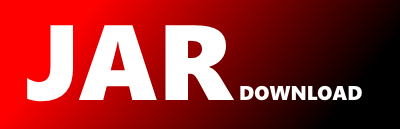
csip.utils.Execution Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of csip-core Show documentation
Show all versions of csip-core Show documentation
The Cloud Services Integration Platform is a SoA implementation to offer a Model-as-a-Service framework, Application Programming Interface, deployment infrastructure, and service implementations for environmental modeling.
/*
* $Id: Execution.java f6ae174e4534 2020-04-27 od $
*
* This file is part of the Cloud Services Integration Platform (CSIP),
* a Model-as-a-Service framework, API and application suite.
*
* 2012-2019, Olaf David and others, OMSLab, Colorado State University.
*
* OMSLab licenses this file to you under the MIT license.
* See the LICENSE file in the project root for more information.
*/
package csip.utils;
import csip.Executable.StdHandler;
import static csip.Executable.TIMED_OUT;
import csip.SessionLogger;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.OutputStreamWriter;
import java.io.Reader;
import java.io.Writer;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.concurrent.CountDownLatch;
import java.util.concurrent.TimeUnit;
import java.util.logging.Level;
/**
* Executable. Helper class to execute external programs.
*
* @author od
*/
class Execution {
final ProcessBuilder pb = new ProcessBuilder();
final File executable;
Object[] args = new Object[]{};
SessionLogger logger;
long time = 3600 * 24;
TimeUnit unit = TimeUnit.SECONDS;
//
Writer stderr = new OutputStreamWriter(System.err) {
@Override
public void close() throws IOException {
System.err.flush();
stderr_closed = -1;
}
};
//
Writer stdout = new OutputStreamWriter(System.out) {
@Override
public void close() throws IOException {
System.out.flush();
stdout_closed = -1;
}
};
StdHandler stdout_handler;
StdHandler stderr_handler;
// Do nothing.
private static class NOPWriter extends Writer {
@Override
public void write(char[] cbuf, int off, int len) throws IOException {
}
@Override
public void flush() throws IOException {
}
@Override
public void close() throws IOException {
}
}
/**
* Create a new ProcessExecution.
*
* @param executable the executable file.
*/
Execution(File executable) {
this.executable = executable;
}
File getFile() {
return executable;
}
/**
* Set the execution arguments.
*
* @param args the command line arguments
*/
void setArguments(Object... args) {
this.args = args;
}
/**
* Set the timeout.
*
* @param time the amount of the time unit.
* @param unit the TimeUnit
*/
void setTimeout(long time, TimeUnit unit) {
if (time < 1) {
throw new IllegalArgumentException("time: " + time);
}
if (unit == null) {
throw new IllegalArgumentException("time unit is null");
}
this.time = time;
this.unit = unit;
}
/**
* Set the execution arguments.
*
* @param args the command line arguments
*/
void setArguments(String... args) {
this.args = args;
}
/**
* Set the execution arguments.
*
* @param args the program arguments.
*/
void setArguments(List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy