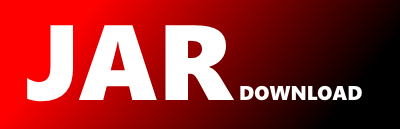
edu.internet2.middleware.grouper.grouperUi.beans.json.AppState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of grouper-ui Show documentation
Show all versions of grouper-ui Show documentation
Internet2 Groups Management User Interface
/*******************************************************************************
* Copyright 2012 Internet2
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
******************************************************************************/
package edu.internet2.middleware.grouper.grouperUi.beans.json;
import java.io.Serializable;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.Set;
import javax.servlet.http.HttpServletRequest;
import org.apache.commons.lang.StringUtils;
import edu.internet2.middleware.grouper.grouperUi.beans.SessionContainer;
import edu.internet2.middleware.grouper.ui.GrouperUiFilter;
import edu.internet2.middleware.grouper.util.GrouperUtil;
/**
* AppState object comes from javascript on ajax requests
*/
@SuppressWarnings("serial")
public class AppState implements Serializable {
/** constant for field name for: hideShows */
public static final String FIELD_HIDE_SHOWS = "hideShows";
/** constant for field name for: initted */
public static final String FIELD_INITTED = "initted";
/** constant for field name for: inittedSimpleMembershipUpdate */
public static final String FIELD_INITTED_SIMPLE_MEMBERSHIP_UPDATE = "inittedSimpleMembershipUpdate";
/** constant for field name for: pagers */
public static final String FIELD_PAGERS = "pagers";
/** constant for field name for: urlArgObjects */
public static final String FIELD_URL_ARG_OBJECTS = "urlArgObjects";
/** constant for field name for: urlInCache */
public static final String FIELD_URL_IN_CACHE = "urlInCache";
/**
* fields which are included in clone method
*/
private static final Set CLONE_FIELDS = GrouperUtil.toSet(
FIELD_HIDE_SHOWS, FIELD_INITTED, FIELD_INITTED_SIMPLE_MEMBERSHIP_UPDATE,
FIELD_PAGERS, FIELD_URL_ARG_OBJECTS, FIELD_URL_IN_CACHE);
/** if simple membership update is initted */
private boolean inittedSimpleMembershipUpdate;
/**
* get an app state object or param
* @param name
* @return the value
*/
public String getUrlArgObjectOrParam(String name) {
HttpServletRequest request = GrouperUiFilter.retrieveHttpServletRequest();
String result = null;
if (this.getUrlArgObjects() != null) {
result = this.getUrlArgObjects().get(name);
}
if (StringUtils.isBlank(result)) {
result = request.getParameter(name);
}
return result;
}
/**
* if simple membership update is initted
* @return the inittedSimpleMembershipUpdate
*/
public boolean isInittedSimpleMembershipUpdate() {
return this.inittedSimpleMembershipUpdate;
}
/**
* if simple membership update is initted
* @param inittedSimpleMembershipUpdate1 the inittedSimpleMembershipUpdate to set
*/
public void setInittedSimpleMembershipUpdate(boolean inittedSimpleMembershipUpdate1) {
this.inittedSimpleMembershipUpdate = inittedSimpleMembershipUpdate1;
}
/** placeholder since safari sends back this function
* return null
* @return url arg object map
*/
public Object getUrlArgObjectMap() {
return null;
}
/** placeholder since safari sends back this function
* @param urlArgObjectMap
*/
public void setUrlArgObjectMap(Object urlArgObjectMap) {
//no need to do anything here
}
/** if the javascript is initted */
private boolean initted;
/**
* store to request scope
*/
public void storeToRequest() {
HttpServletRequest httpServletRequest = GrouperUiFilter.retrieveHttpServletRequest();
httpServletRequest.setAttribute("appState", this);
}
/**
* retrieveFromRequest, must not be null
* @return the app state in request scope
*/
public static AppState retrieveFromRequest() {
HttpServletRequest httpServletRequest = GrouperUiFilter.retrieveHttpServletRequest();
AppState appState = (AppState)httpServletRequest.getAttribute("appState");
if (appState == null) {
throw new RuntimeException("App state is null");
}
return appState;
}
/**
* if the javascript is initted
* @return the initted
*/
public boolean isInitted() {
return this.initted;
}
/**
* if the javascript is initted
* @param initted1 the initted to set
*/
public void setInitted(boolean initted1) {
this.initted = initted1;
}
/**
*
*/
public AppState() {
}
/**
* init a request with app state (from browser)
*/
public void initRequest() {
SessionContainer sessionContainer = SessionContainer.retrieveFromSession();
{
//save screen state of all the hide shows which are in session
Map appStateHideShows = this.getHideShows();
if (appStateHideShows != null) {
Map newAppStateHideShows = new LinkedHashMap();
for (String hideShowName : appStateHideShows.keySet()) {
//clone this
GuiHideShow guiHideShow1 = appStateHideShows.get(hideShowName);
GuiHideShow appStateHideShow = guiHideShow1.clone();
newAppStateHideShows.put(hideShowName, appStateHideShow);
}
this.setHideShows(newAppStateHideShows);
appStateHideShows = newAppStateHideShows;
for (String hideShowName : appStateHideShows.keySet()) {
GuiHideShow sessionHideShow = sessionContainer.getHideShows().get(hideShowName);
if (sessionHideShow != null) {
//copy over the current state
GuiHideShow appStateHideShow = appStateHideShows.get(hideShowName);
sessionHideShow.setShowing( appStateHideShow.isShowing());
}
}
}
}
{
//convert pagers to real object model
Map appStatePagers = this.getPagers();
if (appStatePagers != null) {
Map newAppStatePagers = new LinkedHashMap();
for (String pagerName : appStatePagers.keySet()) {
//morph this
GuiPaging guiPaging1 = appStatePagers.get(pagerName);
GuiPaging appStateHideShow = guiPaging1.clone();
newAppStatePagers.put(pagerName, appStateHideShow);
}
this.setPagers(newAppStatePagers);
}
}
this.storeToRequest();
}
/**
* url in cache
*/
private String urlInCache;
/**
* urlInCache
* @return the urlInCache
*/
public String getUrlInCache() {
return this.urlInCache;
}
/**
* urlInCache
* @param urlInCache1 the urlInCache to set
*/
public void setUrlInCache(String urlInCache1) {
this.urlInCache = urlInCache1;
}
/**
*
* hide shows, the name, and if showing, text, etc. Anything with class:
* shows_hideShowName, e.g. shows_simpleMembershipAdvanced
* Anything with class: hides_hideShowName, e.g. hides_simpleMembershipAdvanced
* will show if false.
*
*/
private Map hideShows = new LinkedHashMap();
/**
*
* hide shows, the name, and if showing, text, etc. Anything with class:
* shows_hideShowName, e.g. shows_simpleMembershipAdvanced
* Anything with class: hides_hideShowName, e.g. hides_simpleMembershipAdvanced
* will show if false.
*
* @return the hideShows
*/
public Map getHideShows() {
return this.hideShows;
}
/**
* deep clone the fields in this object
*/
@Override
public AppState clone() {
return GrouperUtil.clone(this, CLONE_FIELDS);
}
/**
*
* hide shows, the name, and if showing, text, etc. Anything with class:
* shows_hideShowName, e.g. shows_simpleMembershipAdvanced
* Anything with class: hides_hideShowName, e.g. hides_simpleMembershipAdvanced
* will show if false.
*
* @param hideShows1 the hideShows to set
*/
public void setHideShows(Map hideShows1) {
this.hideShows = hideShows1;
}
/**
*
* pagers keep track of which page and how many on a page
*
* @return the pagers
*/
public Map getPagers() {
return this.pagers;
}
/**
*
* pagers keep track of which page and how many on a page
*
* @param pagers1 the pagers to set
*/
public void setPagers(Map pagers1) {
this.pagers = pagers1;
}
/**
*
* pagers keep track of which page and how many on a page
*
*/
private Map pagers = new LinkedHashMap();
/**
* url split out into args
*/
private Map urlArgObjects = new LinkedHashMap();
/**
* @return the urlArgObjects
*/
public Map getUrlArgObjects() {
return this.urlArgObjects;
}
/**
* @param urlArgObjects1 the urlArgObjects to set
*/
public void setUrlArgObjects(Map urlArgObjects1) {
this.urlArgObjects = urlArgObjects1;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy