {@link ShallowCopyState} instances should perform a safe copy-on-write operation.
*
* @author James MacGlashan
*
*/
public interface State {
/**
* Returns the list of state variable keys.
* @return the list of state variable keys.
*/
List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy