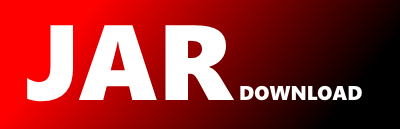
edu.internet2.middleware.grouper.dataField.GrouperDataFieldConfiguration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of grouper Show documentation
Show all versions of grouper Show documentation
Internet2 Groups Management Toolkit
package edu.internet2.middleware.grouper.dataField;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import org.apache.commons.lang3.StringUtils;
import edu.internet2.middleware.grouper.app.config.GrouperConfigurationModuleAttribute;
import edu.internet2.middleware.grouper.app.config.GrouperConfigurationModuleBase;
import edu.internet2.middleware.grouper.cfg.GrouperConfig;
import edu.internet2.middleware.grouper.cfg.dbConfig.ConfigFileName;
import edu.internet2.middleware.grouper.cfg.text.GrouperTextContainer;
import edu.internet2.middleware.grouper.hibernate.GrouperTransaction;
import edu.internet2.middleware.grouper.hibernate.GrouperTransactionHandler;
import edu.internet2.middleware.grouper.internal.dao.GrouperDAOException;
import edu.internet2.middleware.grouper.util.GrouperUtil;
public class GrouperDataFieldConfiguration extends GrouperConfigurationModuleBase {
@Override
public ConfigFileName getConfigFileName() {
return ConfigFileName.GROUPER_PROPERTIES;
}
@Override
public String getConfigItemPrefix() {
if (StringUtils.isBlank(this.getConfigId())) {
throw new RuntimeException("Must have configId!");
}
return "grouperDataField." + this.getConfigId() + ".";
}
@Override
public String getConfigIdRegex() {
return "^(grouperDataField)\\.([^.]+)\\.(.*)$";
}
@Override
protected String getConfigurationTypePrefix() {
return "grouperDataField";
}
@Override
public String getConfigIdThatIdentifiesThisConfig() {
return "dataFieldConfigId";
}
/**
* list of configured data field configs
* @return
*/
public static List retrieveAllDataFieldConfigurations() {
Set classNames = new HashSet();
classNames.add(GrouperDataFieldConfiguration.class.getName());
return (List) (Object) retrieveAllConfigurations(classNames);
}
@Override
public void validatePreSave(boolean isInsert, List errorsToDisplay, Map validationErrorsToDisplay) {
super.validatePreSave(isInsert, errorsToDisplay, validationErrorsToDisplay);
if (errorsToDisplay.size() > 0 || validationErrorsToDisplay.size() > 0) {
return;
}
GrouperConfigurationModuleAttribute fieldAliases = this.retrieveAttributes().get("fieldAliases");
if (fieldAliases != null && StringUtils.isNotBlank(fieldAliases.getValueOrExpressionEvaluation())) {
String fieldAliasesCommaSeparated = fieldAliases.getValueOrExpressionEvaluation();
Set fieldAliasesSet = GrouperUtil.splitTrimToSet(fieldAliasesCommaSeparated, ",");
GrouperDataEngine grouperDataEngine = new GrouperDataEngine();
GrouperConfig grouperConfig = GrouperConfig.retrieveConfig();
grouperDataEngine.loadFieldsAndRows(grouperConfig);
Map fieldConfigByAlias = grouperDataEngine.getFieldConfigByAlias();
for (String fieldAliasBeingAdded: fieldAliasesSet) {
if (fieldConfigByAlias.containsKey(fieldAliasBeingAdded.toLowerCase()) &&
!StringUtils.equals(this.getConfigId(), fieldConfigByAlias.get(fieldAliasBeingAdded.toLowerCase()).getConfigId())) {
String errorMessage = GrouperTextContainer.retrieveFromRequest().getText().get("dataFieldRowAliasAlreadyUsedError");
errorMessage = errorMessage.replace("##dataFieldAlias##", fieldAliasBeingAdded);
errorsToDisplay.add(errorMessage);
}
}
Map rowConfigByAlias = grouperDataEngine.getRowConfigByAlias();
for (String fieldAliasBeingAdded: fieldAliasesSet) {
if (rowConfigByAlias.containsKey(fieldAliasBeingAdded.toLowerCase()) &&
!StringUtils.equals(this.getConfigId(), rowConfigByAlias.get(fieldAliasBeingAdded.toLowerCase()).getConfigId())) {
String errorMessage = GrouperTextContainer.retrieveFromRequest().getText().get("dataFieldRowAliasAlreadyUsedError");
errorMessage = errorMessage.replace("##dataFieldAlias##", fieldAliasBeingAdded);
errorsToDisplay.add(errorMessage);
}
}
}
}
@Override
public void insertConfig(boolean fromUi, StringBuilder message,
List errorsToDisplay, Map validationErrorsToDisplay,
List actionsPerformed) {
GrouperTransaction.callbackGrouperTransaction(new GrouperTransactionHandler() {
@Override
public Object callback(GrouperTransaction grouperTransaction)
throws GrouperDAOException {
GrouperDataFieldConfiguration.super.insertConfig(fromUi, message, errorsToDisplay, validationErrorsToDisplay,
actionsPerformed);
if (errorsToDisplay.size() == 0 && validationErrorsToDisplay.size() == 0) {
GrouperConfig grouperConfig = GrouperConfig.retrieveConfig();
GrouperDataEngine.syncDataFields(grouperConfig);
GrouperDataEngine.syncDataAliases(grouperConfig);
}
return null;
}
});
}
@Override
public void deleteConfig(boolean fromUi) {
GrouperTransaction.callbackGrouperTransaction(new GrouperTransactionHandler() {
@Override
public Object callback(GrouperTransaction grouperTransaction)
throws GrouperDAOException {
GrouperDataFieldConfiguration.super.deleteConfig(fromUi);
GrouperConfig grouperConfig = GrouperConfig.retrieveConfig();
GrouperDataEngine.syncDataAliases(grouperConfig);
GrouperDataEngine.syncDataFields(grouperConfig);
return null;
}
});
}
@Override
public void editConfig(boolean fromUi, StringBuilder message, List errorsToDisplay,
Map validationErrorsToDisplay, List actionsPerformed) {
GrouperTransaction.callbackGrouperTransaction(new GrouperTransactionHandler() {
@Override
public Object callback(GrouperTransaction grouperTransaction)
throws GrouperDAOException {
GrouperDataFieldConfiguration.super.editConfig(fromUi, message, errorsToDisplay, validationErrorsToDisplay,
actionsPerformed);
if (errorsToDisplay.size() == 0 && validationErrorsToDisplay.size() == 0) {
GrouperConfig grouperConfig = GrouperConfig.retrieveConfig();
GrouperDataEngine.syncDataFields(grouperConfig);
GrouperDataEngine.syncDataAliases(grouperConfig);
}
return null;
}
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy