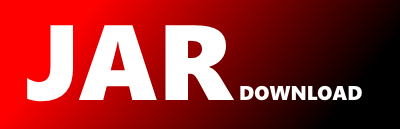
edu.byu.hbll.box.ConstructConfig Maven / Gradle / Ivy
package edu.byu.hbll.box;
import com.fasterxml.jackson.databind.node.JsonNodeFactory;
import com.fasterxml.jackson.databind.node.ObjectNode;
import edu.byu.hbll.box.internal.util.JsonUtils;
import java.util.Objects;
/**
* Configuration for initializing newly instantiated Box extension classes.
*
* @author Charles Draper
*/
public class ConstructConfig {
private String sourceName;
private ObjectNode params = JsonNodeFactory.instance.objectNode();
private ObjectType objectType;
/** Creates a new empty {@link ConstructConfig}. */
public ConstructConfig() {}
/**
* Constructs a new {@link ConstructConfig} with the given source name and object params.
*
* @param sourceName the source name
* @param params the instance specific parameters (found in the params section of the
* configuration)
*/
public ConstructConfig(String sourceName, ObjectNode params) {
this.sourceName = Objects.requireNonNull(sourceName);
this.params = Objects.requireNonNull(params);
}
/**
* Binds the params to the target object using Jackson's binding mechanism and rules.
*
* @param target the target object
*/
public void bind(Object target) {
JsonUtils.bind(params, target);
}
/**
* Returns the source name.
*
* @return the source name
*/
public String getSourceName() {
return sourceName;
}
/**
* Sets the source name.
*
* @param sourceName the source name to set
*/
public void setSourceName(String sourceName) {
this.sourceName = sourceName;
}
/**
* Returns the params.
*
* @return the the instance specific parameters (found in the params section of the configuration)
*/
public ObjectNode getParams() {
return params;
}
/**
* Sets the params.
*
* @param params the the instance specific parameters to set (found in the params section of the
* configuration)
*/
public void setParams(ObjectNode params) {
this.params = Objects.requireNonNull(params);
}
/**
* Returns the object type.
*
* @return the objectType
*/
public ObjectType getObjectType() {
return objectType;
}
/**
* Sets the object type.
*
* @param objectType the objectType to set
*/
public void setObjectType(ObjectType objectType) {
this.objectType = objectType;
}
/**
* Returns whether or not this is of type processor.
*
* @return whether or not this is of type processor
*/
public boolean isProcessor() {
return objectType == ObjectType.PROCESSOR;
}
/**
* Returns whether or not this is of type harvester.
*
* @return whether or not this is of type harvester
*/
public boolean isHarvester() {
return objectType == ObjectType.HARVESTER;
}
/**
* Returns whether or not this is of type database.
*
* @return whether or not this is of type database
*/
public boolean isBoxDatabase() {
return objectType == ObjectType.BOX_DATABASE;
}
/**
* Returns whether or not this is of type cursor database.
*
* @return whether or not this is of type cursor database
*/
public boolean isCursorDatabase() {
return objectType == ObjectType.CURSOR_DATABASE;
}
/**
* Returns if type is other.
*
* @return if type is other
*/
public boolean isOther() {
return objectType == ObjectType.OTHER;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy