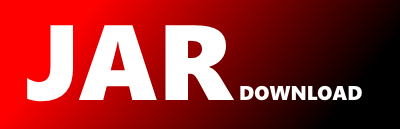
edu.byu.hbll.box.ProcessContext Maven / Gradle / Ivy
package edu.byu.hbll.box;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import lombok.Getter;
/**
* Contains information about each document to be processed including the ID and required
* dependencies.
*
* @author Charles Draper
*/
public class ProcessContext {
/** The singleton Box object. */
@Getter private Box box;
/** The source for this harvest. */
@Getter private Source source;
/** The document Id to process. */
private String id;
/** The dependencies of the document to be processed. */
private Map dependencies = new HashMap<>();
private Map> dependenciesBySource = new HashMap<>();
/**
* Creates a new simple {@link ProcessContext} that only specifies which ID to process.
*
* @param id the document ID to process.
*/
public ProcessContext(String id) {
this.id = id;
}
/**
* Creates a new {@link ProcessContext} with the given ID and dependencies.
*
* @param id the document ID to process.
* @param dependencies the dependencies of the document to be processed.
*/
public ProcessContext(String id, Map dependencies) {
this.id = id;
this.dependencies = dependencies;
for (DocumentId documentId : dependencies.keySet()) {
dependenciesBySource
.computeIfAbsent(documentId.getSourceName(), k -> new ArrayList<>())
.add(dependencies.get(documentId));
}
}
/**
* Creates a new {@link ProcessContext} with the given ID and dependencies.
*
* @param box the singleton Box object
* @param source the source for this harvest
* @param id the document ID to process.
* @param dependencies the dependencies of the document to be processed.
*/
public ProcessContext(
Box box, Source source, String id, Map dependencies) {
this.box = box;
this.source = source;
this.id = id;
this.dependencies = dependencies;
for (DocumentId documentId : dependencies.keySet()) {
dependenciesBySource
.computeIfAbsent(documentId.getSourceName(), k -> new ArrayList<>())
.add(dependencies.get(documentId));
}
}
/**
* Returns the id to be processed.
*
* @return the id to be processed
*/
public String getId() {
return id;
}
/**
* Returns the dependency matching the given document id or null if none.
*
* @param sourceName sourceName of the dependency
* @param id id of the dependency
* @return the dependency identified by the given sourceName and id or null if dependency is not
* processed (not found)
*/
public BoxDocument getDependency(String sourceName, String id) {
return getDependency(new DocumentId(sourceName, id));
}
/**
* Returns the dependency matching the given document id or null if none.
*
* @param documentId documentId of the dependency
* @return the dependency identified by the given documentId or null if dependency is not
* processed (not found)
*/
public BoxDocument getDependency(DocumentId documentId) {
return dependencies.get(documentId);
}
/**
* Returns the dependencies.
*
* @return the dependencies
*/
public Map getDependencies() {
return dependencies;
}
/**
* Gets a list of all dependencies for the given source. If there are no dependencies for the
* source, an empty list is returned.
*
* @param sourceName source name of the dependencies
* @return list of dependency documents
*/
public List getDependencies(String sourceName) {
return dependenciesBySource.getOrDefault(sourceName, Collections.emptyList());
}
/**
* Gets the first dependency for the given source. If there are no dependencies for the source,
* null is returned.
*
* @param sourceName source name of the dependency
* @return first dependency if one exists, null otherwise
*/
public BoxDocument getFirstDependency(String sourceName) {
List dependencies = getDependencies(sourceName);
if (dependencies.isEmpty()) {
return null;
} else {
return dependencies.get(0);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy