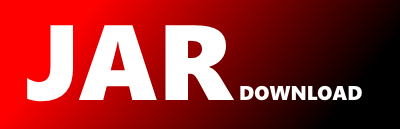
edu.hm.hafner.analysis.parser.ErlcParser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of analysis-model Show documentation
Show all versions of analysis-model Show documentation
This library provides a Java object model to read, aggregate, filter, and query static analysis reports.
It is used by Jenkins' warnings next generation plug-in to visualize the warnings of individual builds.
Additionally, this library is used by a GitHub action to autograde student software projects based on a given set of
metrics (unit tests, code and mutation coverage, static analysis warnings).
package edu.hm.hafner.analysis.parser;
import java.util.Optional;
import java.util.regex.Matcher;
import edu.hm.hafner.analysis.Issue;
import edu.hm.hafner.analysis.IssueBuilder;
import edu.hm.hafner.analysis.LookaheadParser;
import edu.hm.hafner.analysis.Severity;
import edu.hm.hafner.util.LookaheadStream;
/**
* A parser for the erlc compiler warnings.
*
* @author Stefan Brausch
*/
public class ErlcParser extends LookaheadParser {
private static final long serialVersionUID = 8986478184830773892L;
private static final String ERLC_WARNING_PATTERN = "^(.+\\.(?:erl|yrl|mib|bin|rel|asn1|idl)):(\\d*): ([wW]arning:"
+ " )?(.+)$";
/**
* Creates a new instance of {@link ErlcParser}.
*/
public ErlcParser() {
super(ERLC_WARNING_PATTERN);
}
@Override
protected Optional createIssue(final Matcher matcher, final LookaheadStream lookahead,
final IssueBuilder builder) {
Severity priority;
String category;
String categoryMatch = matcher.group(3);
if (equalsIgnoreCase(categoryMatch, "warning: ")) {
priority = Severity.WARNING_NORMAL;
category = categoryMatch.substring(0, categoryMatch.length() - 2);
}
else {
priority = Severity.WARNING_HIGH;
category = "Error";
}
return builder.setFileName(matcher.group(1))
.setLineStart(matcher.group(2))
.setCategory(category)
.setMessage(matcher.group(4))
.setSeverity(priority)
.buildOptional();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy