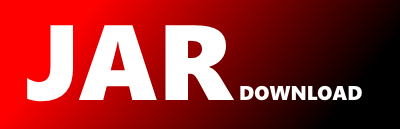
csip.utils.Validation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of csip-core Show documentation
Show all versions of csip-core Show documentation
The Cloud Services Integration Platform is a SoA implementation to offer a Model-as-a-Service framework, Application Programming Interface, deployment infrastructure, and service implementations for environmental modeling.
/*
* $Id:$
*
* This file is part of the Cloud Services Integration Platform (CSIP),
* a Model-as-a-Service framework, API and application suite.
*
* 2012-2019, Olaf David and others, OMSLab, Colorado State University.
*
* OMSLab licenses this file to you under the MIT license.
* See the LICENSE file in the project root for more information.
*/
package csip.utils;
/**
* Generic validation methods..
*
* @author od
*/
public class Validation {
static final double CONUS_NORTH = 49.3457868; // CONUS_NORTH lat
static final double CONUS_WEST = -124.7844079; // CONUS_WEST long
static final double CONUS_EAST = -66.9513812; // CONUS_EAST long
static final double CONUS_SOUTH = 24.7433195; // CONUS_SOUTH lat
static final int MAX_COKEY = 99999999; // Cokeys aren't longer than 8 digits
static final int MAX_MUKEY = 9999999; // Mukeys aren't longer than 7 digits
/**
* checks for the lat/lon to be in CONUS.
*
* @param lat latitude
* @param lon longitude
* @throws IllegalArgumentException if outside.
*/
public static void checkCONUS(double lat, double lon) {
if ((CONUS_SOUTH <= lat) && (lat <= CONUS_NORTH)
&& (CONUS_WEST <= lon) && (lon <= CONUS_EAST)) {
return;
}
throw new IllegalArgumentException("Invalid CONUS coordinates: " + lat + "/" + lon);
}
/**
* Check for valid Cokey.
*
* @param cokey the Cokey number as string, e.g. "12856507"
*/
public static void checkCokey(String cokey) {
if (cokey == null || cokey.isEmpty()) {
throw new IllegalArgumentException("No Cokey value:");
}
try {
checkCokey(new Integer(cokey));
} catch (NumberFormatException E) {
throw new IllegalArgumentException("Illegal Cokey value: " + cokey);
}
}
/**
* Check for valid Cokey.
*
* @param cokey the Cokey as number.
* @throws IllegalArgumentException if invalid
*/
public static void checkCokey(Number cokey) {
if (cokey == null) {
throw new IllegalArgumentException("No Cokey value:");
}
int val = cokey.intValue();
if (val >= 1 && val <= MAX_COKEY) {
return;
}
throw new IllegalArgumentException("Invalid Cokey: " + cokey);
}
/**
* Check for valid CMZ.
*
* @param cmz the CMZ number as string, e.g. "12' or "4.1", no CMZ prefix
* @throws IllegalArgumentException 74 PB 73 HI 72 AK 04.1 37.1 38.1 75 PR
* 15.1
*
*/
public static void checkCMZ(String cmz) {
if (cmz == null || cmz.isEmpty()) {
throw new IllegalArgumentException("No CMZ value:");
}
cmz = cmz.trim();
if (cmz.indexOf(' ') > 0) {
if (cmz.equals("72 AK") || cmz.equals("73 HI")
|| cmz.equals("74 PB") || cmz.equals("75 PR")) {
return;
}
}
try {
checkCMZ(new Double(cmz));
} catch (NumberFormatException E) {
throw new IllegalArgumentException("Illegal CMZ value: " + cmz);
}
}
/**
* Check for valid CMZ.
*
* @param cmz the CMZ as number.
* @throws IllegalArgumentException if invalid
*/
private static void checkCMZ(Number cmz) {
if (cmz == null) {
throw new IllegalArgumentException("No CMZ value:");
}
float val = cmz.floatValue();
if (val == 4.1f || val == 15.1f || val == 37.1f || val == 38.1f) {
return;
}
if (val >= 1 && val <= 71 && (val == (int) val)) {
return;
}
throw new IllegalArgumentException("Invalid CMZ: " + cmz);
}
/**
* Check for valid Mukey.
*
* @param mukey the Mukey number as string, e.g. "2955448"
*/
public static void checkMukey(String mukey) {
if (mukey == null || mukey.isEmpty()) {
throw new IllegalArgumentException("No Mukey value:");
}
try {
checkCokey(new Integer(mukey));
} catch (NumberFormatException E) {
throw new IllegalArgumentException("Illegal Mukey value: " + mukey);
}
}
/**
* Check for valid Mukey.
*
* @param mukey the Mukey as number.
* @throws IllegalArgumentException if invalid
*/
public static void checkMukey(Number mukey) {
if (mukey == null) {
throw new IllegalArgumentException("No Mukey value:");
}
int val = mukey.intValue();
if (val >= 1 && val <= MAX_MUKEY) {
return;
}
throw new IllegalArgumentException("Invalid Mukey: " + mukey);
}
public static void main(String[] args) {
checkCMZ("00012");
checkCMZ("04");
checkCMZ("04.1");
checkCMZ("15");
checkCMZ("73 HI");
checkCMZ(32.0f);
checkCokey("12856507");
checkMukey("2955448");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy