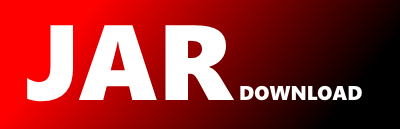
csip.PayloadParameter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of csip-core Show documentation
Show all versions of csip-core Show documentation
The Cloud Services Integration Platform is a SoA implementation to offer a Model-as-a-Service framework, Application Programming Interface, deployment infrastructure, and service implementations for environmental modeling.
/*
* $Id: PayloadParameter.java 9e04709703fa 2020-04-27 od $
*
* This file is part of the Cloud Services Integration Platform (CSIP),
* a Model-as-a-Service framework, API and application suite.
*
* 2012-2019, Olaf David and others, OMSLab, Colorado State University.
*
* OMSLab licenses this file to you under the MIT license.
* See the LICENSE file in the project root for more information.
*/
package csip;
import static csip.ModelDataService.GEOMETRY;
import static csip.ModelDataService.KEY_DESC;
import static csip.ModelDataService.UNIT;
import static csip.ModelDataService.VALUE;
import csip.utils.JSONUtils;
import java.util.Collection;
import java.util.Map;
import org.codehaus.jettison.json.JSONArray;
import org.codehaus.jettison.json.JSONException;
import org.codehaus.jettison.json.JSONObject;
/**
* Payload Parameter access.
*
* @author od
*/
public class PayloadParameter {
private final Map paramMap;
PayloadParameter(Map paramMap) {
this.paramMap = paramMap;
}
private Map getParamMap() {
return paramMap;
}
/**
* Check if all parameter exist.
* @param name the name of the parameter entry
* @return true if present false otherwise.
*/
public boolean has(String name) {
return getParamMap().containsKey(name);
}
/**
* Check if a required parameter exists.
*
* @param names the parameter names to check
* @return this object
* @throws ServiceException if one or more parameter are not found.
*/
public PayloadParameter require(String... names) throws ServiceException {
for (String name : names) {
if (!has(name)) {
throw new ServiceException("Required parameter not found: " + name);
}
}
return this;
}
/**
* Get the number of parameter.
* @return the number of parameter
*/
public int getCount() {
return getParamMap().size();
}
/**
* Get all parameter names.
* @return the set of names.
*/
public Collection getNames() {
return getParamMap().keySet();
}
/**
* Get the entire parameter as JSONObject.
* @param name the parameter name.
* @return the parameter JSONObject.
* @throws ServiceException if there is no such parameter.
*/
public JSONObject getParamJSON(String name) throws ServiceException {
return get(name);
}
/**
* Get a String parameter.
* @param name the parameter name
* @return the parameter value as String
* @throws ServiceException if there is a JSON error.
*/
public String getString(String name) throws ServiceException {
try {
return get(name).getString(VALUE);
} catch (JSONException ex) {
throw new ServiceException("No Value for " + name, ex);
}
}
/**
* Get a String parameter.
* @param name the name of the parameter
* @param def the default value if the parameter is missing
* @return the value of the parameter
* @throws ServiceException if there is a JSON error.
*/
public String getString(String name, String def) throws ServiceException {
try {
JSONObject p = getParamMap().get(name);
return (p == null) ? def : p.getString(VALUE);
} catch (JSONException ex) {
throw new ServiceException("No Value for " + name, ex);
}
}
/**
* Get an int parameter.
* @param name the parameter name
* @return the parameter value as int
* @throws ServiceException if there is a JSON error.
*/
public int getInt(String name) throws ServiceException {
try {
return get(name).getInt(VALUE);
} catch (JSONException ex) {
throw new ServiceException("No Value for " + name, ex);
}
}
/**
* Get a int parameter.
* @param name the name of the parameter
* @param def the default value if parameter does not exist
* @return the int value of the parameter.
* @throws ServiceException if there is a JSON error.
*/
public int getInt(String name, int def) throws ServiceException {
try {
JSONObject p = getParamMap().get(name);
return (p == null) ? def : p.getInt(VALUE);
} catch (JSONException ex) {
throw new ServiceException("No Value for " + name, ex);
}
}
/**
* Get an double parameter.
* @param name the parameter name
* @return the parameter value as double
* @throws ServiceException if there is a JSON error.
*/
public double getDouble(String name) throws ServiceException {
try {
return get(name).getDouble(VALUE);
} catch (JSONException ex) {
throw new ServiceException("No Value for " + name, ex);
}
}
public double getDouble(String name, double min, double max) throws ServiceException {
double val = getDouble(name);
checkRange(name, min, max, val);
return val;
}
/**
* Get a double parameter.
* @param name the name of the parameter
* @param def the default value if parameter does not exist
* @return the double value of the parameter
* @throws ServiceException if there is a JSON error.
*/
public double getDouble(String name, double def) throws ServiceException {
try {
JSONObject p = getParamMap().get(name);
return (p == null) ? def : p.getDouble(VALUE);
} catch (JSONException ex) {
throw new ServiceException("No Value for " + name, ex);
}
}
public double getDouble(String name, double def, double min, double max) throws ServiceException {
double val = getDouble(name, def);
checkRange(name, min, max, val);
return val;
}
private static void checkRange(String name, double min, double max, double val) throws ServiceException {
if (val < min || val > max) {
throw new ServiceException(name + " is: " + val + ", must be in range [" + min + ", " + max + "]");
}
}
/**
* Get a boolean parameter.
* @param name the parameter name.
* @return the parameter value as boolean.
* @throws ServiceException if there is a JSON error.
*/
public boolean getBoolean(String name) throws ServiceException {
try {
return get(name).getBoolean(VALUE);
} catch (JSONException ex) {
throw new ServiceException("No Value for " + name, ex);
}
}
/**
* Get a Boolean parameter.
* @param name the name of the parameter
* @param def the default value.
* @return the boolean value of the parameter.
* @throws ServiceException if there is a JSON error.
*/
public boolean getBoolean(String name, boolean def) throws ServiceException {
try {
JSONObject p = getParamMap().get(name);
return (p == null) ? def : p.getBoolean(VALUE);
} catch (JSONException ex) {
throw new ServiceException("No Value for " + name, ex);
}
}
/**
* Get a long parameter.
* @param name the parameter name.
* @return the parameter value as long.
* @throws ServiceException if there is a JSON error.
*/
public long getLong(String name) throws ServiceException {
try {
return get(name).getLong(VALUE);
} catch (JSONException ex) {
throw new ServiceException("No Value for " + name, ex);
}
}
/**
* Get a Long parameter.
* @param name the name of the parameter
* @param def the default value if parameter does not exist
* @return the long value of the parameter
* @throws ServiceException if there is a JSON error.
*/
public long getLong(String name, long def) throws ServiceException {
try {
JSONObject p = getParamMap().get(name);
return (p == null) ? def : p.getLong(VALUE);
} catch (JSONException ex) {
throw new ServiceException("No Value for " + name, ex);
}
}
/**
* Get a JSONObject parameter value.
* @param name the parameter name.
* @return the parameter value as JSONObject.
* @throws ServiceException if there is a JSON error.
*/
public JSONObject getJSON(String name) throws ServiceException {
try {
return get(name).getJSONObject(VALUE);
} catch (JSONException ex) {
throw new ServiceException("No Value for " + name, ex);
}
}
/**
* Get a Long parameter.
* @param name the name of the parameter
* @param def the default value if parameter does not exist
* @return the JSONObject of the parameter
* @throws ServiceException if there is a JSON error.
*/
public JSONObject getJSON(String name, JSONObject def) throws ServiceException {
try {
JSONObject p = getParamMap().get(name);
return (p == null) ? def : p.getJSONObject(VALUE);
} catch (JSONException ex) {
throw new ServiceException("No Value for " + name, ex);
}
}
/**
* Get a JSONArray parameter.
* @param name the parameter name.
* @return the parameter value as JSONObject.
* @throws ServiceException if there is a JSON error.
*/
public JSONArray getJSONArray(String name) throws ServiceException {
try {
return get(name).getJSONArray(VALUE);
} catch (JSONException ex) {
throw new ServiceException("No Value for " + name, ex);
}
}
/**
* Get a Long parameter.
* @param name the name of the parameter
* @param def the default value if parameter does not exist
* @return the JSONObject of the parameter
* @throws ServiceException if there is a JSON error.
*/
public JSONArray getJSONArray(String name, JSONArray def) throws ServiceException {
try {
JSONObject p = getParamMap().get(name);
return (p == null) ? def : p.getJSONArray(VALUE);
} catch (JSONException ex) {
throw new ServiceException("No Value for " + name, ex);
}
}
/**
* Get a int[] parameter.
* @param name the parameter name.
* @return the parameter value as JSONObject.
* @throws ServiceException if there is a JSON error.
*/
public int[] getIntArray(String name) throws ServiceException {
try {
JSONArray a = get(name).getJSONArray(VALUE);
return JSONUtils.toIntArray(a);
} catch (JSONException ex) {
throw new ServiceException("No Value for " + name, ex);
}
}
/**
* Get a int[] parameter.
* @param name the name of the parameter
* @param def the default value if parameter does not exist
* @return the JSONObject of the parameter
* @throws ServiceException if there is a JSON error.
*/
public int[] getIntArray(String name, int[] def) throws ServiceException {
try {
JSONObject p = getParamMap().get(name);
return (p == null) ? def : JSONUtils.toIntArray(p.getJSONArray(VALUE));
} catch (JSONException ex) {
throw new ServiceException("No Value for " + name, ex);
}
}
/**
* Get a boolean[] parameter.
* @param name the parameter name.
* @return the parameter value as JSONObject.
* @throws ServiceException if there is a JSON error.
*/
public boolean[] getBooleanArray(String name) throws ServiceException {
try {
JSONArray a = get(name).getJSONArray(VALUE);
return JSONUtils.toBooleanArray(a);
} catch (JSONException ex) {
throw new ServiceException("No Value for " + name, ex);
}
}
/**
* Get a boolean[] parameter.
* @param name the name of the parameter
* @param def the default value if parameter does not exist
* @return the JSONObject of the parameter
* @throws ServiceException if there is a JSON error.
*/
public boolean[] getBooleanArray(String name, boolean[] def) throws ServiceException {
try {
JSONObject p = getParamMap().get(name);
return (p == null) ? def : JSONUtils.toBooleanArray(p.getJSONArray(VALUE));
} catch (JSONException ex) {
throw new ServiceException("No Value for " + name, ex);
}
}
/**
* Get a long[] parameter.
* @param name the parameter name.
* @return the parameter value as JSONObject.
* @throws ServiceException if there is a JSON error.
*/
public long[] getLongArray(String name) throws ServiceException {
try {
JSONArray a = get(name).getJSONArray(VALUE);
return JSONUtils.toLongArray(a);
} catch (JSONException ex) {
throw new ServiceException("No Value for " + name, ex);
}
}
/**
* Get a long[] parameter.
* @param name the name of the parameter
* @param def the default value if parameter does not exist
* @return the JSONObject of the parameter
* @throws ServiceException if there is a JSON error.
*/
public long[] getLongArray(String name, long[] def) throws ServiceException {
try {
JSONObject p = getParamMap().get(name);
return (p == null) ? def : JSONUtils.toLongArray(p.getJSONArray(VALUE));
} catch (JSONException ex) {
throw new ServiceException("No Value for " + name, ex);
}
}
/**
* Get a String[] parameter.
* @param name the parameter name.
* @return the parameter value as JSONObject.
* @throws ServiceException if there is a JSON error.
*/
public String[] getStringArray(String name) throws ServiceException {
try {
JSONArray a = get(name).getJSONArray(VALUE);
return JSONUtils.toStringArray(a);
} catch (JSONException ex) {
throw new ServiceException("No Value for " + name, ex);
}
}
/**
* Get a String[] parameter.
* @param name the name of the parameter
* @param def the default value if parameter does not exist
* @return the JSONObject of the parameter
* @throws ServiceException if there is a JSON error.
*/
public String[] getStringArray(String name, String[] def) throws ServiceException {
try {
JSONObject p = getParamMap().get(name);
return (p == null) ? def : JSONUtils.toStringArray(p.getJSONArray(VALUE));
} catch (JSONException ex) {
throw new ServiceException("No Value for " + name, ex);
}
}
/**
* Get a double[] parameter.
* @param name the parameter name.
* @return the parameter value as JSONObject.
* @throws ServiceException if there is no array.
*/
public double[] getDoubleArray(String name) throws ServiceException {
try {
JSONArray a = get(name).getJSONArray(VALUE);
return JSONUtils.toDoubleArray(a);
} catch (JSONException ex) {
throw new ServiceException("No Value for " + name, ex);
}
}
/**
* Get a double[] parameter.
* @param name the name of the parameter
* @param def the default value if parameter does not exist
* @return the JSONObject of the parameter
* @throws ServiceException if there is no array
*/
public double[] getDoubleArray(String name, double[] def) throws ServiceException {
try {
JSONObject p = getParamMap().get(name);
return (p == null) ? def : JSONUtils.toDoubleArray(p.getJSONArray(VALUE));
} catch (JSONException ex) {
throw new ServiceException("No Value for " + name, ex);
}
}
/**
* Get the unit of a parameter.
* @param name the parameter name
* @return the unit as string, 'null' if there is none.
* @throws ServiceException if unit is missing
*/
public String getUnit(String name) throws ServiceException {
try {
return get(name).getString(UNIT);
} catch (JSONException ex) {
throw new ServiceException("No unit for " + name);
}
}
/**
* Get the description of a parameter.
* @param name the parameter name
* @return the description as string, 'null' if there is none.
* @throws ServiceException if there is no description.
*/
public String getDescr(String name) throws ServiceException {
try {
return get(name).getString(KEY_DESC);
} catch (JSONException ex) {
throw new ServiceException("No description for " + name);
}
}
/**
* Get the geometry of a parameter.
* @param name the name if the parameter
* @return the geometry of a parameter
* @throws ServiceException if there is no geometry
*/
public JSONObject getGeometry(String name) throws ServiceException {
try {
return get(name).getJSONObject(GEOMETRY);
} catch (JSONException ex) {
throw new ServiceException("No geometry for " + name);
}
}
/**
* Get the parameter meta info.
*
* @param name the parameter name
* @param metaKey the meta data key
* @return the meta data value, or null is there is no key.
* @throws ServiceException if something goes wrong
*/
protected String getMetaInfo(String name, String metaKey) throws ServiceException {
JSONObject p = get(name);
try {
if (p.has(metaKey)) {
return p.getString(metaKey);
}
} catch (JSONException e) {
throw new ServiceException("error getting meta value '" + metaKey + "' for parameter: " + name);
}
return null;
}
/**
* Get the full JSON parameter record.
* @param name the parameter name
* @return the JSON record.
*/
private JSONObject get(String name) throws ServiceException {
JSONObject p = getParamMap().get(name);
if (p == null) {
throw new ServiceException("Parameter not found: '" + name + "'");
}
return p;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy