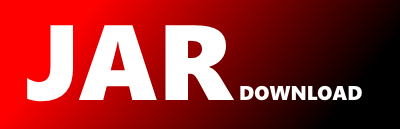
edu.byu.hbll.box.Source Maven / Gradle / Ivy
package edu.byu.hbll.box;
import edu.byu.hbll.box.internal.core.DocumentIterable;
import edu.byu.hbll.box.internal.core.Registry;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.List;
import java.util.stream.Stream;
import java.util.stream.StreamSupport;
/**
* Main interface into a source.
*
* @author Charles Draper
*/
public class Source {
private final String name;
private final SourceConfig config;
private final Registry registry;
private final boolean principal;
/**
* Constructs a new {@link Source} given the config and registry.
*
* @param config the source config
* @param registry the registry
*/
Source(SourceConfig config, Registry registry) {
this.name = config.getName();
this.config = config;
this.registry = registry;
this.principal = registry.getPrincipalSource().getName().equals(config.getName());
}
/**
* Returns whether or not this source is the principal source.
*
* @return whether or not this source is the principal source
*/
public boolean isPrincipal() {
return principal;
}
/** Clears the database. All documents, metadata, cursor, etc are removed. */
public void clear() {
registry.getSource(name).getDb().clear();
}
/**
* Returns whether or not this node is the primary node in the cluster for this source.
*
* @return whether or not this node is the primary for this source
*/
public boolean isPrimary() {
return registry.isPrimary(name);
}
/**
* Collects and returns all documents found according to the given query.
*
* @param query the query
* @return found documents
*/
public QueryResult collect(BoxQuery query) {
QueryResult result = new QueryResult();
find(query).forEach(d -> result.add(d));
result.updateNextCursor(query);
return result;
}
/**
* Returns documents found according to the given query in the form of an {@link Iterable}.
*
* @param query the query
* @return found documents
*/
public Iterable find(BoxQuery query) {
return new DocumentIterable(
query, q -> registry.getDocumentHandler().find(registry.verifySource(name), q));
}
/**
* Returns the underlying configuration.
*
* @return the underlying configuration
*/
public SourceConfig getConfig() {
return config;
}
/**
* Returns a snapshot of the health of the Source.
*
* @return the health of Box
*/
public SourceHealth getHealth() {
return registry.getBoxHealthCheck().getHealth().getSourceHealth(name);
}
/**
* Returns the name of this source.
*
* @return the name of this source
*/
public String getName() {
return name;
}
/**
* Queues up the given id to be run against the processor at the given time. Depending on how many
* ids are in the queue ready to be processed, processing of this could take place considerably
* after the given time. If the id is already in the queue, this will overwrite the attempt time.
*
* @param id the id to add to the queue
* @param attempt attempt to process at this time
*/
public void addToQueue(String id, Instant attempt) {
addToQueue(Arrays.asList(id), attempt);
}
/**
* Queues up the given ids to be run against the processor.
*
* @param ids the ids to add to the queue
*/
public void addToQueue(String... ids) {
addToQueue(Arrays.asList(ids), Instant.now());
}
/**
* Queues up the given ids to be run against the processor.
*
* @param ids the ids to add to the queue
*/
public void addToQueue(Collection ids) {
addToQueue(ids, Instant.now());
}
/**
* Queues up the given ids to be run against the processor at the given time. Depending on how
* many ids are in the queue ready to be processed, processing of these could take place
* considerably after the given time. If the id is already in the queue, this will overwrite the
* attempt time.
*
* @param ids the ids to add to the queue
* @param attempt attempt to process at this time
*/
public void addToQueue(Collection ids, Instant attempt) {
registry.getDocumentHandler().addToQueue(registry.verifySource(name), ids, attempt, true);
}
/**
* Adds the given entries to this source's process queue.
*
* @param entries entries to add to the queue
*/
public void addToQueue(QueueEntry... entries) {
config.getDb().addToQueue(List.of(entries));
}
/**
* Deletes the given ids from the process queue if they exist.
*
* @param ids the ids to delete from queue
*/
public void deleteFromQueue(String... ids) {
deleteFromQueue(List.of(ids));
}
/**
* Deletes the given ids from the process queue if they exist.
*
* @param ids the ids to delete from queue
* @throws IllegalStateException if not databases is configured
*/
public void deleteFromQueue(Collection ids) {
if (config.getDb() == null) {
throw new IllegalStateException("database is null");
}
config.getDb().deleteFromQueue(ids);
}
/**
* Registers a listener with the updates notification system. The update notification system
* executes all registered listeners at most once per second whenever there is an update within a
* source.
*
* @param listener the runnable to execute when there's an update detected
*/
public void registerForUpdateNotifications(Runnable listener) {
registry.getUpdatesNotifier().register(registry.verifySource(name), listener);
}
/**
* Saves the given documents to the database. The modified and cursor fields are only updated for
* new or updated documents.
*
* @param documents the documents to save
*/
public void save(BoxDocument... documents) {
save(List.of(documents));
}
/**
* Saves the given documents to the database. The modified and cursor fields are only updated for
* new or updated documents.
*
* @param documents the documents to save
*/
public void save(Collection extends BoxDocument> documents) {
registry.getDocumentHandler().save(registry.verifySource(name), documents);
}
/**
* Returns documents found according to the given query in the form of a {@link Stream}.
*
* @param query the query
* @return found documents
*/
public Stream stream(BoxQuery query) {
return StreamSupport.stream(find(query).spliterator(), false);
}
/** Triggers the harvester to run. */
public void triggerHarvest() {
registry.triggerHarvest(registry.verifySource(name));
}
/**
* Returns a count of the number of documents that match the query. For id type queries, the count
* is the same as the number of requested ids. For harvest type queries, the limit
* parameter is ignored, but all other parameters are honored.
*
* @param query the query
* @return number of matching documents
*/
public long count(BoxQuery query) {
return config.getDb().count(query);
}
/**
* Processes the given id and returns the document.
*
* @param id the id to process
* @return the resulting document
*/
public BoxDocument process(String id) {
return process(List.of(id)).get(0);
}
/**
* Processes the given ids and returns the documents.
*
* @param ids the ids to process
* @return the resulting documents
*/
public List process(String... ids) {
return collect(new BoxQuery(ids).setProcess(true));
}
/**
* Processes the given ids and returns the documents.
*
* @param ids the ids to process
* @return the resulting documents
*/
public List process(Collection ids) {
return collect(new BoxQuery(ids).setProcess(true));
}
/**
* Retrieves the document for the given id.
*
* @param id the id of the document to retrieve
* @return the document
*/
public BoxDocument get(String id) {
return get(List.of(id)).get(0);
}
/**
* Retrieves the documents for the given ids.
*
* @param ids the ids of the documents to retrieve
* @return the documents
*/
public List get(String... ids) {
return get(List.of(ids));
}
/**
* Retrieves the documents for the given ids.
*
* @param ids the ids of the documents to retrieve
* @return the resulting documents
*/
public List get(Collection ids) {
return collect(new BoxQuery(ids));
}
@Override
public String toString() {
return name;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy