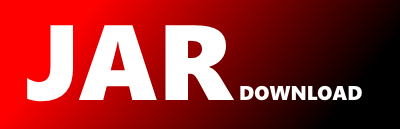
edu.internet2.middleware.grouper.app.gsh.template.GshTemplateConfiguration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of grouper Show documentation
Show all versions of grouper Show documentation
Internet2 Groups Management Toolkit
package edu.internet2.middleware.grouper.app.gsh.template;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.regex.Pattern;
import java.util.stream.Collectors;
import org.apache.commons.lang3.StringUtils;
import edu.internet2.middleware.grouper.Group;
import edu.internet2.middleware.grouper.GroupFinder;
import edu.internet2.middleware.grouper.GrouperSession;
import edu.internet2.middleware.grouper.Stem;
import edu.internet2.middleware.grouper.StemFinder;
import edu.internet2.middleware.grouper.Stem.Scope;
import edu.internet2.middleware.grouper.app.config.GrouperConfigurationModuleAttribute;
import edu.internet2.middleware.grouper.app.config.GrouperConfigurationModuleBase;
import edu.internet2.middleware.grouper.audit.AuditEntry;
import edu.internet2.middleware.grouper.audit.AuditTypeBuiltin;
import edu.internet2.middleware.grouper.cfg.dbConfig.ConfigFileName;
import edu.internet2.middleware.grouper.cfg.text.GrouperTextContainer;
import edu.internet2.middleware.grouper.hibernate.AuditControl;
import edu.internet2.middleware.grouper.hibernate.GrouperTransactionType;
import edu.internet2.middleware.grouper.hibernate.HibernateHandler;
import edu.internet2.middleware.grouper.hibernate.HibernateHandlerBean;
import edu.internet2.middleware.grouper.hibernate.HibernateSession;
import edu.internet2.middleware.grouper.internal.dao.GrouperDAOException;
import edu.internet2.middleware.grouper.util.GrouperUtil;
import edu.internet2.middleware.grouperClient.config.ConfigPropertiesCascadeBase;
public class GshTemplateConfiguration extends GrouperConfigurationModuleBase {
@Override
public ConfigFileName getConfigFileName() {
return ConfigFileName.GROUPER_PROPERTIES;
}
@Override
public String getConfigItemPrefix() {
if (StringUtils.isBlank(this.getConfigId())) {
throw new RuntimeException("Must have configId!");
}
return "grouperGshTemplate." + this.getConfigId() + ".";
}
@Override
public String getConfigIdRegex() {
return "^(grouperGshTemplate)\\.([^.]+)\\.(.*)$";
}
@Override
protected String getConfigurationTypePrefix() {
return "grouperGshTemplate";
}
@Override
public String getConfigIdThatIdentifiesThisConfig() {
return "testGshTemplate";
}
/**
* list of configured gsh template configs
* @return
*/
public static List retrieveAllGshTemplateConfigs() {
Set classNames = new HashSet();
classNames.add(GshTemplateConfiguration.class.getName());
return (List) (Object) retrieveAllConfigurations(classNames);
}
@Override
public void validatePreSave(boolean isInsert, List errorsToDisplay,
Map validationErrorsToDisplay) {
super.validatePreSave(isInsert, errorsToDisplay, validationErrorsToDisplay);
if (errorsToDisplay.size() > 0 || validationErrorsToDisplay.size() > 0) {
return;
}
Map attributes = this.retrieveAttributes();
GrouperConfigurationModuleAttribute showOnGroupsAttribute = attributes.get("showOnGroups");
String showOnGroupsValue = showOnGroupsAttribute.getValueOrExpressionEvaluation();
boolean showTemplateOnAllGroups = true;
Set groupsWhereTemplateIsAvailable = null;
Stem stemUnderWhichAnyGroupCanHaveTemplate = null;
if (GrouperUtil.booleanValue(showOnGroupsValue, false)) {
GrouperConfigurationModuleAttribute groupShowTypeAttribute = attributes.get("groupShowType");
String groupShowTypeValue = groupShowTypeAttribute.getValueOrExpressionEvaluation();
GshTemplateGroupShowType groupShowType = GshTemplateGroupShowType.valueOfIgnoreCase(groupShowTypeValue, true);
if (groupShowType == GshTemplateGroupShowType.certainGroups) {
showTemplateOnAllGroups = false;
GrouperConfigurationModuleAttribute groupUuidsToShowAttribute = attributes.get("groupUuidsToShow");
String groupUuidsToShow = groupUuidsToShowAttribute.getValueOrExpressionEvaluation();
String[] groupUuidsOrNamesToShow = GrouperUtil.splitTrim(groupUuidsToShow, ",");
Set groupUuidOrNamesToShowIn = GrouperUtil.toSet(groupUuidsOrNamesToShow);
groupsWhereTemplateIsAvailable = new GroupFinder()
.assignGroupNames(groupUuidOrNamesToShowIn)
.findGroups();
groupsWhereTemplateIsAvailable.addAll(new GroupFinder()
.assignGroupIds(groupUuidOrNamesToShowIn)
.findGroups());
if (groupsWhereTemplateIsAvailable.size() < groupUuidOrNamesToShowIn.size()) {
Set groupIdsThatWereFound = groupsWhereTemplateIsAvailable.stream().map(group -> group.getId()).collect(Collectors.toSet());
Set groupNamesThatWereFound = groupsWhereTemplateIsAvailable.stream().map(group -> group.getName()).collect(Collectors.toSet());
for (String groupUuidOrNameOnUi: groupUuidOrNamesToShowIn) {
if (!groupIdsThatWereFound.contains(groupUuidOrNameOnUi) && !groupNamesThatWereFound.contains(groupUuidOrNameOnUi)) {
String error = GrouperTextContainer.textOrNull("gshTemplateConfigSaveErrorGroupNotFound");
error = GrouperUtil.replace(error, "$$groupUUIDOrName$$", groupUuidOrNameOnUi);
validationErrorsToDisplay.put(groupUuidsToShowAttribute.getHtmlForElementIdHandle(), error);
}
}
}
} else if (groupShowType == GshTemplateGroupShowType.groupsInFolder) {
showTemplateOnAllGroups = false;
GrouperConfigurationModuleAttribute folderUuidForGroupsInFolderAttribute = attributes.get("folderUuidForGroupsInFolder");
String folderUuidForGroupsInFolder = folderUuidForGroupsInFolderAttribute.getValueOrExpressionEvaluation();
stemUnderWhichAnyGroupCanHaveTemplate = StemFinder.findByUuid(GrouperSession.staticGrouperSession(), folderUuidForGroupsInFolder, false);
if (stemUnderWhichAnyGroupCanHaveTemplate == null) {
stemUnderWhichAnyGroupCanHaveTemplate = StemFinder.findByName(GrouperSession.staticGrouperSession(), folderUuidForGroupsInFolder, false);
}
if (stemUnderWhichAnyGroupCanHaveTemplate == null) {
String error = GrouperTextContainer.textOrNull("gshTemplateConfigSaveErrorFolderNotFound");
error = GrouperUtil.replace(error, "$$folderUUIDOrName$$", folderUuidForGroupsInFolder);
validationErrorsToDisplay.put(folderUuidForGroupsInFolderAttribute.getHtmlForElementIdHandle(), error);
}
}
}
GrouperConfigurationModuleAttribute showOnFoldersAttribute = attributes.get("showOnFolders");
String showOnFoldersValue = showOnFoldersAttribute.getValueOrExpressionEvaluation();
if (GrouperUtil.booleanValue(showOnFoldersValue, false)) {
GrouperConfigurationModuleAttribute folderShowTypeAttribute = attributes.get("folderShowType");
String folderShowTypeValue = folderShowTypeAttribute.getValueOrExpressionEvaluation();
GshTemplateFolderShowType folderShowType = GshTemplateFolderShowType.valueOfIgnoreCase(folderShowTypeValue, true);
if (folderShowType == GshTemplateFolderShowType.certainFolders) {
showTemplateOnAllGroups = false;
GrouperConfigurationModuleAttribute folderUuidsToShowAttribute = attributes.get("folderUuidToShow");
String folderUuidsToShow = folderUuidsToShowAttribute.getValueOrExpressionEvaluation();
String[] folderUuidsOrNamesToShow = GrouperUtil.splitTrim(folderUuidsToShow, ",");
Set folderUuidOrNamesToShowIn = GrouperUtil.toSet(folderUuidsOrNamesToShow);
Set stemsToShowTemplateIn = new StemFinder()
.assignStemNames(folderUuidOrNamesToShowIn)
.findStems();
stemsToShowTemplateIn.addAll(new StemFinder()
.assignStemIds(folderUuidOrNamesToShowIn)
.findStems());
if (stemsToShowTemplateIn.size() < folderUuidOrNamesToShowIn.size()) {
Set stemIdsThatWereFound = stemsToShowTemplateIn.stream().map(stem -> stem.getId()).collect(Collectors.toSet());
Set stemNamesThatWereFound = stemsToShowTemplateIn.stream().map(stem -> stem.getName()).collect(Collectors.toSet());
for (String folderUuidOrNameOnUi: folderUuidOrNamesToShowIn) {
if (!stemIdsThatWereFound.contains(folderUuidOrNameOnUi) && !stemNamesThatWereFound.contains(folderUuidOrNameOnUi)) {
String error = GrouperTextContainer.textOrNull("gshTemplateConfigSaveErrorFolderNotFound");
error = GrouperUtil.replace(error, "$$folderUUIDOrName$$", folderUuidOrNameOnUi);
validationErrorsToDisplay.put(folderUuidsToShowAttribute.getHtmlForElementIdHandle(), error);
}
}
}
}
}
GrouperConfigurationModuleAttribute runButtonGroupOrFolderAttribute = attributes.get("runButtonGroupOrFolder");
String runButtonGroupOrFolderAttributeValue = runButtonGroupOrFolderAttribute.getValueOrExpressionEvaluation();
if (StringUtils.equals("group", runButtonGroupOrFolderAttributeValue)) {
GrouperConfigurationModuleAttribute defaultRunButtonGroupUuidOrNameAttribute = attributes.get("defaultRunButtonGroupUuidOrName");
String groupUuidOrName = defaultRunButtonGroupUuidOrNameAttribute.getValueOrExpressionEvaluation();
Group group = GroupFinder.findByUuid(groupUuidOrName, false);
if (group == null) {
group = GroupFinder.findByName(groupUuidOrName, false);
}
if (group == null) {
String error = GrouperTextContainer.textOrNull("gshTemplateConfigSaveErrorGroupNotFound");
error = GrouperUtil.replace(error, "$$groupUUIDOrName$$", groupUuidOrName);
validationErrorsToDisplay.put(defaultRunButtonGroupUuidOrNameAttribute.getHtmlForElementIdHandle(), error);
} else {
// we still need to check if the default run group is one of the groups where the template can show
if (!showTemplateOnAllGroups) {
if (groupsWhereTemplateIsAvailable != null && !groupsWhereTemplateIsAvailable.contains(group)) {
String error = GrouperTextContainer.textOrNull("gshTemplateConfigSaveErrorDefaultRunGroupNotInGroupsToShowList");
error = GrouperUtil.replace(error, "$$groupUUIDOrName$$", groupUuidOrName);
validationErrorsToDisplay.put(defaultRunButtonGroupUuidOrNameAttribute.getHtmlForElementIdHandle(), error);
} else if (stemUnderWhichAnyGroupCanHaveTemplate != null) {
GrouperConfigurationModuleAttribute groupShowOnDescendantsAttribute = attributes.get("groupShowOnDescendants");
String groupShowOnDescendants = groupShowOnDescendantsAttribute.getValueOrExpressionEvaluation();
GshTemplateGroupShowOnDescendants showOnDescendants = GshTemplateGroupShowOnDescendants.valueOfIgnoreCase(groupShowOnDescendants, true);
if (GshTemplateGroupShowOnDescendants.descendants == showOnDescendants && !stemUnderWhichAnyGroupCanHaveTemplate.isChildGroup(group)) {
String error = GrouperTextContainer.textOrNull("gshTemplateConfigSaveErrorDefaultRunGroupNotUnderFolderToShow");
error = GrouperUtil.replace(error, "$$groupUUIDOrName$$", groupUuidOrName);
validationErrorsToDisplay.put(defaultRunButtonGroupUuidOrNameAttribute.getHtmlForElementIdHandle(), error);
} else if (GshTemplateGroupShowOnDescendants.oneChildLevel == showOnDescendants && !stemUnderWhichAnyGroupCanHaveTemplate.getChildGroups(Scope.ONE).contains(group)) {
String error = GrouperTextContainer.textOrNull("gshTemplateConfigSaveErrorDefaultRunGroupNotUnderFolderToShow");
error = GrouperUtil.replace(error, "$$groupUUIDOrName$$", groupUuidOrName);
error = GrouperUtil.replace(error, "$$folderUUIDOrName$$", stemUnderWhichAnyGroupCanHaveTemplate.getName());
validationErrorsToDisplay.put(defaultRunButtonGroupUuidOrNameAttribute.getHtmlForElementIdHandle(), error);
}
}
}
}
} else if (StringUtils.equals("folder", runButtonGroupOrFolderAttributeValue)) {
GrouperConfigurationModuleAttribute defaultRunButtonFolderUuidOrNameAttribute = attributes.get("defaultRunButtonFolderUuidOrName");
String folderUuidOrName = defaultRunButtonFolderUuidOrNameAttribute.getValueOrExpressionEvaluation();
Stem stem = StemFinder.findByUuid(GrouperSession.staticGrouperSession(), folderUuidOrName, false);
if (stem == null) {
stem = StemFinder.findByName(GrouperSession.staticGrouperSession(), folderUuidOrName, false);
}
if (stem == null) {
String error = GrouperTextContainer.textOrNull("gshTemplateConfigSaveErrorFolderNotFound");
error = GrouperUtil.replace(error, "$$folderUUIDOrName$$", folderUuidOrName);
validationErrorsToDisplay.put(defaultRunButtonFolderUuidOrNameAttribute.getHtmlForElementIdHandle(), error);
return;
}
// we still need to check if the default run folder is one of the folders where the template can show
if (!canDefaultRunFolderShowTemplate(stem)) {
String error = GrouperTextContainer.textOrNull("gshTemplateConfigSaveErrorDefaultRunFolderNotInFoldersToShowList");
error = GrouperUtil.replace(error, "$$folderUUIDOrName$$", folderUuidOrName);
validationErrorsToDisplay.put(defaultRunButtonFolderUuidOrNameAttribute.getHtmlForElementIdHandle(), error);
return;
}
}
GrouperConfigurationModuleAttribute numberOfInputsAttribute = attributes.get("numberOfInputs");
String valueOrExpressionEvaluation = numberOfInputsAttribute.getValueOrExpressionEvaluation();
int numberOfInputs = GrouperUtil.intValue(valueOrExpressionEvaluation, 0);
for (int i=0; i" + e.getMessage() + "
";
}
}
if (invalidRegex) {
validationErrorsToDisplay.put(validationRegexAttribute.getHtmlForElementIdHandle(), error);
return;
}
}
}
}
private boolean canDefaultRunFolderShowTemplate(Stem defaultRunFolder) {
Map© 2015 - 2024 Weber Informatics LLC | Privacy Policy