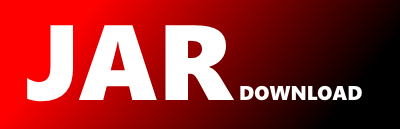
edu.byu.hbll.box.ProcessResult Maven / Gradle / Ivy
package edu.byu.hbll.box;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
/**
* Contains the result of processing a batch of documents.
*
* @author Charles Draper
*/
public class ProcessResult extends ArrayList {
private static final long serialVersionUID = 1L;
/** Creates an empty {@link ProcessResult}. */
public ProcessResult() {}
/**
* Creates a new {@link ProcessResult} by adding the initial documents.
*
* @param documents the resulting documents to be saved
*/
public ProcessResult(BoxDocument... documents) {
addAll(Arrays.asList(documents));
}
/**
* Creates a new {@link ProcessResult} by adding the initial documents.
*
* @param documents the resulting documents to be saved
*/
public ProcessResult(Collection extends BoxDocument> documents) {
addAll(documents);
}
/**
* Same as add() except returns this.
*
* @param document the resulting document to be saved
* @return this
*/
public ProcessResult addDocument(BoxDocument document) {
add(document);
return this;
}
/**
* Same as addAll() except returns this.
*
* @param documents the resulting documents to be saved
* @return this
*/
public ProcessResult addDocuments(BoxDocument... documents) {
return addDocuments(Arrays.asList(documents));
}
/**
* Same as addAll() except returns this.
*
* @param documents the resulting documents to be saved
* @return this
*/
public ProcessResult addDocuments(Collection extends BoxDocument> documents) {
documents.forEach(d -> addDocument(d));
return this;
}
/**
* Marks the start of a new group to be processed identified by the given groupId. This is
* necessary for identifying orphaned documents and deleting them when they're no longer part of
* the group.
*
* @param groupId the group's id
* @return this
*/
public ProcessResult startGroup(String groupId) {
add(new StartGroupDocument(groupId));
return this;
}
/**
* Marks the moment a group finished processing. This is necessary for identifying orphaned
* documents and deleting them when they're no longer part of the group.
*
* @param groupId the group's id
* @return this
*/
public ProcessResult endGroup(String groupId) {
add(new EndGroupDocument(groupId));
return this;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy