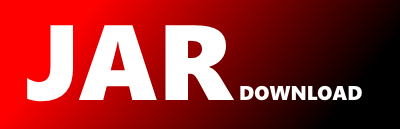
edu.isi.nlp.collections.ShufflingIterable Maven / Gradle / Ivy
The newest version!
package edu.isi.nlp.collections;
import static com.google.common.base.Preconditions.checkNotNull;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.Lists;
import java.util.Collections;
import java.util.Iterator;
import java.util.List;
import java.util.Random;
/**
* This is an {@link Iterable} which provides its data in a random order every time an iterator is
* requested. Note this must load its entire underlying {@link Iterable} into memory.
*
* @author Ryan Gabbard, Constantine Lignos
*/
public final class ShufflingIterable implements Iterable {
private final ImmutableList data;
private final Random rng;
private ShufflingIterable(final Iterable iterable, final Random rng) {
this.data = ImmutableList.copyOf(iterable);
this.rng = checkNotNull(rng);
}
/**
* Returns a new {@link ShufflingIterable} for the specified iterable and random number generator.
*
* @param iterable the source iterable
* @param rng the random number generator to use in shuffling the iterable
* @return a new shuffling iterable for contents of the original iterable
*/
public static ShufflingIterable from(final Iterable iterable, final Random rng) {
return new ShufflingIterable<>(iterable, rng);
}
/** Returns a new iterator that iterates over a new random ordering of the data. */
@Override
public Iterator iterator() {
final List shuffledList = Lists.newArrayList(data);
Collections.shuffle(shuffledList, rng);
return Collections.unmodifiableList(shuffledList).iterator();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy