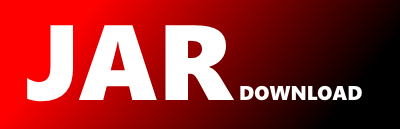
edu.byu.hbll.box.QueryResult Maven / Gradle / Ivy
The newest version!
package edu.byu.hbll.box;
import java.util.ArrayList;
import java.util.Collection;
/** A response from a querying box. */
public class QueryResult extends ArrayList {
private static final long serialVersionUID = 1L;
/** The next cursor. */
private long nextCursor;
/** Constructs a new blank {@link QueryResult}. */
public QueryResult() {}
/**
* Constructs a new {@link QueryResult} initialized with the given documents.
*
* @param documents the intial documents
*/
public QueryResult(Collection extends BoxDocument> documents) {
this.addAll(documents);
}
/**
* Returns the nextCursor.
*
* @return the nextCursor
*/
public long getNextCursor() {
return nextCursor;
}
/**
* Sets the next cursor.
*
* @param nextCursor the nextCursor to set
* @return this
*/
public QueryResult setNextCursor(long nextCursor) {
this.nextCursor = nextCursor;
return this;
}
/**
* Updates nextCursor according to the last document in the query result. If the result is empty,
* it reuses the cursor in the query.
*
* @param query the original query containing the cursor
* @return this
*/
public QueryResult updateNextCursor(BoxQuery query) {
if (!isEmpty()) {
nextCursor =
get(size() - 1).getCursor().orElse(BoxQuery.DEFAULT_CURSOR)
+ (query.isAscendingOrder() ? 1 : -1);
} else {
nextCursor = query.getCursorOrDefault();
}
return this;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy