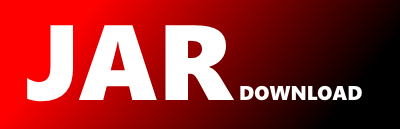
edu.byu.hbll.box.HarvestResult Maven / Gradle / Ivy
package edu.byu.hbll.box;
import com.fasterxml.jackson.databind.node.JsonNodeFactory;
import com.fasterxml.jackson.databind.node.ObjectNode;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
/**
* Contains a batch of resulting documents and future harvest information for a given harvest.
*
* @author Charles Draper
*/
public class HarvestResult extends ArrayList {
private static final long serialVersionUID = 1L;
/** An object describing where the harvest left off. */
private ObjectNode cursor;
/**
* Whether or not there are more documents to harvest at this time. If null, use
* !documents.isEmpty() instead.
*/
private boolean more;
/** Creates an empty {@link HarvestResult}. */
public HarvestResult() {}
/**
* Creates a completely initialized {@link HarvestResult}.
*
* @param documents the resulting documents to be added
* @param cursor an object describing where the harvest left off
* @param more whether or not there are more documents to harvest at this time
*/
public HarvestResult(
Collection extends BoxDocument> documents, ObjectNode cursor, boolean more) {
addAll(documents);
this.cursor = cursor;
this.more = more;
}
/**
* Sets more. Default false.
*
* @param more whether or not there are more documents to harvest at this time.
* @return this {@link HarvestResult}
*/
public HarvestResult setMore(boolean more) {
this.more = more;
return this;
}
/**
* Creates a new {@link HarvestResult} with the given cursor.
*
* @param cursor an object describing where the harvest left off.
* @return this {@link HarvestResult}
*/
public HarvestResult setCursor(ObjectNode cursor) {
this.cursor = cursor;
return this;
}
/**
* Same as add() except returns this.
*
* @param document resulting document to add.
* @return this {@link HarvestResult}
*/
public HarvestResult addDocument(BoxDocument document) {
add(document);
return this;
}
/**
* Same as addAll() except returns this.
*
* @param documents resulting documents to add.
* @return this {@link HarvestResult}
*/
public HarvestResult addDocuments(BoxDocument... documents) {
return addDocuments(Arrays.asList(documents));
}
/**
* Same as addAll() except returns this.
*
* @param documents resulting documents to add.
* @return this {@link HarvestResult}
*/
public HarvestResult addDocuments(Collection extends BoxDocument> documents) {
documents.forEach(d -> add(d));
return this;
}
/**
* Returns the cursor to store.
*
* @return the cursor
*/
public ObjectNode getCursor() {
return cursor;
}
/**
* Returns whether or not there are more documents to harvest.
*
* @return the more
*/
public boolean hasMore() {
return more;
}
/**
* Returns the cursor. If cursor is null, it is first set to an empty {@link ObjectNode}.
*
* @return the cursor
*/
public ObjectNode withCursor() {
if (cursor == null) {
this.cursor = JsonNodeFactory.instance.objectNode();
}
return this.cursor;
}
/**
* Marks the start of a new group to be processed identified by the given groupId. This is
* necessary for identifying orphaned documents and deleting them when they're no longer part of
* the group.
*
* @param groupId the group's id
* @return this
*/
public HarvestResult startGroup(String groupId) {
add(new StartGroupDocument(groupId));
return this;
}
/**
* Marks the moment a group finished processing. This is necessary for identifying orphaned
* documents and deleting them when they're no longer part of the group.
*
* @param groupId the group's id
* @return this
*/
public HarvestResult endGroup(String groupId) {
add(new EndGroupDocument(groupId));
return this;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy