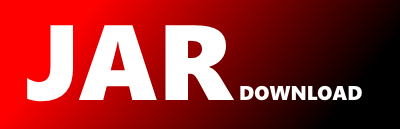
edu.internet2.middleware.grouper.ws.soap_v2_4.WsMessageAcknowledgeResults Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of grouper-ws Show documentation
Show all versions of grouper-ws Show documentation
Internet2 Groups Management WS Core
/*******************************************************************************
* Copyright 2016 Internet2
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
******************************************************************************/
package edu.internet2.middleware.grouper.ws.soap_v2_4;
import edu.internet2.middleware.grouper.misc.GrouperVersion;
import edu.internet2.middleware.grouper.ws.WsResultCode;
/**
* returned from the acknowledge web service
*
* @author vsachdeva
*
*/
public class WsMessageAcknowledgeResults {
/**
* result code of a request
*/
public static enum WsMessageAcknowledgeResultsCode implements WsResultCode {
/** messages acknowledged successfully (lite http status code 200) (success: T) */
SUCCESS(200),
/** problems with operation (lite http status code 500) (success: F) */
EXCEPTION(500),
/** invalid query (e.g. if everything blank) (lite http status code 400) (success: F) */
INVALID_QUERY(400);
/** get the name label for a certain version of client
* @param clientVersion
* @return name
*/
@Override
public String nameForVersion(GrouperVersion clientVersion) {
return this.name();
}
/** http status code for rest/lite e.g. 200 */
private int httpStatusCode;
/**
* status code for rest/lite e.g. 200
* @param statusCode
*/
private WsMessageAcknowledgeResultsCode(int statusCode) {
this.httpStatusCode = statusCode;
}
/**
* @see edu.internet2.middleware.grouper.ws.WsResultCode#getHttpStatusCode()
*/
@Override
public int getHttpStatusCode() {
return this.httpStatusCode;
}
/**
* if this is a successful result
*
* @return true if success
*/
@Override
public boolean isSuccess() {
return this == SUCCESS;
}
}
/**
* queue or topic to send to
*/
private String queueOrTopicName;
/**
* if there are multiple messaging systems, specify which one
*/
private String messageSystemName;
/**
* has 0 to many messages which were acknowledged
*/
private String[] messageIds;
/**
* metadata about the result
*/
private WsResultMeta resultMetadata = new WsResultMeta();
/**
* metadata about the response
*/
private WsResponseMeta responseMetadata = new WsResponseMeta();
/**
* @return the messageIds which were acknowledged
*/
public String[] getMessageIds() {
return this.messageIds;
}
/**
* @param messageIds1 the messages which were acknowledged
*/
public void setMessageIds(String[] messageIds1) {
this.messageIds = messageIds1;
}
/**
*
* @return queueOrTopicName
*/
public String getQueueOrTopicName() {
return this.queueOrTopicName;
}
/**
* @param queueOrTopicName1
*/
public void setQueueOrTopicName(String queueOrTopicName1) {
this.queueOrTopicName = queueOrTopicName1;
}
/**
* @return messageSystemName - if there are multiple messaging systems, specify which one
*/
public String getMessageSystemName() {
return this.messageSystemName;
}
/**
* @param messageSystemName1 - if there are multiple messaging systems, specify which one
*/
public void setMessageSystemName(String messageSystemName1) {
this.messageSystemName = messageSystemName1;
}
/**
* @return the resultMetadata
*/
public WsResultMeta getResultMetadata() {
return this.resultMetadata;
}
/**
* @see edu.internet2.middleware.grouper.ws.rest.WsResponseBean#getResponseMetadata()
* @return the response metadata
*/
public WsResponseMeta getResponseMetadata() {
return this.responseMetadata;
}
/**
* @param responseMetadata1 the responseMetadata to set
*/
public void setResponseMetadata(WsResponseMeta responseMetadata1) {
this.responseMetadata = responseMetadata1;
}
/**
* @param resultMetadata1 the resultMetadata to set
*/
public void setResultMetadata(WsResultMeta resultMetadata1) {
this.resultMetadata = resultMetadata1;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy