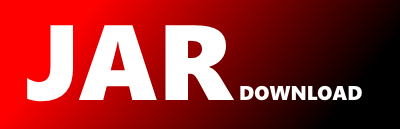
edu.hm.hafner.analysis.AntModuleDetector Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of analysis-model Show documentation
Show all versions of analysis-model Show documentation
This library provides a Java object model to read, aggregate, filter, and query static analysis reports.
It is used by Jenkins' warnings next generation plug-in to visualize the warnings of individual builds.
Additionally, this library is used by a GitHub action to autograde student software projects based on a given set of
metrics (unit tests, code and mutation coverage, static analysis warnings).
package edu.hm.hafner.analysis;
import java.io.IOException;
import java.io.InputStream;
import java.nio.file.InvalidPathException;
import java.util.List;
import java.util.Map;
import org.apache.commons.lang3.StringUtils;
import org.xml.sax.SAXException;
import edu.hm.hafner.analysis.ModuleDetector.FileSystem;
/**
* Detects module names by parsing the name of a source file, the ANT build.xml.
*/
public class AntModuleDetector extends AbstractModuleDetector {
static final String ANT_PROJECT = "build.xml";
AntModuleDetector(final FileSystem fileSystem) {
super(fileSystem);
}
@Override
String getPattern() {
return ALL_DIRECTORIES + ANT_PROJECT;
}
@Override
void collectProjects(final Map mapping, final List projects) {
for (String fileName : projects) {
if (fileName.endsWith(ANT_PROJECT)) {
addMapping(mapping, fileName, ANT_PROJECT, parseBuildXml(fileName));
}
}
}
/**
* Returns the project name stored in the Ant build.xml.
*
* @param buildXml
* Ant build.xml file name
*
* @return the project name or an empty string if the name could not be resolved
*/
private String parseBuildXml(final String buildXml) {
try (InputStream file = getFactory().open(buildXml)) {
SecureDigester digester = new SecureDigester(ModuleDetector.class);
digester.push(new StringBuilder());
String xPath = "project";
digester.addCallMethod(xPath, "append", 1);
digester.addCallParam(xPath, 0, "name");
StringBuilder result = digester.parse(file);
return result.toString();
}
catch (IOException | SAXException | InvalidPathException ignored) {
// ignore
}
return StringUtils.EMPTY;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy