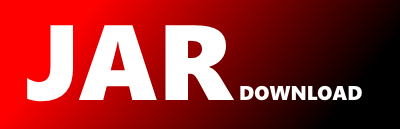
edu.internet2.middleware.grouper.dictionary.GrouperDictionary Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of grouper Show documentation
Show all versions of grouper Show documentation
Internet2 Groups Management Toolkit
/**
* @author mchyzer
* $Id$
*/
package edu.internet2.middleware.grouper.dictionary;
import java.sql.Timestamp;
import edu.internet2.middleware.grouper.tableIndex.TableIndex;
import edu.internet2.middleware.grouper.tableIndex.TableIndexType;
import edu.internet2.middleware.grouper.util.GrouperUtil;
import edu.internet2.middleware.grouperClient.jdbc.GcDbVersionable;
import edu.internet2.middleware.grouperClient.jdbc.GcPersist;
import edu.internet2.middleware.grouperClient.jdbc.GcPersistableClass;
import edu.internet2.middleware.grouperClient.jdbc.GcPersistableField;
import edu.internet2.middleware.grouperClient.jdbc.GcSqlAssignPrimaryKey;
import edu.internet2.middleware.grouperClient.util.GrouperClientUtils;
import org.apache.commons.lang3.builder.EqualsBuilder;
/**
* Dictionary holds strings in one place for other tables to refer to
*/
@GcPersistableClass(tableName="grouper_dictionary", defaultFieldPersist=GcPersist.doPersist)
public class GrouperDictionary implements GcSqlAssignPrimaryKey, GcDbVersionable {
/**
* version from db
*/
@GcPersistableField(persist = GcPersist.dontPersist)
private GrouperDictionary dbVersion;
/**
* take a snapshot of the data since this is what is in the db
*/
@Override
public void dbVersionReset() {
//lets get the state from the db so we know what has changed
this.dbVersion = this.clone();
}
/**
* if we need to update this object
* @return if needs to update this object
*/
@Override
public boolean dbVersionDifferent() {
return !this.equalsDeep(this.dbVersion);
}
/**
* db version
*/
@Override
public void dbVersionDelete() {
this.dbVersion = null;
}
public void storePrepare() {
if (this.createdOn == null) {
this.createdOn = new Timestamp(System.currentTimeMillis());
}
if (this.lastReferenced == null) {
this.lastReferenced = new Timestamp(System.currentTimeMillis());
}
if (this.preLoad == null) {
this.preLoad = "F";
}
}
/**
* deep clone the fields in this object
*/
@Override
public GrouperDictionary clone() {
GrouperDictionary grouperDictionary = new GrouperDictionary();
//dbVersion DONT CLONE
grouperDictionary.internalId = this.internalId;
grouperDictionary.lastReferenced = this.lastReferenced;
grouperDictionary.preLoad = this.preLoad;
grouperDictionary.theText = this.theText;
return grouperDictionary;
}
/**
*
*/
public boolean equalsDeep(Object obj) {
if (this==obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof GrouperDictionary)) {
return false;
}
GrouperDictionary other = (GrouperDictionary) obj;
return new EqualsBuilder()
//dbVersion DONT EQUALS
.append(this.createdOn, other.createdOn)
.append(this.internalId, other.internalId)
.append(this.lastReferenced, other.lastReferenced)
.append(this.preLoad, other.preLoad)
.append(this.theText, other.theText)
.isEquals();
}
//########## END GENERATED BY GcDbVersionableGenerate.java ###########
/**
* text for this dictionary item
*/
private String theText;
/**
* text for this dictionary item
* @return
*/
public String getTheText() {
return theText;
}
/**
* text for this dictionary item
* @param theText
*/
public void setTheText(String theText) {
this.theText = theText;
}
private Timestamp createdOn = null;
public Timestamp getCreatedOn() {
return createdOn;
}
public void setCreatedOn(Timestamp created) {
this.createdOn = created;
}
private Timestamp lastReferenced = null;
public Timestamp getLastReferenced() {
return lastReferenced;
}
public void setLastReferenced(Timestamp lastReferenced) {
this.lastReferenced = lastReferenced;
}
private String preLoad;
public boolean isPreLoad() {
return GrouperUtil.booleanValue(preLoad);
}
public void setOftenUsed(boolean oftenUsed) {
this.preLoad = oftenUsed ? "T" : "F";
}
public String getPreLoadDb() {
return this.preLoad;
}
public void setPreLoadDb(String oftenUsed) {
this.preLoad = oftenUsed;
}
/**
*
* @param args
*/
public static void main(String[] args) {
GrouperDictionary grouperDictionary = new GrouperDictionary();
grouperDictionary.setTheText("abc");
}
/**
*
*/
@Override
public String toString() {
return GrouperClientUtils.toStringReflection(this, null);
}
/**
* internal integer id
*/
@GcPersistableField(primaryKey=true, primaryKeyManuallyAssigned=true)
private long internalId = -1;
/**
* internal integer id
* @return
*/
public long getInternalId() {
return internalId;
}
/**
* internal integer id
* @param internalId
*/
public void setInternalId(long internalId) {
this.internalId = internalId;
}
/**
*
*/
public GrouperDictionary() {
}
/**
*
*/
@Override
public boolean gcSqlAssignNewPrimaryKeyForInsert() {
if (this.internalId != -1) {
return false;
}
this.internalId = TableIndex.reserveId(TableIndexType.dictionary);
return true;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy