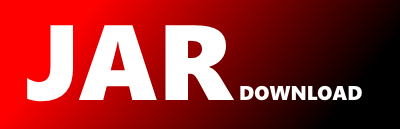
edu.byu.hbll.box.internal.util.BoxUtils Maven / Gradle / Ivy
The newest version!
package edu.byu.hbll.box.internal.util;
import edu.byu.hbll.box.BoxQuery;
import java.time.Duration;
import java.time.Instant;
import java.time.temporal.ChronoUnit;
import java.util.Collection;
import java.util.Collections;
import java.util.LinkedHashSet;
import java.util.Random;
import java.util.Set;
import java.util.stream.Collectors;
/**
* Random utilities used by Box.
*
* @author Charles Draper
*/
public class BoxUtils {
private static final Random r = new Random();
/**
* Calculates a retry date/time based on a base date/time (eg. first attempt), a retry delay, and
* retry jitter.
*
* The minimum (ie, the first) delay corresponds to the retryDelay
. Subsequent
* delays are calculated by taking the difference between NOW and the base
. This
* essentially doubles the delay each time. A random amount of jitter +/- is added to the
* calculated delay. The bounds of the jitter is based on the retryJitter
parameter
* which is a percentage of the calculated delay. This random variation can be critical if
* failures occur during certain windows of time or as part of batches with other conflicting
* items.
*
*
Examples:
*
*
* - Given a
base
of 1 second ago, retryDelay
of 1 minute,
* retryJitter
of 0.5, the retry date/time will be anywhere from 30 seconds to 90
* seconds from NOW (minimum of 1 minute +/- 1 minute * 0.5).
* - Given a
base
of 8 minutes ago, retryDelay
of 1 minute,
* retryJitter
of 0.5, the retry date/time will be anywhere from 4 minutes to 12
* minutes from NOW (8 minutes +/- 8 minutes * 0.5)..
*
*
* @param base the base date/time the retry date/time is calculated from
* @param retryDelay the initial or minimum delay before processing again
* @param retryJitter a percentage of the calculated delay added or subtracted
* @return the retry date/time
*/
public static Instant retryDateTime(Instant base, Duration retryDelay, double retryJitter) {
long delay = Math.max(retryDelay.toMillis(), ChronoUnit.MILLIS.between(base, Instant.now()));
double jitterCoefficient = r.nextDouble() * (retryJitter * 2) + (1 - retryJitter);
long delayWithJitter = (long) (delay * jitterCoefficient);
return Instant.now().plusMillis(delayWithJitter);
}
/**
* Converts non _at_doc fields into _at_doc fields.
*
* @param fields dot-notated fields denoting what should be projected
* @return the new fields
*/
public static Set canonicalizeFields(Collection fields) {
return Collections.unmodifiableSet(
(Set)
fields.stream()
.map(
field -> {
if (!field.equals(BoxQuery.DOCUMENT_FIELD)
&& !field.startsWith("@doc.")
&& !field.equals(BoxQuery.METADATA_FIELD)
&& !field.startsWith("@box.")) {
return "@doc." + field;
} else {
return field;
}
})
.collect(Collectors.toCollection(LinkedHashSet::new)));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy