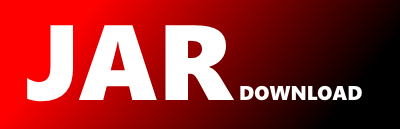
edu.hm.hafner.analysis.parser.DockerLintParser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of analysis-model Show documentation
Show all versions of analysis-model Show documentation
This library provides a Java object model to read, aggregate, filter, and query static analysis reports.
It is used by Jenkins' warnings next generation plug-in to visualize the warnings of individual builds.
Additionally, this library is used by a GitHub action to autograde student software projects based on a given set of
metrics (unit tests, code and mutation coverage, static analysis warnings).
package edu.hm.hafner.analysis.parser;
import org.json.JSONArray;
import org.json.JSONObject;
import edu.hm.hafner.analysis.Issue;
import edu.hm.hafner.analysis.IssueBuilder;
import edu.hm.hafner.analysis.Report;
import edu.hm.hafner.analysis.Severity;
/**
* A parser for dockerlint json output.
*
*
* Possible usage via docker is:
*
* {@code
*
* docker run -it --rm -v $PWD:/root/ \
* projectatomic/dockerfile-lint \
* dockerfile_lint -j -f Dockerfile.
*
}
*
* @author Andreas Mandel
* @see dockerlint
*/
public class DockerLintParser extends JsonIssueParser {
private static final long serialVersionUID = -4077698163775928314L;
@Override
protected void parseJsonObject(final Report report, final JSONObject jsonReport, final IssueBuilder issueBuilder) {
for (String severityGroupName : jsonReport.keySet()) {
Object severityGroup = jsonReport.get(severityGroupName);
if (severityGroup instanceof JSONObject) {
JSONArray data = ((JSONObject) severityGroup).optJSONArray("data");
for (Object issue : data) {
if (issue instanceof JSONObject) {
report.add(convertToIssue((JSONObject) issue, issueBuilder));
}
}
}
}
}
private Issue convertToIssue(final JSONObject jsonIssue, final IssueBuilder builder) {
StringBuilder message = new StringBuilder();
message.append(jsonIssue.optString("message"));
if (jsonIssue.has("description")) {
message.append(" - ");
message.append(jsonIssue.getString("description"));
}
if (jsonIssue.has("reference_url")) {
Object refUrl = jsonIssue.get("reference_url");
message.append(" See ");
message.append(collapseReferenceUrl(refUrl));
}
builder.setMessage(message.toString());
builder.setSeverity(toSeverity(jsonIssue.optString("level")));
builder.setLineStart(jsonIssue.optInt("line", -1));
builder.setCategory(jsonIssue.optString("label", null));
builder.setFileName("Dockerfile"); // simpler than reading "lineContent" & "instruction" & "count" & "regex"
return builder.buildAndClean();
}
private String collapseReferenceUrl(final Object refUrl) {
StringBuilder referenceUrl = new StringBuilder();
if (refUrl instanceof JSONArray) {
for (Object part : (JSONArray) refUrl) {
referenceUrl.append(part);
}
}
else {
referenceUrl.append(refUrl);
}
return referenceUrl.toString();
}
private Severity toSeverity(final String level) {
switch (level) {
case "warn":
return Severity.WARNING_NORMAL;
case "error":
return Severity.WARNING_HIGH;
default:
return Severity.WARNING_LOW;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy