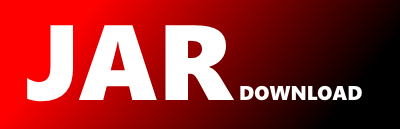
main.java.burlap.behavior.functionapproximation.dense.PFFeatures Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of burlap Show documentation
Show all versions of burlap Show documentation
The Brown-UMBC Reinforcement Learning and Planning (BURLAP) Java code library is for the use and
development of single or multi-agent planning and learning algorithms and domains to accompany them. The library
uses a highly flexible state/observation representation where you define states with your own Java classes, enabling
support for domains that discrete, continuous, relational, or anything else. Planning and learning algorithms range from classic forward search
planning to value-function-based stochastic planning and learning algorithms.
The newest version!
package burlap.behavior.functionapproximation.dense;
import burlap.mdp.core.oo.OODomain;
import burlap.mdp.core.oo.propositional.GroundedProp;
import burlap.mdp.core.oo.propositional.PropositionalFunction;
import burlap.mdp.core.oo.state.OOState;
import burlap.mdp.core.state.State;
import java.util.LinkedList;
import java.util.List;
/**
* Binary features that are determined from a list of {@link PropositionalFunction}s. The element for a corresponding
* {@link PropositionalFunction} is set to 1, when any possible binding for the {@link PropositionalFunction} is true
* for the input state.
*/
public class PFFeatures implements DenseStateFeatures {
protected PropositionalFunction [] pfsToUse;
/**
* Initializes using all propositional functions that belong to the domain
* @param domain the domain containing all the propositional functions to use
*/
public PFFeatures(OODomain domain){
this.pfsToUse = new PropositionalFunction[domain.propFunctions().size()];
int i = 0;
for(PropositionalFunction pf : domain.propFunctions()){
this.pfsToUse[i] = pf;
i++;
}
}
/**
* Initializes using the list of given propositional functions.
* @param pfs the propositional functions to use.
*/
public PFFeatures(List pfs){
this.pfsToUse = new PropositionalFunction[pfs.size()];
this.pfsToUse = pfs.toArray(this.pfsToUse);
}
/**
* Initializes using the array of given propositional functions.
* @param pfs the propositional functions to use.
*/
public PFFeatures(PropositionalFunction [] pfs){
this.pfsToUse = pfs.clone();
}
@Override
public double[] features(State s) {
List featureValueList = new LinkedList();
for(PropositionalFunction pf : this.pfsToUse){
//List gps = s.getAllGroundedPropsFor(pf);
List gps = pf.allGroundings((OOState)s);
for(GroundedProp gp : gps){
if(gp.isTrue((OOState)s)){
featureValueList.add(1.);
}
else{
featureValueList.add(0.);
}
}
}
double [] fv = new double[featureValueList.size()];
int i = 0;
for(double f : featureValueList){
fv[i] = f;
i++;
}
return fv;
}
@Override
public DenseStateFeatures copy() {
return new PFFeatures(this.pfsToUse.clone());
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy