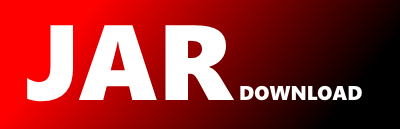
edu.isi.nlp.collections.ImmutableMultitable Maven / Gradle / Ivy
package edu.isi.nlp.collections;
import com.google.common.collect.ImmutableMultimap;
import com.google.common.collect.Multimap;
import com.google.common.collect.Table;
import edu.isi.nlp.IsiNlpImmutable;
import java.util.Collection;
import java.util.Set;
import javax.annotation.Nullable;
import org.immutables.value.Value;
/**
* An immutable {@link Multitable}.
*
* Guava immutable collections generally guarantee that the iteration order is the same as the
* insertion order. In a multiable, for a single pair of row and column indices, multiple insertions
* may occur and the handling of duplicates is specified by the implementation, so it is difficult
* to faithfully recreate the insertion order for iteration. To partially reflect the insertion
* order, iteration over rows and columns reflects the order in which values were first inserted
* into those rows and columns. Cell iteration order is row-major, first following the iteration
* order of rows then of columns.
*
* @author Chester Palen-Michel, Constantine Lignos, Ryan Gabbard
*/
public abstract class ImmutableMultitable extends AbstractMultitable
implements Multitable {
@Override
public boolean contains(@Nullable final Object rowKey, @Nullable final Object columnKey) {
return table().contains(rowKey, columnKey);
}
@Override
public boolean containsRow(@Nullable final Object rowKey) {
return table().containsRow(rowKey);
}
@Override
public boolean containsColumn(@Nullable final Object columnKey) {
return table().containsColumn(columnKey);
}
@Override
public boolean containsValue(@Nullable final Object value) {
for (final R rowKey : table().rowKeySet()) {
for (final C columnKey : table().columnKeySet()) {
final Collection values = table().get(rowKey, columnKey);
if (values != null) {
// The argument must always be of type Object by contract, even though the collection is
// of type V
//noinspection SuspiciousMethodCalls
if (values.contains(value)) {
return true;
}
}
}
}
return false;
}
@Override
public boolean isEmpty() {
return table().isEmpty();
}
@Override
public Set rowKeySet() {
return table().rowKeySet();
}
@Override
public Set columnKeySet() {
return table().columnKeySet();
}
@Override
public Multimap row(final R rowKey) {
final Multimap row = rowMap().get(rowKey);
if (row != null) {
return row;
} else {
return ImmutableMultimap.of();
}
}
@Override
public Multimap column(final C columnKey) {
final Multimap col = columnMap().get(columnKey);
if (col != null) {
return col;
} else {
return ImmutableMultimap.of();
}
}
/**
* Guaranteed to throw an exception and leave the table unmodified.
*
* @throws UnsupportedOperationException always
* @deprecated Unsupported operation.
*/
@Deprecated
@Override
public final void clear() {
throw new UnsupportedOperationException();
}
/**
* Guaranteed to throw an exception and leave the table unmodified.
*
* @throws UnsupportedOperationException always
* @deprecated Unsupported operation.
*/
@Deprecated
@Override
public boolean put(final R rowKey, final C columnKey, final V value) {
throw new UnsupportedOperationException();
}
/**
* Guaranteed to throw an exception and leave the table unmodified.
*
* @throws UnsupportedOperationException always
* @deprecated Unsupported operation.
*/
@Deprecated
@Override
public boolean putAll(final R rowKey, final C columnKey, final Iterable extends V> values) {
throw new UnsupportedOperationException();
}
/**
* Guaranteed to throw an exception and leave the table unmodified.
*
* @throws UnsupportedOperationException always
* @deprecated Unsupported operation.
*/
@Deprecated
@Override
public boolean putAll(final Multitable extends R, ? extends C, ? extends V> table) {
throw new UnsupportedOperationException();
}
/**
* Guaranteed to throw an exception and leave the table unmodified.
*
* @throws UnsupportedOperationException always
* @deprecated Unsupported operation.
*/
@Deprecated
@Override
public boolean remove(
@Nullable final Object rowKey, @Nullable final Object columnKey, final Object value) {
throw new UnsupportedOperationException();
}
/**
* Guaranteed to throw an exception and leave the table unmodified.
*
* @throws UnsupportedOperationException always
* @deprecated Unsupported operation.
*/
@Deprecated
@Override
public boolean removeAll(@Nullable final Object rowKey, @Nullable final Object columnKey) {
throw new UnsupportedOperationException();
}
/** Returns the backing table. */
protected abstract Table> table();
/** For use in {@code ImmutableMultitable} builders only. */
@IsiNlpImmutable
@Value.Immutable
abstract static class RowKeyColumnKeyPair {
public abstract R row();
public abstract C column();
public static RowKeyColumnKeyPair of(R row, C col) {
return ImmutableRowKeyColumnKeyPair.builder().row(row).column(col).build();
}
}
public interface Builder {
ImmutableMultitable.Builder put(R rowKey, C columnKey, V value);
ImmutableMultitable.Builder putAll(
final R rowKey, final C columnKey, final Iterable extends V> values);
ImmutableMultitable build();
}
}