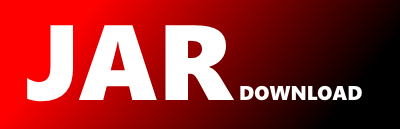
main.java.burlap.behavior.singleagent.auxiliary.gridset.FlatStateGridder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of burlap Show documentation
Show all versions of burlap Show documentation
The Brown-UMBC Reinforcement Learning and Planning (BURLAP) Java code library is for the use and
development of single or multi-agent planning and learning algorithms and domains to accompany them. The library
uses a highly flexible state/observation representation where you define states with your own Java classes, enabling
support for domains that discrete, continuous, relational, or anything else. Planning and learning algorithms range from classic forward search
planning to value-function-based stochastic planning and learning algorithms.
The newest version!
package burlap.behavior.singleagent.auxiliary.gridset;
import burlap.mdp.core.state.MutableState;
import burlap.mdp.core.state.State;
import java.util.*;
/**
* This class is used to generate a set of continuous states that are spaced over grid points. The grid dimensions are specified
* using the {@link #gridDimension(Object, VariableGridSpec)} or {@link #gridDimension(Object, double, double, int)}
* method. Gridding is performed by manipulating (and copying) an input {@link MutableState}. If the input state
* contains state variables that do not have a grid spec, then those values will hold constant.
* @author James MacGlashan.
*/
public class FlatStateGridder {
protected Map
© 2015 - 2024 Weber Informatics LLC | Privacy Policy