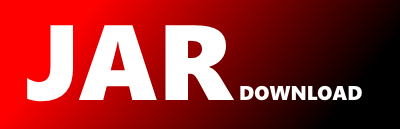
csip.utils.LRUCache Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of csip-core Show documentation
Show all versions of csip-core Show documentation
The Cloud Services Integration Platform is a SoA implementation to offer a Model-as-a-Service framework, Application Programming Interface, deployment infrastructure, and service implementations for environmental modeling.
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package csip.utils;
import java.util.LinkedHashMap;
import java.util.Map;
/**
* LRU cache.
*
* @author od
*/
public class LRUCache {
static final int DEFAULT_SIZE = 16;
Map m = new LinkedHashMap<>();
int size;
K _key;
V _val;
public LRUCache(int size) {
if (size < 1) {
throw new IllegalArgumentException("size >= 1!");
}
this.size = size;
}
public LRUCache() {
this(DEFAULT_SIZE);
}
public V put(K key, V val) {
V prev = m.put(_key = key, _val = val);
if (m.size() > size) {
// evict the first element
K first = m.keySet().iterator().next();
m.remove(first);
}
return prev;
}
public V get(K key) {
// accessing the last one again?
if (key.equals(_key)) {
return _val;
}
if (m.containsKey(key)) {
V val = m.get(key);
// remove it from wherever it is in the cache
m.remove(key);
// put it at the end.
m.put(_key = key, _val = val);
return val;
}
return null;
}
public void clear() {
m.clear();
}
public static void main(String[] args) {
LRUCache c = new LRUCache<>();
System.out.println(c.put("ff", "1"));
System.out.println(c.put("ff2", "2"));
System.out.println(c.put("ff3", "3"));
System.out.println(c.m);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy