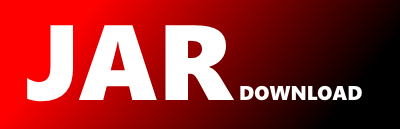
edu.hm.hafner.analysis.registry.CompositeParserDescriptor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of analysis-model Show documentation
Show all versions of analysis-model Show documentation
This library provides a Java object model to read, aggregate, filter, and query static analysis reports.
It is used by Jenkins' warnings next generation plug-in to visualize the warnings of individual builds.
Additionally, this library is used by a GitHub action to autograde student software projects based on a given set of
metrics (unit tests, code and mutation coverage, static analysis warnings).
package edu.hm.hafner.analysis.registry;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import edu.hm.hafner.analysis.IssueParser;
import edu.hm.hafner.analysis.ReaderFactory;
import edu.hm.hafner.analysis.Report;
/**
* A {@link CompositeParserDescriptor} is composed of several tools. Every parser of this suite will be called on the
* input file, the results will be aggregated afterward.
*
* @author Ullrich Hafner
*/
abstract class CompositeParserDescriptor extends ParserDescriptor {
/**
* Creates a new {@link ParserDescriptor} instance.
*
* @param id
* the technical ID
* @param name
* the name of the parser
*/
CompositeParserDescriptor(final String id, final String name) {
super(id, name);
}
@Override
public final IssueParser createParser(final Option... options) {
return new CompositeParser(createParsers());
}
/**
* Returns a collection of parsers to scan a log file and return the issues reported in such a file.
*
* @return the parsers to use
*/
protected abstract Collection extends IssueParser> createParsers();
/**
* Wraps all parsers into a collection.
*
* @param parser
* the parser to wrap
*
* @return a singleton collection
*/
protected Collection extends IssueParser> asList(final IssueParser... parser) {
List parsers = new ArrayList<>();
Collections.addAll(parsers, parser);
return parsers;
}
/**
* Combines several parsers into a single composite parser. The issues of all the individual parsers will be
* aggregated.
*/
private static class CompositeParser extends IssueParser {
private static final long serialVersionUID = -2319098057308618997L;
private final List parsers = new ArrayList<>();
/**
* Creates a new instance of {@link CompositeParser}.
*
* @param parsers
* the parsers to use to scan the input files
*/
CompositeParser(final Collection extends IssueParser> parsers) {
super();
this.parsers.addAll(parsers);
}
@Override
public Report parse(final ReaderFactory readerFactory) {
Report aggregated = new Report();
for (IssueParser parser : parsers) {
if (parser.accepts(readerFactory)) {
aggregated.addAll(parser.parse(readerFactory));
}
}
return aggregated;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy