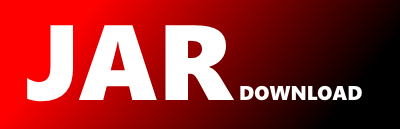
edu.byu.hbll.box.internal.util.UriBuilder Maven / Gradle / Ivy
package edu.byu.hbll.box.internal.util;
import java.net.URI;
import java.net.URLEncoder;
import java.nio.charset.StandardCharsets;
import java.util.ArrayList;
import java.util.Collection;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.Optional;
/**
* A uri builder.
*
* @author Charles Draper
*/
public class UriBuilder {
private String scheme = "http";
private String host;
private int port = -1;
private List paths = new ArrayList<>();
private Map> queryParams = new LinkedHashMap<>();
/**
* Creates a new {@link UriBuilder} based on the given uri.
*
* @param uri the default uri
*/
public UriBuilder(URI uri) {
scheme(uri.getScheme());
host(uri.getHost());
port(uri.getPort());
path(uri.getPath());
}
/**
* Creates a new {@link UriBuilder} based on the given uri.
*
* @param uri the default uri
*/
public UriBuilder(String uri) {
this(URI.create(uri));
}
/**
* Sets the scheme.
*
* @param scheme the scheme to set
* @return this
*/
public UriBuilder scheme(String scheme) {
this.scheme = scheme;
return this;
}
/**
* Sets the host.
*
* @param host the host to set
* @return this
*/
public UriBuilder host(String host) {
this.host = host;
return this;
}
/**
* Sets the port.
*
* @param port the port to set
* @return this
*/
public UriBuilder port(int port) {
this.port = port;
return this;
}
/**
* Adds to the path.
*
* @param path the path to add
* @return this
*/
public UriBuilder path(String path) {
paths.add(path.replaceAll("^/", ""));
return this;
}
/**
* Adds query parameters to the uri.
*
* @param name the name of the query parameter
* @param value the value(s) to add
* @return this
*/
public UriBuilder queryParam(String name, Object... value) {
for (Object v : value) {
if (v instanceof Optional) {
v = ((Optional>) v).orElse(null);
}
if (v == null) {
// do nothing
} else if (v instanceof Collection) {
((Collection>) v).forEach(p -> queryParam(name, p.toString()));
} else {
queryParam(name, v.toString());
}
}
return this;
}
private void queryParam(String name, String value) {
queryParams.computeIfAbsent(name, k -> new ArrayList<>()).add(value);
}
/**
* Builds the {@link URI}.
*
* @return the newly built uri
*/
public URI build() {
StringBuilder builder = new StringBuilder();
builder.append(scheme);
builder.append("://");
builder.append(host);
if (port != -1) {
builder.append(":");
builder.append(port);
}
boolean prevSlash = false;
for (String path : paths) {
if (!prevSlash) {
builder.append("/");
}
builder.append(path);
prevSlash = path.endsWith("/");
}
if (!queryParams.isEmpty()) {
builder.append("?");
boolean first = true;
for (String name : queryParams.keySet()) {
for (String value : queryParams.get(name)) {
if (!first) {
builder.append("&");
}
builder.append(name);
builder.append("=");
builder.append(URLEncoder.encode(value, StandardCharsets.UTF_8));
first = false;
}
}
}
return URI.create(builder.toString());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy