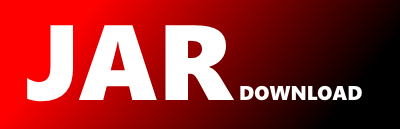
edu.isi.nlp.ImmutableExplicitOrderingNonExclusive Maven / Gradle / Ivy
package edu.isi.nlp;
import com.google.common.base.Preconditions;
import com.google.common.collect.ImmutableMap;
import com.google.common.primitives.Booleans;
import java.util.Map;
import javax.annotation.Generated;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
/**
* Immutable implementation of {@link ExplicitOrderingNonExclusive}.
*
* Use the builder to create immutable instances:
* {@code ImmutableExplicitOrderingNonExclusive.builder()}.
*/
@SuppressWarnings({"all"})
@ParametersAreNonnullByDefault
@Generated({"Immutables.generator", "ExplicitOrderingNonExclusive"})
@Immutable
final class ImmutableExplicitOrderingNonExclusive
extends ExplicitOrderingNonExclusive {
private final ImmutableMap rankMap;
private final boolean unrankedIsFirst;
private ImmutableExplicitOrderingNonExclusive(ImmutableExplicitOrderingNonExclusive.Builder builder) {
this.rankMap = builder.rankMap.build();
this.unrankedIsFirst = builder.unrankedIsFirstIsSet()
? builder.unrankedIsFirst
: super.unrankedIsFirst();
}
private ImmutableExplicitOrderingNonExclusive(ImmutableMap rankMap, boolean unrankedIsFirst) {
this.rankMap = rankMap;
this.unrankedIsFirst = unrankedIsFirst;
}
/**
* @return The value of the {@code rankMap} attribute
*/
@Override
ImmutableMap rankMap() {
return rankMap;
}
/**
* @return The value of the {@code unrankedIsFirst} attribute
*/
@Override
boolean unrankedIsFirst() {
return unrankedIsFirst;
}
/**
* Copy the current immutable object by replacing the {@link ExplicitOrderingNonExclusive#rankMap() rankMap} map with the specified map.
* Nulls are not permitted as keys or values.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param entries The entries to be added to the rankMap map
* @return A modified copy of {@code this} object
*/
public final ImmutableExplicitOrderingNonExclusive withRankMap(Map extends T, ? extends Integer> entries) {
if (this.rankMap == entries) return this;
ImmutableMap newValue = ImmutableMap.copyOf(entries);
return new ImmutableExplicitOrderingNonExclusive(newValue, this.unrankedIsFirst);
}
/**
* Copy the current immutable object by setting a value for the {@link ExplicitOrderingNonExclusive#unrankedIsFirst() unrankedIsFirst} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for unrankedIsFirst
* @return A modified copy of the {@code this} object
*/
public final ImmutableExplicitOrderingNonExclusive withUnrankedIsFirst(boolean value) {
if (this.unrankedIsFirst == value) return this;
return new ImmutableExplicitOrderingNonExclusive(this.rankMap, value);
}
/**
* This instance is equal to all instances of {@code ImmutableExplicitOrderingNonExclusive} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@SuppressWarnings("unchecked")
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableExplicitOrderingNonExclusive>
&& equalTo((ImmutableExplicitOrderingNonExclusive) another);
}
private boolean equalTo(ImmutableExplicitOrderingNonExclusive another) {
return rankMap.equals(another.rankMap)
&& unrankedIsFirst == another.unrankedIsFirst;
}
/**
* Computes a hash code from attributes: {@code rankMap}, {@code unrankedIsFirst}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 31;
h = h * 17 + rankMap.hashCode();
h = h * 17 + Booleans.hashCode(unrankedIsFirst);
return h;
}
/**
* Creates an immutable copy of a {@link ExplicitOrderingNonExclusive} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param generic parameter T
* @param instance The instance to copy
* @return A copied immutable ExplicitOrderingNonExclusive instance
*/
public static ExplicitOrderingNonExclusive copyOf(ExplicitOrderingNonExclusive instance) {
if (instance instanceof ImmutableExplicitOrderingNonExclusive>) {
return (ImmutableExplicitOrderingNonExclusive) instance;
}
return ImmutableExplicitOrderingNonExclusive.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ExplicitOrderingNonExclusive ExplicitOrderingNonExclusive}.
* @param generic parameter T
* @return A new ExplicitOrderingNonExclusive builder
*/
public static ImmutableExplicitOrderingNonExclusive.Builder builder() {
return new ImmutableExplicitOrderingNonExclusive.Builder();
}
/**
* Builds instances of type {@link ExplicitOrderingNonExclusive ExplicitOrderingNonExclusive}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@NotThreadSafe
static final class Builder {
private static final long OPT_BIT_UNRANKED_IS_FIRST = 0x1L;
private long optBits;
private ImmutableMap.Builder rankMap = ImmutableMap.builder();
private boolean unrankedIsFirst;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code ExplicitOrderingNonExclusive} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* Collection elements and entries will be added, not replaced.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(ExplicitOrderingNonExclusive instance) {
Preconditions.checkNotNull(instance, "instance");
putAllRankMap(instance.rankMap());
unrankedIsFirst(instance.unrankedIsFirst());
return this;
}
/**
* Put one entry to the {@link ExplicitOrderingNonExclusive#rankMap() rankMap} map.
* @param key The key in the rankMap map
* @param value The associated value in the rankMap map
* @return {@code this} builder for use in a chained invocation
*/
public final Builder putRankMap(T key, int value) {
this.rankMap.put(key, value);
return this;
}
/**
* Put one entry to the {@link ExplicitOrderingNonExclusive#rankMap() rankMap} map. Nulls are not permitted
* @param entry The key and value entry
* @return {@code this} builder for use in a chained invocation
*/
public final Builder putRankMap(Map.Entry extends T, ? extends Integer> entry) {
this.rankMap.put(entry);
return this;
}
/**
* Sets or replaces all mappings from the specified map as entries for the {@link ExplicitOrderingNonExclusive#rankMap() rankMap} map. Nulls are not permitted
* @param rankMap The entries that will be added to the rankMap map
* @return {@code this} builder for use in a chained invocation
*/
public final Builder rankMap(Map extends T, ? extends Integer> rankMap) {
this.rankMap = ImmutableMap.builder();
return putAllRankMap(rankMap);
}
/**
* Put all mappings from the specified map as entries to {@link ExplicitOrderingNonExclusive#rankMap() rankMap} map. Nulls are not permitted
* @param rankMap The entries that will be added to the rankMap map
* @return {@code this} builder for use in a chained invocation
*/
public final Builder putAllRankMap(Map extends T, ? extends Integer> rankMap) {
this.rankMap.putAll(rankMap);
return this;
}
/**
* Initializes the value for the {@link ExplicitOrderingNonExclusive#unrankedIsFirst() unrankedIsFirst} attribute.
* If not set, this attribute will have a default value as returned by the initializer of {@link ExplicitOrderingNonExclusive#unrankedIsFirst() unrankedIsFirst}.
* @param unrankedIsFirst The value for unrankedIsFirst
* @return {@code this} builder for use in a chained invocation
*/
public final Builder unrankedIsFirst(boolean unrankedIsFirst) {
this.unrankedIsFirst = unrankedIsFirst;
optBits |= OPT_BIT_UNRANKED_IS_FIRST;
return this;
}
/**
* Builds a new {@link ExplicitOrderingNonExclusive ExplicitOrderingNonExclusive}.
* @return An immutable instance of ExplicitOrderingNonExclusive
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ExplicitOrderingNonExclusive build() {
return new ImmutableExplicitOrderingNonExclusive(this);
}
private boolean unrankedIsFirstIsSet() {
return (optBits & OPT_BIT_UNRANKED_IS_FIRST) != 0;
}
}
}