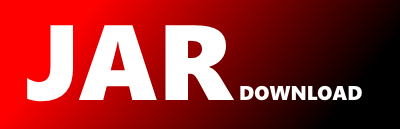
edu.byu.hbll.box.Facet Maven / Gradle / Ivy
The newest version!
package edu.byu.hbll.box;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.io.Serializable;
import java.util.Collection;
import java.util.LinkedHashMap;
import java.util.LinkedHashSet;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Set;
import java.util.stream.Collectors;
/**
* Represents a facet. Immutable.
*
* @author Charles Draper
*/
public final class Facet implements Serializable {
private static final long serialVersionUID = 1L;
/** The name of the facet. */
@JsonProperty private String name;
/** The facet vlaue. */
@JsonProperty private String value;
/** Constructor for serializers/deserializers. */
@SuppressWarnings("unused")
private Facet() {}
/**
* Creates a new {@link Facet} with the given name and value.
*
* @param name name of the facet group
* @param value value of the facet
*/
public Facet(String name, String value) {
this.name = validateName(name);
this.value = Objects.requireNonNull(value);
}
@Override
public int hashCode() {
return Objects.hash(name, value);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof Facet)) {
return false;
}
Facet other = (Facet) o;
return Objects.equals(name, other.name) && Objects.equals(value, other.value);
}
@Override
public String toString() {
return name + ":" + value;
}
/**
* Validates a facet group name. Facet names must contain only characters in [0-9A-Za-z_].
*
* @param name name of the facet group
* @return whether or not the name is valid
*/
public static String validateName(String name) {
if (name == null || !name.matches("[0-9A-Za-z_]+")) {
throw new IllegalArgumentException("facet keys must be alphanumeric");
}
return name;
}
/**
* Parses a facet value in the form of "NAME:VALUE".
*
* @param facet the facet value
* @return the parsed facet
*/
public static Facet parse(String facet) {
return new Facet(validateName(facet.replaceFirst(":.+", "")), facet.replaceFirst(".+?:", ""));
}
/**
* Parses facet values in the form of "NAME:VALUE".
*
* @param facets the facets to parse
* @return the parsed facets
*/
public static List parse(Collection facets) {
return facets.stream().map(f -> parse(f)).collect(Collectors.toList());
}
/**
* Utility method for grouping facets by name.
*
* @param facets a collection of facets
* @return a map of group name to a set of corresponding facets
*/
public static Map> group(Collection facets) {
Map> facetMap = new LinkedHashMap<>();
for (Facet facet : facets) {
facetMap.computeIfAbsent(facet.getName(), f -> new LinkedHashSet<>()).add(facet);
}
return facetMap;
}
/**
* Returns the name of the facet.
*
* @return the name of the facet
*/
public String getName() {
return name;
}
/**
* Returns the value of the facet.
*
* @return the value of the facet.
*/
public String getValue() {
return value;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy