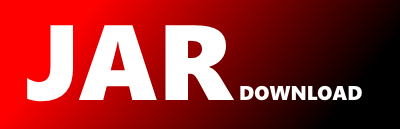
csip.PayloadAttachments Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of csip-core Show documentation
Show all versions of csip-core Show documentation
The Cloud Services Integration Platform is a SoA implementation to offer a Model-as-a-Service framework, Application Programming Interface, deployment infrastructure, and service implementations for environmental modeling.
/*
* $Id: PayloadAttachments.java 56b3e81122ec 2019-08-26 od $
*
* This file is part of the Cloud Services Integration Platform (CSIP),
* a Model-as-a-Service framework, API and application suite.
*
* 2012-2019, Olaf David and others, OMSLab, Colorado State University.
*
* OMSLab licenses this file to you under the MIT license.
* See the LICENSE file in the project root for more information.
*/
package csip;
import java.io.File;
import java.util.Arrays;
import java.util.Collection;
import java.util.Set;
import java.util.TreeSet;
/**
* FormDataInput
*
* @author od
*/
public class PayloadAttachments {
private final String[] inputs;
private final ModelDataService mds;
PayloadAttachments(String[] inputs, ModelDataService mds) {
this.inputs = inputs;
this.mds = mds;
}
/**
* Get the attached files for this request. This includes the request.
* @return The set of files.
*/
public Collection getFiles() {
Set f = new TreeSet<>();
for (String att : inputs) {
File file = new File(mds.getWorkspaceDir(), att);
if (file.exists()) {
f.add(file);
}
}
return f;
}
/**
* Get the number of attachments. This includes the request.
* @return the number of files attached.
*/
public int getFilesCount() {
return inputs.length;
}
/**
* Check is a input file exists in the workspace.
*
* @param name the file name
* @return true if the file exist, false otherwise
* @throws ServiceException if there is a JSON error.
*/
public boolean hasFile(String name) throws ServiceException {
return getFile(name) != null;
}
/**
* Check if a required file attachment exists in the workspace.
*
* @param name the file name
* @return this object
* @throws ServiceException if the parameter is not found.
*/
public PayloadAttachments require(String name) throws ServiceException {
if (!hasFile(name)) {
throw new ServiceException("File attachment not found :" + name);
}
return this;
}
/**
* Get a file object for a given file name.
* @param name the file name (no path)
* @return the file object with its absolute file path within the workspace.
* It returns null if the file is not input or does not exist.
* @throws csip.ServiceException if the file is missing
*/
public File getFile(String name) throws ServiceException {
if (!Arrays.asList(inputs).contains(name)) {
return null;
}
File f = mds.getWorkspaceFile(name);
if (f.exists()) {
return f;
}
throw new ServiceException("Missing Input File: " + name);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy