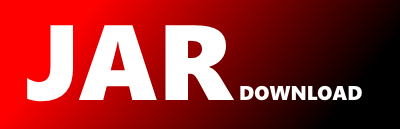
oms3.doc.Documents Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of oms Show documentation
Show all versions of oms Show documentation
Object Modeling System (OMS) is a pure Java object-oriented framework.
OMS v3.+ is a highly interoperable and lightweight modeling framework for component-based model and simulation development on multiple platforms.
/*
* $Id: Documents.java 7cba5ba59d73 2018-11-29 [email protected] $
*
* This file is part of the Object Modeling System (OMS),
* 2007-2012, Olaf David and others, Colorado State University.
*
* OMS is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, version 2.1.
*
* OMS is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with OMS. If not, see .
*/
package oms3.doc;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.PrintStream;
import java.lang.reflect.Field;
import java.net.MalformedURLException;
import java.net.URI;
import java.net.URISyntaxException;
import java.net.URL;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.Comparator;
import java.util.Date;
import java.util.HashMap;
import java.util.List;
import java.util.Locale;
import java.util.Map;
import java.util.ResourceBundle;
import java.util.StringTokenizer;
import oms3.annotations.*;
import oms3.io.CSProperties;
import oms3.util.Components;
/** Documenting a Model.
*
* @author od
*/
public class Documents {
/** Document
*
* @param file the xml outputfile.
* @param comp the component to document
* @param params the model parameter
* @param title the title
* @throws FileNotFoundException attempt to open a file has failed
*/
public static void db5Sim(File file, Class comp, CSProperties params, String title) throws FileNotFoundException {
db5Sim(file, comp, params, title, Locale.getDefault());
}
public static void db5Sim(File file, Class comp, CSProperties params, String title, Locale loc) throws FileNotFoundException {
DB5 db5 = new DB5(loc);
db5.generateDB5(file, comp, params, title);
}
/**
*
*/
static class DB5 {
private static String db50 = "";
Locale loc;
ResourceBundle b;
DB5(Locale loc) {
this.loc = loc;
b = ResourceBundle.getBundle("oms3.doc.Loc", loc);
}
void generateDB5(File file, Class comp, CSProperties params, String title) throws FileNotFoundException {
SimpleDateFormat df = new SimpleDateFormat(b.getString("date_format"), loc);
PrintStream f = new PrintStream(file);
f.println(db50);
f.println("");
f.println("" + title + " ");
f.println("" + b.getString("subtitle") + " ");
f.println("" + df.format(new Date()) + " ");
f.println(" ");
if (comp != null) {
Collection> c = Components.internalComponents(comp);
f.println(getComponentsChapter(c));
}
if (params != null) {
f.println(getParamChapter(params));
}
f.println(" ");
f.println(" ");
f.close();
}
String getParamChapter(CSProperties p) {
StringBuilder db = new StringBuilder();
db.append("");
db.append("" + b.getString("parameterset") + " ");
db.append(paramList("Parameter", p));
db.append(" ");
return db.toString();
}
String paramList(String title, CSProperties p) {
if (p.size() == 0) {
return "";
}
List keys = new ArrayList(p.keySet());
Collections.sort(keys);
StringBuilder db = new StringBuilder();
db.append("");
for (String name : keys) {
db.append(varlistentry(name, p.get(name).toString(), p.getInfo(name)));
}
db.append(" ");
return db.toString();
}
String varlistentry(String name, String item, Map info) {
StringBuilder db = new StringBuilder();
db.append("" + name + " ");
db.append("" + name + " (" + b.getString("parameter") + ") "
+ "Value ");
String descr = info.get("descr");
if (descr != null) {
db.append(" - " + descr);
}
db.append(" ");
db.append("");
db.append(item);
db.append("
");
for (String key : info.keySet()) {
if (!key.equals("descr")) {
db.append("" + key + " - " + info.get(key) + " ");
}
}
db.append(" ");
db.append(" ");
return db.toString();
}
String classSection(Class> c) {
StringBuilder db = new StringBuilder();
db.append("");
db.append("" + b.getString("component") + " '" + c.getSimpleName() + "' ");
db.append("" + c.getSimpleName() + " (" + b.getString("component") + ") ");
Keywords keywords = (Keywords) c.getAnnotation(Keywords.class);
if (keywords != null && !keywords.value().isEmpty()) {
StringTokenizer t = new StringTokenizer(keywords.value(), ",");
while (t.hasMoreTokens()) {
db.append("" + t.nextToken().trim() + " (" + b.getString("keyword") + ") "
+ c.getSimpleName() + " ");
}
}
Description descr = (Description) c.getAnnotation(Description.class);
if (descr != null) {
String d = locDesc(descr, loc);
db.append(" ");
}
db.append("");
db.append(varlistentry(b.getString("name"), "" + c.getName() + "
"));
Author author = (Author) c.getAnnotation(Author.class);
if (author != null) {
StringTokenizer t = new StringTokenizer(author.name(), ",");
StringBuilder authindex = new StringBuilder();
while (t.hasMoreTokens()) {
authindex.append("" + t.nextToken().trim() + " (" + b.getString("author") + ") "
+ c.getSimpleName() + " ");
}
db.append(varlistentry(b.getString("author"), author.name() + (author.contact().isEmpty() ? "" : " - " + author.contact()),
authindex.toString()));
}
if (keywords != null && !keywords.value().isEmpty()) {
db.append(varlistentry(b.getString("keyword"), keywords.value()));
}
VersionInfo version = (VersionInfo) c.getAnnotation(VersionInfo.class);
if (version != null) {
db.append(varlistentry(b.getString("version"), version.value()));
}
SourceInfo source = (SourceInfo) c.getAnnotation(SourceInfo.class);
if (source != null) {
String src = source.value();
// if (src.startsWith("$") && src.endsWith("$")) {
// src = src.substring(src.indexOf(' ') + 1, src.lastIndexOf(' '));
// }
db.append(varlistentry(b.getString("source"), src));
}
License lic = (License) c.getAnnotation(License.class);
if (lic != null) {
db.append(varlistentry(b.getString("license"), lic.value()));
}
db.append(" ");
db.append(table(b.getString("parameter"), b.getString("parameter"), c, Components.parameter(c)));
db.append(table(b.getString("var_in"), b.getString("variable"), c, Components.inVars(c)));
db.append(table(b.getString("var_out"), b.getString("variable"), c, Components.outVars(c)));
Bibliography biblio = (Bibliography) c.getAnnotation(Bibliography.class);
if (biblio != null) {
db.append("");
db.append("" + b.getString("bibliography")+ " ");
db.append("");
StringBuffer bs = new StringBuffer("");
for (String entry : biblio.value()) {
bs.append("" + entry + " ");
}
bs.append(" ");
db.append(varlistentry("", bs.toString()));
db.append(" ");
db.append(" ");
}
Documentation doc = (Documentation) c.getAnnotation(Documentation.class);
if (doc != null) {
String v = doc.value();
if (v.endsWith(".xml")) {
try {
URL url = new URL(v);
db.append(" ");
} catch (MalformedURLException E) {
try {
File rel = new File(v);
// relative reference "src/...."
if (!rel.isAbsolute() && System.getProperty("oms3.work") != null) {
File work = new File(System.getProperty("oms3.work"));
File locF = locFile(work, v, loc);
v = locF.toString().replace('\\', '/');
}
File f = new File(v);
if (f.exists()) {
db.append(" ");
} else {
System.out.println("Document not found: " + v);
}
} catch (IllegalArgumentException iae) {
iae.printStackTrace(System.out);
System.out.println("Illegal Argument: " + iae.getMessage() + " " + v);
}
}
} else {
db.append("" + b.getString("further") + " ");
db.append("");
db.append(v);
db.append(" ");
db.append(" ");
}
}
db.append(" ");
return db.toString();
}
@SuppressWarnings("unchecked")
String getComponentsChapter(Collection> comps) {
StringBuffer db = new StringBuffer();
// Model component
db.append("");
db.append("" + b.getString("model") + " ");
Class mai = comps.iterator().next(); // the first component is top.
db.append(classSection(mai));
db.append(" ");
comps.remove(mai); // remove the main component
Map>> pmap = categorize(comps);
List pl = new ArrayList(pmap.keySet());
Collections.sort(pl, new Comparator() {
@Override
public int compare(Package o1, Package o2) {
return o1.getName().compareToIgnoreCase(o2.getName());
}
});
// Subcomponents.
db.append("");
db.append("" + b.getString("sub") + " ");
for (Package p : pl) {
db.append("");
db.append("'" + p.getName() + "' ");
List> co = pmap.get(p);
Collections.sort(co, new Comparator>() {
@Override
public int compare(Class o1, Class o2) {
return o1.getSimpleName().compareToIgnoreCase(o2.getSimpleName());
}
});
for (Class c : co) {
db.append(classSection(c));
}
db.append(" ");
}
db.append(" ");
return db.toString();
}
String table(String title, String cl, Class comp, List l) {
if (l.size() == 0) {
return "";
}
Collections.sort(l, new Comparator() {
@Override
public int compare(Field o1, Field o2) {
return o1.getName().compareToIgnoreCase(o2.getName());
}
});
StringBuffer db = new StringBuffer();
db.append("");
db.append("" + title + " ");
db.append("");
for (Field field : l) {
db.append("" + field.getName() + " ");
Unit unit = field.getAnnotation(Unit.class);
if (unit != null) {
db.append(" [");
db.append(unit.value());
db.append("]
");
}
db.append(" - " + field.getType().getSimpleName() + "
");
Range range = field.getAnnotation(Range.class);
if (range != null) {
db.append(" (");
db.append(range.min() == Double.MIN_VALUE ? "" : range.min());
db.append(" ... ");
db.append(range.max() == Double.MAX_VALUE ? "" : range.max());
db.append(")");
}
db.append("" + field.getName() + " (" + cl + ") "
+ field.getDeclaringClass().getSimpleName() + " ");
db.append(" ");
db.append("");
Description descr = field.getAnnotation(Description.class);
if (descr != null) {
String d = locDesc(descr, loc);
db.append("");
}
db.append(" ");
db.append(" ");
}
db.append(" ");
db.append(" ");
return db.toString();
}
static String varlistentry(String name, String item) {
return varlistentry(name, item, "");
}
static String varlistentry(String name, String item, String indexterm) {
StringBuffer db = new StringBuffer();
db.append("" + name + " " + indexterm + " ");
db.append("");
db.append(item);
db.append(" ");
db.append(" ");
return db.toString();
}
static Map>> categorize(Collection> comp) {
Map>> packages = new HashMap>>();
for (Class> c : comp) {
Package p = c.getPackage();
List> tos = packages.get(p);
if (tos == null) {
tos = new ArrayList>();
packages.put(p, tos);
}
tos.add(c);
}
return packages;
}
static File locFile(File work, String descr, Locale l) {
if (l == null) {
return new File(work, descr);
}
String locName = descr.substring(0, descr.lastIndexOf('.'));
String locExt = descr.substring(descr.lastIndexOf('.'));
String locLan = l.getLanguage();
File f = new File(work, locName + "_" + locLan + locExt);
if (f.exists()) {
return f;
}
f = new File(work, descr);
if (f.exists()) {
return f;
}
throw new IllegalArgumentException(descr);
}
static String locDesc(Description descr, Locale l) {
if (l == null) {
return descr.value();
}
if (l.getLanguage().equals("en")) {
return descr.en().isEmpty() ? descr.value() : descr.en();
} else if (l.getLanguage().equals("de")) {
return descr.de().isEmpty() ? descr.value() : descr.de();
}
return descr.value();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy