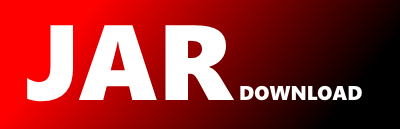
edu.isi.nlp.ImmutableAnnotationWrapperModule Maven / Gradle / Ivy
package edu.isi.nlp;
import com.google.common.base.MoreObjects;
import com.google.common.base.Preconditions;
import com.google.common.collect.ImmutableMap;
import com.google.common.collect.ImmutableSet;
import com.google.common.collect.Lists;
import com.google.inject.Key;
import com.google.inject.TypeLiteral;
import edu.isi.nlp.parameters.Parameters;
import java.lang.annotation.Annotation;
import java.util.List;
import java.util.Map;
import javax.annotation.Generated;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
/**
* Immutable implementation of {@link AnnotationWrapperModule}.
*
* Use the builder to create immutable instances:
* {@code new AnnotationWrapperModule.Builder()}.
*/
@SuppressWarnings({"unchecked", "all"})
@ParametersAreNonnullByDefault
@Generated({"Immutables.generator", "AnnotationWrapperModule"})
@Immutable
final class ImmutableAnnotationWrapperModule extends AnnotationWrapperModule {
private final String wrappedModuleParam;
private final Class extends Annotation> wrappingAnnotation;
private final ImmutableSet> simpleTypesToWrap;
private final ImmutableSet> setsToWrap;
private final ImmutableMap, TypeLiteral>> mapsToWrap;
private final Parameters params;
private ImmutableAnnotationWrapperModule(
String wrappedModuleParam,
Class extends Annotation> wrappingAnnotation,
ImmutableSet> simpleTypesToWrap,
ImmutableSet> setsToWrap,
ImmutableMap, TypeLiteral>> mapsToWrap,
Parameters params) {
this.wrappedModuleParam = wrappedModuleParam;
this.wrappingAnnotation = wrappingAnnotation;
this.simpleTypesToWrap = simpleTypesToWrap;
this.setsToWrap = setsToWrap;
this.mapsToWrap = mapsToWrap;
this.params = params;
}
/**
* @return The value of the {@code wrappedModuleParam} attribute
*/
@Override
String wrappedModuleParam() {
return wrappedModuleParam;
}
/**
* @return The value of the {@code wrappingAnnotation} attribute
*/
@Override
Class extends Annotation> wrappingAnnotation() {
return wrappingAnnotation;
}
/**
* @return The value of the {@code simpleTypesToWrap} attribute
*/
@Override
ImmutableSet> simpleTypesToWrap() {
return simpleTypesToWrap;
}
/**
* @return The value of the {@code setsToWrap} attribute
*/
@Override
ImmutableSet> setsToWrap() {
return setsToWrap;
}
/**
* @return The value of the {@code mapsToWrap} attribute
*/
@Override
ImmutableMap, TypeLiteral>> mapsToWrap() {
return mapsToWrap;
}
/**
* @return The value of the {@code params} attribute
*/
@Override
Parameters params() {
return params;
}
/**
* Copy the current immutable object by setting a value for the {@link AnnotationWrapperModule#wrappedModuleParam() wrappedModuleParam} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for wrappedModuleParam
* @return A modified copy of the {@code this} object
*/
public final ImmutableAnnotationWrapperModule withWrappedModuleParam(String value) {
if (this.wrappedModuleParam.equals(value)) return this;
String newValue = Preconditions.checkNotNull(value, "wrappedModuleParam");
return validate(new ImmutableAnnotationWrapperModule(
newValue,
this.wrappingAnnotation,
this.simpleTypesToWrap,
this.setsToWrap,
this.mapsToWrap,
this.params));
}
/**
* Copy the current immutable object by setting a value for the {@link AnnotationWrapperModule#wrappingAnnotation() wrappingAnnotation} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for wrappingAnnotation
* @return A modified copy of the {@code this} object
*/
public final ImmutableAnnotationWrapperModule withWrappingAnnotation(Class extends Annotation> value) {
if (this.wrappingAnnotation == value) return this;
Class extends Annotation> newValue = Preconditions.checkNotNull(value, "wrappingAnnotation");
return validate(new ImmutableAnnotationWrapperModule(
this.wrappedModuleParam,
newValue,
this.simpleTypesToWrap,
this.setsToWrap,
this.mapsToWrap,
this.params));
}
/**
* Copy the current immutable object with elements that replace the content of {@link AnnotationWrapperModule#simpleTypesToWrap() simpleTypesToWrap}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
@SafeVarargs
public final ImmutableAnnotationWrapperModule withSimpleTypesToWrap(Key>... elements) {
ImmutableSet> newValue = ImmutableSet.copyOf(elements);
return validate(new ImmutableAnnotationWrapperModule(
this.wrappedModuleParam,
this.wrappingAnnotation,
newValue,
this.setsToWrap,
this.mapsToWrap,
this.params));
}
/**
* Copy the current immutable object with elements that replace the content of {@link AnnotationWrapperModule#simpleTypesToWrap() simpleTypesToWrap}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of simpleTypesToWrap elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableAnnotationWrapperModule withSimpleTypesToWrap(Iterable extends Key>> elements) {
if (this.simpleTypesToWrap == elements) return this;
ImmutableSet> newValue = ImmutableSet.copyOf(elements);
return validate(new ImmutableAnnotationWrapperModule(
this.wrappedModuleParam,
this.wrappingAnnotation,
newValue,
this.setsToWrap,
this.mapsToWrap,
this.params));
}
/**
* Copy the current immutable object with elements that replace the content of {@link AnnotationWrapperModule#setsToWrap() setsToWrap}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
@SafeVarargs
public final ImmutableAnnotationWrapperModule withSetsToWrap(TypeLiteral>... elements) {
ImmutableSet> newValue = ImmutableSet.copyOf(elements);
return validate(new ImmutableAnnotationWrapperModule(
this.wrappedModuleParam,
this.wrappingAnnotation,
this.simpleTypesToWrap,
newValue,
this.mapsToWrap,
this.params));
}
/**
* Copy the current immutable object with elements that replace the content of {@link AnnotationWrapperModule#setsToWrap() setsToWrap}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of setsToWrap elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableAnnotationWrapperModule withSetsToWrap(Iterable extends TypeLiteral>> elements) {
if (this.setsToWrap == elements) return this;
ImmutableSet> newValue = ImmutableSet.copyOf(elements);
return validate(new ImmutableAnnotationWrapperModule(
this.wrappedModuleParam,
this.wrappingAnnotation,
this.simpleTypesToWrap,
newValue,
this.mapsToWrap,
this.params));
}
/**
* Copy the current immutable object by replacing the {@link AnnotationWrapperModule#mapsToWrap() mapsToWrap} map with the specified map.
* Nulls are not permitted as keys or values.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param entries The entries to be added to the mapsToWrap map
* @return A modified copy of {@code this} object
*/
public final ImmutableAnnotationWrapperModule withMapsToWrap(Map extends TypeLiteral>, ? extends TypeLiteral>> entries) {
if (this.mapsToWrap == entries) return this;
ImmutableMap, TypeLiteral>> newValue = ImmutableMap.copyOf(entries);
return validate(new ImmutableAnnotationWrapperModule(
this.wrappedModuleParam,
this.wrappingAnnotation,
this.simpleTypesToWrap,
this.setsToWrap,
newValue,
this.params));
}
/**
* Copy the current immutable object by setting a value for the {@link AnnotationWrapperModule#params() params} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for params
* @return A modified copy of the {@code this} object
*/
public final ImmutableAnnotationWrapperModule withParams(Parameters value) {
if (this.params == value) return this;
Parameters newValue = Preconditions.checkNotNull(value, "params");
return validate(new ImmutableAnnotationWrapperModule(
this.wrappedModuleParam,
this.wrappingAnnotation,
this.simpleTypesToWrap,
this.setsToWrap,
this.mapsToWrap,
newValue));
}
/**
* This instance is equal to all instances of {@code ImmutableAnnotationWrapperModule} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableAnnotationWrapperModule
&& equalTo((ImmutableAnnotationWrapperModule) another);
}
private boolean equalTo(ImmutableAnnotationWrapperModule another) {
return wrappedModuleParam.equals(another.wrappedModuleParam)
&& wrappingAnnotation.equals(another.wrappingAnnotation)
&& simpleTypesToWrap.equals(another.simpleTypesToWrap)
&& setsToWrap.equals(another.setsToWrap)
&& mapsToWrap.equals(another.mapsToWrap)
&& params.equals(another.params);
}
/**
* Computes a hash code from attributes: {@code wrappedModuleParam}, {@code wrappingAnnotation}, {@code simpleTypesToWrap}, {@code setsToWrap}, {@code mapsToWrap}, {@code params}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 31;
h = h * 17 + wrappedModuleParam.hashCode();
h = h * 17 + wrappingAnnotation.hashCode();
h = h * 17 + simpleTypesToWrap.hashCode();
h = h * 17 + setsToWrap.hashCode();
h = h * 17 + mapsToWrap.hashCode();
h = h * 17 + params.hashCode();
return h;
}
/**
* Prints the immutable value {@code AnnotationWrapperModule} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return MoreObjects.toStringHelper("AnnotationWrapperModule")
.omitNullValues()
.add("wrappedModuleParam", wrappedModuleParam)
.add("wrappingAnnotation", wrappingAnnotation)
.add("simpleTypesToWrap", simpleTypesToWrap)
.add("setsToWrap", setsToWrap)
.add("mapsToWrap", mapsToWrap)
.add("params", params)
.toString();
}
private static ImmutableAnnotationWrapperModule validate(ImmutableAnnotationWrapperModule instance) {
instance.check();
return instance;
}
/**
* Creates an immutable copy of a {@link AnnotationWrapperModule} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable AnnotationWrapperModule instance
*/
public static AnnotationWrapperModule copyOf(AnnotationWrapperModule instance) {
if (instance instanceof ImmutableAnnotationWrapperModule) {
return (ImmutableAnnotationWrapperModule) instance;
}
return new AnnotationWrapperModule.Builder()
.from(instance)
.build();
}
/**
* Builds instances of type {@link AnnotationWrapperModule AnnotationWrapperModule}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@NotThreadSafe
static class Builder {
private static final long INIT_BIT_WRAPPED_MODULE_PARAM = 0x1L;
private static final long INIT_BIT_WRAPPING_ANNOTATION = 0x2L;
private static final long INIT_BIT_PARAMS = 0x4L;
private long initBits = 0x7L;
private @Nullable String wrappedModuleParam;
private @Nullable Class extends Annotation> wrappingAnnotation;
private ImmutableSet.Builder> simpleTypesToWrap = ImmutableSet.builder();
private ImmutableSet.Builder> setsToWrap = ImmutableSet.builder();
private ImmutableMap.Builder, TypeLiteral>> mapsToWrap = ImmutableMap.builder();
private @Nullable Parameters params;
/**
* Creates a builder for {@link AnnotationWrapperModule AnnotationWrapperModule} instances.
*/
Builder() {
if (!(this instanceof AnnotationWrapperModule.Builder)) {
throw new UnsupportedOperationException("Use: new AnnotationWrapperModule.Builder()");
}
}
/**
* Fill a builder with attribute values from the provided {@code AnnotationWrapperModule} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* Collection elements and entries will be added, not replaced.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final AnnotationWrapperModule.Builder from(AnnotationWrapperModule instance) {
Preconditions.checkNotNull(instance, "instance");
wrappedModuleParam(instance.wrappedModuleParam());
wrappingAnnotation(instance.wrappingAnnotation());
addAllSimpleTypesToWrap(instance.simpleTypesToWrap());
addAllSetsToWrap(instance.setsToWrap());
putAllMapsToWrap(instance.mapsToWrap());
params(instance.params());
return (AnnotationWrapperModule.Builder) this;
}
/**
* Initializes the value for the {@link AnnotationWrapperModule#wrappedModuleParam() wrappedModuleParam} attribute.
* @param wrappedModuleParam The value for wrappedModuleParam
* @return {@code this} builder for use in a chained invocation
*/
public final AnnotationWrapperModule.Builder wrappedModuleParam(String wrappedModuleParam) {
this.wrappedModuleParam = Preconditions.checkNotNull(wrappedModuleParam, "wrappedModuleParam");
initBits &= ~INIT_BIT_WRAPPED_MODULE_PARAM;
return (AnnotationWrapperModule.Builder) this;
}
/**
* Initializes the value for the {@link AnnotationWrapperModule#wrappingAnnotation() wrappingAnnotation} attribute.
* @param wrappingAnnotation The value for wrappingAnnotation
* @return {@code this} builder for use in a chained invocation
*/
public final AnnotationWrapperModule.Builder wrappingAnnotation(Class extends Annotation> wrappingAnnotation) {
this.wrappingAnnotation = Preconditions.checkNotNull(wrappingAnnotation, "wrappingAnnotation");
initBits &= ~INIT_BIT_WRAPPING_ANNOTATION;
return (AnnotationWrapperModule.Builder) this;
}
/**
* Adds one element to {@link AnnotationWrapperModule#simpleTypesToWrap() simpleTypesToWrap} set.
* @param element A simpleTypesToWrap element
* @return {@code this} builder for use in a chained invocation
*/
public final AnnotationWrapperModule.Builder addSimpleTypesToWrap(Key> element) {
this.simpleTypesToWrap.add(element);
return (AnnotationWrapperModule.Builder) this;
}
/**
* Adds elements to {@link AnnotationWrapperModule#simpleTypesToWrap() simpleTypesToWrap} set.
* @param elements An array of simpleTypesToWrap elements
* @return {@code this} builder for use in a chained invocation
*/
@SafeVarargs
public final AnnotationWrapperModule.Builder addSimpleTypesToWrap(Key>... elements) {
this.simpleTypesToWrap.add(elements);
return (AnnotationWrapperModule.Builder) this;
}
/**
* Sets or replaces all elements for {@link AnnotationWrapperModule#simpleTypesToWrap() simpleTypesToWrap} set.
* @param elements An iterable of simpleTypesToWrap elements
* @return {@code this} builder for use in a chained invocation
*/
public final AnnotationWrapperModule.Builder simpleTypesToWrap(Iterable extends Key>> elements) {
this.simpleTypesToWrap = ImmutableSet.builder();
return addAllSimpleTypesToWrap(elements);
}
/**
* Adds elements to {@link AnnotationWrapperModule#simpleTypesToWrap() simpleTypesToWrap} set.
* @param elements An iterable of simpleTypesToWrap elements
* @return {@code this} builder for use in a chained invocation
*/
public final AnnotationWrapperModule.Builder addAllSimpleTypesToWrap(Iterable extends Key>> elements) {
this.simpleTypesToWrap.addAll(elements);
return (AnnotationWrapperModule.Builder) this;
}
/**
* Adds one element to {@link AnnotationWrapperModule#setsToWrap() setsToWrap} set.
* @param element A setsToWrap element
* @return {@code this} builder for use in a chained invocation
*/
public final AnnotationWrapperModule.Builder addSetsToWrap(TypeLiteral> element) {
this.setsToWrap.add(element);
return (AnnotationWrapperModule.Builder) this;
}
/**
* Adds elements to {@link AnnotationWrapperModule#setsToWrap() setsToWrap} set.
* @param elements An array of setsToWrap elements
* @return {@code this} builder for use in a chained invocation
*/
@SafeVarargs
public final AnnotationWrapperModule.Builder addSetsToWrap(TypeLiteral>... elements) {
this.setsToWrap.add(elements);
return (AnnotationWrapperModule.Builder) this;
}
/**
* Sets or replaces all elements for {@link AnnotationWrapperModule#setsToWrap() setsToWrap} set.
* @param elements An iterable of setsToWrap elements
* @return {@code this} builder for use in a chained invocation
*/
public final AnnotationWrapperModule.Builder setsToWrap(Iterable extends TypeLiteral>> elements) {
this.setsToWrap = ImmutableSet.builder();
return addAllSetsToWrap(elements);
}
/**
* Adds elements to {@link AnnotationWrapperModule#setsToWrap() setsToWrap} set.
* @param elements An iterable of setsToWrap elements
* @return {@code this} builder for use in a chained invocation
*/
public final AnnotationWrapperModule.Builder addAllSetsToWrap(Iterable extends TypeLiteral>> elements) {
this.setsToWrap.addAll(elements);
return (AnnotationWrapperModule.Builder) this;
}
/**
* Put one entry to the {@link AnnotationWrapperModule#mapsToWrap() mapsToWrap} map.
* @param key The key in the mapsToWrap map
* @param value The associated value in the mapsToWrap map
* @return {@code this} builder for use in a chained invocation
*/
public final AnnotationWrapperModule.Builder putMapsToWrap(TypeLiteral> key, TypeLiteral> value) {
this.mapsToWrap.put(key, value);
return (AnnotationWrapperModule.Builder) this;
}
/**
* Put one entry to the {@link AnnotationWrapperModule#mapsToWrap() mapsToWrap} map. Nulls are not permitted
* @param entry The key and value entry
* @return {@code this} builder for use in a chained invocation
*/
public final AnnotationWrapperModule.Builder putMapsToWrap(Map.Entry extends TypeLiteral>, ? extends TypeLiteral>> entry) {
this.mapsToWrap.put(entry);
return (AnnotationWrapperModule.Builder) this;
}
/**
* Sets or replaces all mappings from the specified map as entries for the {@link AnnotationWrapperModule#mapsToWrap() mapsToWrap} map. Nulls are not permitted
* @param mapsToWrap The entries that will be added to the mapsToWrap map
* @return {@code this} builder for use in a chained invocation
*/
public final AnnotationWrapperModule.Builder mapsToWrap(Map extends TypeLiteral>, ? extends TypeLiteral>> mapsToWrap) {
this.mapsToWrap = ImmutableMap.builder();
return putAllMapsToWrap(mapsToWrap);
}
/**
* Put all mappings from the specified map as entries to {@link AnnotationWrapperModule#mapsToWrap() mapsToWrap} map. Nulls are not permitted
* @param mapsToWrap The entries that will be added to the mapsToWrap map
* @return {@code this} builder for use in a chained invocation
*/
public final AnnotationWrapperModule.Builder putAllMapsToWrap(Map extends TypeLiteral>, ? extends TypeLiteral>> mapsToWrap) {
this.mapsToWrap.putAll(mapsToWrap);
return (AnnotationWrapperModule.Builder) this;
}
/**
* Initializes the value for the {@link AnnotationWrapperModule#params() params} attribute.
* @param params The value for params
* @return {@code this} builder for use in a chained invocation
*/
public final AnnotationWrapperModule.Builder params(Parameters params) {
this.params = Preconditions.checkNotNull(params, "params");
initBits &= ~INIT_BIT_PARAMS;
return (AnnotationWrapperModule.Builder) this;
}
/**
* Builds a new {@link AnnotationWrapperModule AnnotationWrapperModule}.
* @return An immutable instance of AnnotationWrapperModule
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public AnnotationWrapperModule build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return ImmutableAnnotationWrapperModule.validate(new ImmutableAnnotationWrapperModule(
wrappedModuleParam,
wrappingAnnotation,
simpleTypesToWrap.build(),
setsToWrap.build(),
mapsToWrap.build(),
params));
}
private String formatRequiredAttributesMessage() {
List attributes = Lists.newArrayList();
if ((initBits & INIT_BIT_WRAPPED_MODULE_PARAM) != 0) attributes.add("wrappedModuleParam");
if ((initBits & INIT_BIT_WRAPPING_ANNOTATION) != 0) attributes.add("wrappingAnnotation");
if ((initBits & INIT_BIT_PARAMS) != 0) attributes.add("params");
return "Cannot build AnnotationWrapperModule, some of required attributes are not set " + attributes;
}
}
}