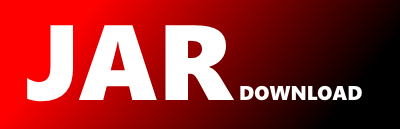
edu.emory.mathcs.nlp.common.constituent.CTLib Maven / Gradle / Ivy
The newest version!
/**
* Copyright 2015, Emory University
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package edu.emory.mathcs.nlp.common.constituent;
import java.util.List;
import java.util.Set;
import java.util.function.Predicate;
import java.util.regex.Pattern;
/**
* @author Jinho D. Choi ({@code [email protected]})
*/
public class CTLib
{
static public String toForms(List tokens, int beginIndex, int endIndex, String delim)
{
StringBuilder build = new StringBuilder();
int i;
for (i=beginIndex; i matchC(String constituentTag)
{
return node -> node.isConstituentTag(constituentTag);
}
static public Predicate matchCo(String... constituentTags)
{
return node -> node.isConstituentTagAny(constituentTags);
}
static public Predicate matchCo(Set constituentTags)
{
return node -> constituentTags.contains(node.getConstituentTag());
}
static public Predicate matchCp(String constituentPrefix)
{
return node -> node.getConstituentTag().startsWith(constituentPrefix);
}
static public Predicate matchCF(String constituentTag, String functionTag)
{
return node -> node.isConstituentTag(constituentTag) && node.hasFunctionTag(functionTag);
}
static public Predicate matchCFa(String constituentTag, String... functionTags)
{
return node -> node.isConstituentTag(constituentTag) && node.hasFunctionTagAll(functionTags);
}
static public Predicate matchCFo(String constituentTag, String... functionTags)
{
return node -> node.isConstituentTag(constituentTag) && node.hasFunctionTagAny(functionTags);
}
static public Predicate matchF(String functionTag)
{
return node -> node.hasFunctionTag(functionTag);
}
static public Predicate matchFa(String... functionTags)
{
return node -> node.hasFunctionTagAll(functionTags);
}
static public Predicate matchFo(String... functionTags)
{
return node -> node.hasFunctionTagAny(functionTags);
}
static public Predicate matchP(Pattern constituentPattern)
{
return node -> node.matchesConstituentTag(constituentPattern);
}
static public Predicate matchPFa(Pattern constituentPattern, String... functionTags)
{
return node -> node.matchesConstituentTag(constituentPattern) && node.hasFunctionTagAll(functionTags);
}
static public Predicate matchPFo(Pattern constituentPattern, String... functionTags)
{
return node -> node.matchesConstituentTag(constituentPattern) && node.hasFunctionTagAny(functionTags);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy