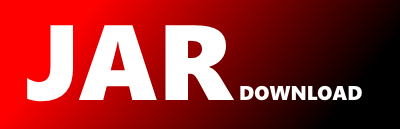
edu.byu.hbll.box.ProcessBatch Maven / Gradle / Ivy
package edu.byu.hbll.box;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import java.util.stream.Collectors;
/**
* A batch of ids/contexts to be processed by a {@link Processor}.
*
* @author Charles Draper
*/
public class ProcessBatch extends ArrayList {
private static final long serialVersionUID = 1L;
/** Creates a new empty {@link ProcessBatch}. */
public ProcessBatch() {}
/**
* Creates a new {@link ProcessBatch} initialized with the given contexts.
*
* @param contexts the contexts associated with this batch
*/
public ProcessBatch(Collection extends ProcessContext> contexts) {
this.addAll(contexts);
}
/**
* Returns the ids.
*
* @return the ids from the batch of contexts
*/
public List getIds() {
return stream().map(c -> c.getId()).collect(Collectors.toList());
}
/**
* Returns the first id or null if batch is empty.
*
* @return the first id from the batch, null if the batch is empty
*/
public String getFirstId() {
return stream().findFirst().map(c -> c.getId()).orElse(null);
}
/**
* Returns the first found context or null if empty.
*
* @return the first context from the batch, null if the batch is empty
*/
public ProcessContext getFirstContext() {
return stream().findFirst().orElse(null);
}
/**
* Returns the context for the given id or null if not found.
*
* @param id the document id
* @return the context associated with the given id, null if id not found
*/
public ProcessContext get(String id) {
return stream().filter(c -> c.getId().equals(id)).findFirst().orElse(null);
}
/**
* Creates a new {@link ProcessBatch} from a list of ids only (ie, no dependency information).
*
* @param ids the ids for the batch.
* @return a new batch
*/
public static ProcessBatch ofIds(List ids) {
ProcessBatch batch = new ProcessBatch();
ids.forEach(id -> batch.add(new ProcessContext(id)));
return batch;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy