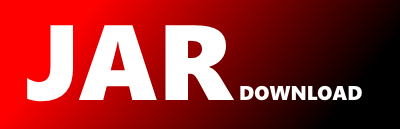
edu.hm.hafner.analysis.parser.CodeCheckerParser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of analysis-model Show documentation
Show all versions of analysis-model Show documentation
This library provides a Java object model to read, aggregate, filter, and query static analysis reports.
It is used by Jenkins' warnings next generation plug-in to visualize the warnings of individual builds.
Additionally, this library is used by a GitHub action to autograde student software projects based on a given set of
metrics (unit tests, code and mutation coverage, static analysis warnings).
package edu.hm.hafner.analysis.parser;
import java.util.Optional;
import java.util.regex.Matcher;
import edu.hm.hafner.analysis.Issue;
import edu.hm.hafner.analysis.IssueBuilder;
import edu.hm.hafner.analysis.LookaheadParser;
import edu.hm.hafner.analysis.Severity;
import edu.hm.hafner.util.LookaheadStream;
/**
* A parser for the clang-tidy static analysis warnings parsed by Codechecker. Codechecker parses the {@code *.plist}
* files and converts it into plain text file.
*
*
* Better for human readers and for using grep and diff. It also puts the human-readable Severity at the start of a
* line.
*
*/
public class CodeCheckerParser extends LookaheadParser {
private static final long serialVersionUID = -3015592762345283582L;
private static final String CODE_CHECKER_DEFECT_PATTERN =
"^\\[(?CRITICAL|HIGH|MEDIUM|LOW|UNSPECIFIED|STYLE)\\] (?.+):(?\\d+):(?\\d+): (?.*?) \\[(?[^\\s]*?)\\]$";
/**
* Creates a new instance of {@link CodeCheckerParser}.
*/
public CodeCheckerParser() {
super(CODE_CHECKER_DEFECT_PATTERN);
}
@Override
protected Optional createIssue(final Matcher matcher, final LookaheadStream lookahead,
final IssueBuilder builder) {
Severity severity = getSeverity(matcher.group("severity"));
return builder.setFileName(matcher.group("path"))
.setSeverity(severity)
.setLineStart(matcher.group("line"))
.setColumnStart(matcher.group("column"))
.setCategory(matcher.group("category"))
.setMessage(matcher.group("message"))
.buildOptional();
}
private Severity getSeverity(final String severityText) {
if (severityText.contains("CRITICAL")) {
return Severity.ERROR;
}
if (severityText.contains("HIGH")) {
return Severity.WARNING_HIGH;
}
if (severityText.contains("MEDIUM")) {
return Severity.WARNING_NORMAL;
}
return Severity.WARNING_LOW;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy