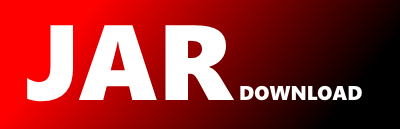
edu.byu.hbll.box.DatabaseCopier Maven / Gradle / Ivy
package edu.byu.hbll.box;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.JsonNode;
import edu.byu.hbll.box.internal.core.DefaultObjectFactory;
import edu.byu.hbll.json.YamlLoader;
import java.io.IOException;
import java.nio.file.Paths;
/**
* Copies one box database to another. This includes a main method which requires a single yaml file
* with the following format.
*
*
* sources:
* - from: src1
* - from: src2a
* to: src2b
* db:
* from:
* type: # source database type
* params: # source database parameters
* to:
* type: # destination database type
* params: # destination database parameters
*
*/
public class DatabaseCopier {
/**
* Run the copier from the command line.
*
* @param args command-line arguments
* @throws JsonProcessingException if an error occurs parsing the yaml file
* @throws IOException if an error occurs parsing the yaml file
*/
public static void main(String[] args) throws JsonProcessingException, IOException {
if (args.length != 1) {
System.err.println("the copier requires exactly one argument, path to the yaml");
return;
}
JsonNode config = new YamlLoader().load(Paths.get(args[0]));
for (JsonNode source : config.path("sources")) {
String from;
String to;
if (source.isObject()) {
from = source.path("from").asText();
to = source.path("to").asText(from);
} else {
from = source.asText();
to = from;
}
ObjectFactory objectFactory = new DefaultObjectFactory();
JsonNode databaseConfig = config.path("db");
BoxDatabase fromdb = BoxDatabase.create(from, objectFactory, databaseConfig.path("from"));
BoxDatabase todb = BoxDatabase.create(to, objectFactory, databaseConfig.path("to"));
copy(fromdb, todb);
}
}
/**
* Copies one database to another. Note that this only copies documents. The process queue and
* groups are not copied.
*
* @param from the source database
* @param to the destinations database
*/
public static void copy(BoxDatabase from, BoxDatabase to) {
long cursor = 0;
while (true) {
QueryResult result = from.find(new BoxQuery().setCursor(cursor).addAllStatuses());
if (result.isEmpty()) {
break;
}
to.save(result);
cursor = result.getNextCursor();
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy