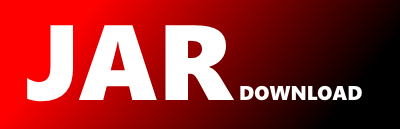
edu.isi.nlp.collections.ImmutableOverlappingRangeSet Maven / Gradle / Ivy
The newest version!
package edu.isi.nlp.collections;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.ImmutableSet;
import com.google.common.collect.Ordering;
import com.google.common.collect.Range;
import com.google.common.collect.Sets;
import java.util.Collection;
import java.util.Set;
/**
* A Naive implementation of an OverlappingRangeSet - uses O(nlog(n)) operation to construct the
* object, then uses O(n) operations to find an offending ranges)
*
* @author Jay DeYoung
*/
public final class ImmutableOverlappingRangeSet>
implements OverlappingRangeSet {
private final ImmutableList> ranges;
private ImmutableOverlappingRangeSet(final Iterable> ranges) {
this.ranges = ImmutableOverlappingRangeSet.rangeOrdering().immutableSortedCopy(ranges);
}
public static > ImmutableOverlappingRangeSet create(
final Iterable> ranges) {
return new ImmutableOverlappingRangeSet(ranges);
}
/**
* Returns all ranges for which {@link Range#contains(Comparable)} item is true, without
* preserving multiplicity.
*/
@Override
public Collection> rangesContaining(final T item) {
final ImmutableSet.Builder> ret = ImmutableSet.builder();
for (final Range range : ranges) {
if (range.contains(item)) {
ret.add(range);
}
}
return ret.build();
}
/**
* Finds every range in this object for which {@code range}.{@link Range#encloses(Range)} {@code
* queryRange}, without preserving multiplicity.
*/
@Override
public Collection> rangesContaining(final Range queryRange) {
final ImmutableSet.Builder> ret = ImmutableSet.builder();
for (final Range range : ranges) {
if (range.encloses(queryRange)) {
ret.add(range);
}
}
return ret.build();
}
/**
* Finds every range in this object for which {@code queryRange}.{@link Range#encloses(Range)},
* without preserving multiplicity.
*/
@Override
public Collection> rangesContainedBy(final Range queryRange) {
final ImmutableSet.Builder> ret = ImmutableSet.builder();
for (final Range range : ranges) {
if (queryRange.encloses(range)) {
ret.add(range);
}
}
return ret.build();
}
/**
* Returns all {@link Range}s {@link Range#isConnected(Range)} to the {@code queryRange} for which
* {@link Range#isEmpty()} is false. Does not preserve multiplicity of {@link Range}s
*/
@Override
public Collection> rangesOverlapping(final Range queryRange) {
final ImmutableSet.Builder> ret = ImmutableSet.builder();
for (final Range range : ranges) {
if (range.isConnected(queryRange) && !range.intersection(queryRange).isEmpty()) {
ret.add(range);
}
}
return ret.build();
}
private static > Ordering> rangeOrdering() {
return Ordering.natural()
.onResultOf(RangeUtils.lowerEndPointFunction())
.compound(Ordering.natural().onResultOf(RangeUtils.upperEndPointFunction()))
.compound(Ordering.usingToString());
}
public static > Builder builder() {
return new Builder<>();
}
public static class Builder> {
private final Set> ranges = Sets.newHashSet();
public void addRange(final Range range) {
ranges.add(range);
}
public void addRanges(final Collection> ranges) {
this.ranges.addAll(ranges);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy