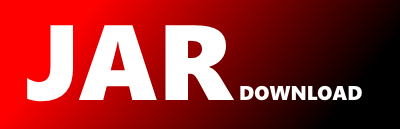
edu.internet2.middleware.grouper.app.provisioning.GrouperProvisioningSettings Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of grouper Show documentation
Show all versions of grouper Show documentation
Internet2 Groups Management Toolkit
package edu.internet2.middleware.grouper.app.provisioning;
import java.util.HashMap;
import java.util.Map;
import java.util.Map.Entry;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.databind.ObjectMapper;
import edu.internet2.middleware.grouper.app.loader.GrouperLoaderConfig;
import edu.internet2.middleware.grouper.cfg.GrouperConfig;
import edu.internet2.middleware.grouper.util.GrouperUtil;
import edu.internet2.middleware.grouperClient.util.ExpirableCache;
public class GrouperProvisioningSettings {
private static final Pattern grouperProvisioningTargetKey = Pattern.compile("^provisioner\\.([\\w-]+)\\.class$");
private static ExpirableCache> __targetsCacheInternal;
private static synchronized ExpirableCache> targetsCache() {
if (__targetsCacheInternal == null) {
__targetsCacheInternal = new ExpirableCache>(5);
__targetsCacheInternal.registerDatabaseClearableCache("grouperProvisioningTargetsCache");
}
return __targetsCacheInternal;
}
public static void clearTargetsCache() {
targetsCache().notifyDatabaseOfChanges();
targetsCache().clear();
}
private static Map populateTargets() {
Map result = new HashMap();
Map propertiesMap = GrouperLoaderConfig.retrieveConfig().propertiesMap(grouperProvisioningTargetKey);
for (Entry entry: propertiesMap.entrySet()) {
String property = entry.getKey();
Matcher matcher = grouperProvisioningTargetKey.matcher(property);
if (matcher.matches()) {
// name is the part of the property key
String name = matcher.group(1);
String groupAllowedToAssign = GrouperLoaderConfig.retrieveConfig().propertyValueString("provisioner."+name+".groupAllowedToAssign", null);
String groupAllowedToView = GrouperLoaderConfig.retrieveConfig().propertyValueString("provisioner."+name+".groupAllowedToView", null);
boolean allowAssignmentsOnlyOnOneStem = GrouperLoaderConfig.retrieveConfig().propertyValueBoolean("provisioner."+name+".allowAssignmentsOnlyOnOneStem", false);
boolean readOnly = GrouperLoaderConfig.retrieveConfig().propertyValueBoolean("provisioner."+name+".readOnly", false);
GrouperProvisioningTarget target = new GrouperProvisioningTarget(name, name);
target.setGroupAllowedToAssign(groupAllowedToAssign);
target.setGroupAllowedToView(groupAllowedToView);
target.setAllowAssignmentsOnlyOnOneStem(allowAssignmentsOnlyOnOneStem);
target.setReadOnly(readOnly);
result.put(name, target);
}
}
return result;
}
/**
* if provisioning in ui is enabled
* @return if provisioning in ui is enabled
*/
public static boolean provisioningInUiEnabled() {
return GrouperConfig.retrieveConfig().propertyValueBoolean("provisioningInUi.enable", false);
}
/**
*
* @return the stem name with no last colon
*/
public static String provisioningConfigStemName() {
return GrouperUtil.stripSuffix(GrouperConfig.retrieveConfig().propertyValueString("provisioningInUi.systemFolder",
GrouperConfig.retrieveConfig().propertyValueString("grouper.rootStemForBuiltinObjects") + ":provisioning"), ":");
}
/**
* all the provisioning targets
* @return targets
*/
public static Map getTargets(boolean useCache) {
Map result = targetsCache().get(Boolean.TRUE);
if (result == null || !useCache) {
result = populateTargets();
targetsCache().put(Boolean.TRUE, result);
}
return result;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy