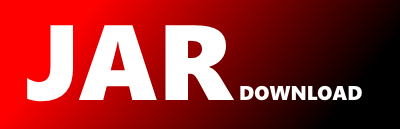
ngmf.util.PreProcessor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of oms Show documentation
Show all versions of oms Show documentation
Object Modeling System (OMS) is a pure Java object-oriented framework.
OMS v3.+ is a highly interoperable and lightweight modeling framework for component-based model and simulation development on multiple platforms.
/*
* $Id:$
*
* This file is part of the Object Modeling System (OMS),
* 2007-2012, Olaf David and others, Colorado State University.
*
* OMS is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, version 2.1.
*
* OMS is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with OMS. If not, see .
*/
package ngmf.util;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileReader;
import java.io.IOException;
import java.util.ArrayList;
import java.util.LinkedList;
import java.util.List;
/**
* Java/Groovy pre-processor, currently processing only '#include "???"'
* statements.
*
* @author od
*/
public class PreProcessor {
private static final String PP_INCLUDE = "#include";
private static List getFile(File file) throws IOException {
List lines = new ArrayList<>();
try (BufferedReader r = new BufferedReader(new FileReader(file))) {
String line;
while ((line = r.readLine()) != null) {
lines.add(line);
}
}
return lines;
}
private static String toString(List lines) {
StringBuilder b = new StringBuilder();
for (String line : lines) {
b.append(line).append('\n');
}
return b.toString();
}
private static List processFile(File file, LinkedList incl) throws IOException {
incl.push(file);
List lines = getFile(file);
int linescount = lines.size();
int i = 0;
do {
String line = lines.get(i);
if (line.trim().startsWith(PP_INCLUDE)) { // this will allow also comments : // include...
File inc = parseIncludeName(file, line, incl);
if (incl.contains(inc)) {
throw new RuntimeException(file + ": circular " + PP_INCLUDE + " of:" + inc.toString());
}
List l = processFile(inc, incl);
lines.remove(i); // remove the '#include' line
lines.addAll(i, l); // replace with content of the file.
i += l.size() - 1; // adjust looping variables.
linescount += l.size() - 1;
}
} while (++i < linescount);
incl.pop();
return lines;
}
private static File parseIncludeName(File file, String line, LinkedList incl) {
int count = line.length() - line.replace("\"", "").length();
if (count != 2) {
throw new RuntimeException(file + ": incorrect " + PP_INCLUDE + " statement '" + line + "'");
}
try {
String a = line.substring(line.indexOf("\"") + 1, line.lastIndexOf("\"")).trim();
if (a == null || a.isEmpty()) {
throw new RuntimeException(file + ": empty " + PP_INCLUDE + " file: " + line);
}
File f = new File(a); // absolute file
if (f.exists() && f.canRead() && f.isFile()) {
return f;
}
for (File i : incl) {
f = new File(i.getParentFile(), a); // relative file, use dir of prev includes
if (f.exists() && f.canRead() && f.isFile()) {
return f;
}
}
throw new RuntimeException(file + ": " + PP_INCLUDE + " file not found: '" + a + "'");
} catch (IndexOutOfBoundsException E) {
throw new RuntimeException(file + ": incorrect " + PP_INCLUDE + " statement: '" + line + "'");
}
}
/**
* Resolves preprocessing commands while getting the file content.
*
* @param f the src file
* @return the content of f parsed and processed
* @throws IOException produced by failed or interrupted I/O operations
*/
public static String getContent(File f) throws IOException {
List l = processFile(f, new LinkedList());
return toString(l);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy