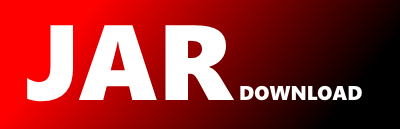
edu.isi.nlp.files.ImmutableMultitableLoader Maven / Gradle / Ivy
package edu.isi.nlp.files;
import com.google.common.base.Function;
import com.google.common.base.MoreObjects;
import com.google.common.base.Optional;
import com.google.common.base.Preconditions;
import com.google.common.base.Splitter;
import com.google.common.collect.Lists;
import java.util.List;
import javax.annotation.Generated;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
/**
* Immutable implementation of {@link MultitableLoader}.
*
* Use the builder to create immutable instances:
* {@code new MultitableLoader.Builder()}.
*/
@SuppressWarnings({"all"})
@ParametersAreNonnullByDefault
@Generated({"Immutables.generator", "MultitableLoader"})
@Immutable
final class ImmutableMultitableLoader extends MultitableLoader {
private final Optional valueListSplitter;
private final Splitter fieldSplitter;
private final Function rowInterpreter;
private final Function columnInterpreter;
private final Function valueInterpreter;
private ImmutableMultitableLoader(ImmutableMultitableLoader.Builder builder) {
this.valueListSplitter = builder.valueListSplitter;
this.rowInterpreter = builder.rowInterpreter;
this.columnInterpreter = builder.columnInterpreter;
this.valueInterpreter = builder.valueInterpreter;
this.fieldSplitter = builder.fieldSplitter != null
? builder.fieldSplitter
: Preconditions.checkNotNull(super.fieldSplitter(), "fieldSplitter");
}
private ImmutableMultitableLoader(
Optional valueListSplitter,
Splitter fieldSplitter,
Function rowInterpreter,
Function columnInterpreter,
Function valueInterpreter) {
this.valueListSplitter = valueListSplitter;
this.fieldSplitter = fieldSplitter;
this.rowInterpreter = rowInterpreter;
this.columnInterpreter = columnInterpreter;
this.valueInterpreter = valueInterpreter;
}
/**
* @return The value of the {@code valueListSplitter} attribute
*/
@Override
public Optional valueListSplitter() {
return valueListSplitter;
}
/**
* @return The value of the {@code fieldSplitter} attribute
*/
@Override
public Splitter fieldSplitter() {
return fieldSplitter;
}
/**
* @return The value of the {@code rowInterpreter} attribute
*/
@Override
public Function rowInterpreter() {
return rowInterpreter;
}
/**
* @return The value of the {@code columnInterpreter} attribute
*/
@Override
public Function columnInterpreter() {
return columnInterpreter;
}
/**
* @return The value of the {@code valueInterpreter} attribute
*/
@Override
public Function valueInterpreter() {
return valueInterpreter;
}
/**
* Copy the current immutable object by setting a present value for the optional {@link MultitableLoader#valueListSplitter() valueListSplitter} attribute.
* @param value The value for valueListSplitter
* @return A modified copy of {@code this} object
*/
public final ImmutableMultitableLoader withValueListSplitter(Splitter value) {
Optional newValue = Optional.of(value);
if (this.valueListSplitter.isPresent() && this.valueListSplitter.get() == value) return this;
return new ImmutableMultitableLoader(
newValue,
this.fieldSplitter,
this.rowInterpreter,
this.columnInterpreter,
this.valueInterpreter);
}
/**
* Copy the current immutable object by setting an optional value for the {@link MultitableLoader#valueListSplitter() valueListSplitter} attribute.
* A shallow reference equality check on the optional value is used to prevent copying of the same value by returning {@code this}.
* @param optional A value for valueListSplitter
* @return A modified copy of {@code this} object
*/
public final ImmutableMultitableLoader withValueListSplitter(Optional optional) {
Optional value = Preconditions.checkNotNull(optional, "valueListSplitter");
if (!this.valueListSplitter.isPresent() && !value.isPresent()) return this;
if (this.valueListSplitter.isPresent() && value.isPresent() && this.valueListSplitter.get() == value.get()) return this;
return new ImmutableMultitableLoader(value, this.fieldSplitter, this.rowInterpreter, this.columnInterpreter, this.valueInterpreter);
}
/**
* Copy the current immutable object by setting a value for the {@link MultitableLoader#fieldSplitter() fieldSplitter} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for fieldSplitter
* @return A modified copy of the {@code this} object
*/
public final ImmutableMultitableLoader withFieldSplitter(Splitter value) {
if (this.fieldSplitter == value) return this;
Splitter newValue = Preconditions.checkNotNull(value, "fieldSplitter");
return new ImmutableMultitableLoader(
this.valueListSplitter,
newValue,
this.rowInterpreter,
this.columnInterpreter,
this.valueInterpreter);
}
/**
* Copy the current immutable object by setting a value for the {@link MultitableLoader#rowInterpreter() rowInterpreter} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for rowInterpreter
* @return A modified copy of the {@code this} object
*/
public final ImmutableMultitableLoader withRowInterpreter(Function value) {
if (this.rowInterpreter == value) return this;
Function newValue = Preconditions.checkNotNull(value, "rowInterpreter");
return new ImmutableMultitableLoader(
this.valueListSplitter,
this.fieldSplitter,
newValue,
this.columnInterpreter,
this.valueInterpreter);
}
/**
* Copy the current immutable object by setting a value for the {@link MultitableLoader#columnInterpreter() columnInterpreter} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for columnInterpreter
* @return A modified copy of the {@code this} object
*/
public final ImmutableMultitableLoader withColumnInterpreter(Function value) {
if (this.columnInterpreter == value) return this;
Function newValue = Preconditions.checkNotNull(value, "columnInterpreter");
return new ImmutableMultitableLoader(
this.valueListSplitter,
this.fieldSplitter,
this.rowInterpreter,
newValue,
this.valueInterpreter);
}
/**
* Copy the current immutable object by setting a value for the {@link MultitableLoader#valueInterpreter() valueInterpreter} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for valueInterpreter
* @return A modified copy of the {@code this} object
*/
public final ImmutableMultitableLoader withValueInterpreter(Function value) {
if (this.valueInterpreter == value) return this;
Function newValue = Preconditions.checkNotNull(value, "valueInterpreter");
return new ImmutableMultitableLoader(
this.valueListSplitter,
this.fieldSplitter,
this.rowInterpreter,
this.columnInterpreter,
newValue);
}
/**
* This instance is equal to all instances of {@code ImmutableMultitableLoader} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@SuppressWarnings("unchecked")
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableMultitableLoader, ?, ?>
&& equalTo((ImmutableMultitableLoader) another);
}
private boolean equalTo(ImmutableMultitableLoader another) {
return valueListSplitter.equals(another.valueListSplitter)
&& fieldSplitter.equals(another.fieldSplitter)
&& rowInterpreter.equals(another.rowInterpreter)
&& columnInterpreter.equals(another.columnInterpreter)
&& valueInterpreter.equals(another.valueInterpreter);
}
/**
* Computes a hash code from attributes: {@code valueListSplitter}, {@code fieldSplitter}, {@code rowInterpreter}, {@code columnInterpreter}, {@code valueInterpreter}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 31;
h = h * 17 + valueListSplitter.hashCode();
h = h * 17 + fieldSplitter.hashCode();
h = h * 17 + rowInterpreter.hashCode();
h = h * 17 + columnInterpreter.hashCode();
h = h * 17 + valueInterpreter.hashCode();
return h;
}
/**
* Prints the immutable value {@code MultitableLoader} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return MoreObjects.toStringHelper("MultitableLoader")
.omitNullValues()
.add("valueListSplitter", valueListSplitter.orNull())
.add("fieldSplitter", fieldSplitter)
.add("rowInterpreter", rowInterpreter)
.add("columnInterpreter", columnInterpreter)
.add("valueInterpreter", valueInterpreter)
.toString();
}
/**
* Creates an immutable copy of a {@link MultitableLoader} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param generic parameter R
* @param generic parameter C
* @param generic parameter V
* @param instance The instance to copy
* @return A copied immutable MultitableLoader instance
*/
public static MultitableLoader copyOf(MultitableLoader instance) {
if (instance instanceof ImmutableMultitableLoader, ?, ?>) {
return (ImmutableMultitableLoader) instance;
}
return new MultitableLoader.Builder()
.from(instance)
.build();
}
/**
* Builds instances of type {@link MultitableLoader MultitableLoader}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@NotThreadSafe
static class Builder {
private static final long INIT_BIT_ROW_INTERPRETER = 0x1L;
private static final long INIT_BIT_COLUMN_INTERPRETER = 0x2L;
private static final long INIT_BIT_VALUE_INTERPRETER = 0x4L;
private long initBits = 0x7L;
private Optional valueListSplitter = Optional.absent();
private @Nullable Splitter fieldSplitter;
private @Nullable Function rowInterpreter;
private @Nullable Function columnInterpreter;
private @Nullable Function valueInterpreter;
/**
* Creates a builder for {@link MultitableLoader MultitableLoader} instances.
*/
Builder() {
if (!(this instanceof MultitableLoader.Builder, ?, ?>)) {
throw new UnsupportedOperationException("Use: new MultitableLoader.Builder()");
}
}
/**
* Fill a builder with attribute values from the provided {@code MultitableLoader} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final MultitableLoader.Builder from(MultitableLoader instance) {
Preconditions.checkNotNull(instance, "instance");
Optional valueListSplitterOptional = instance.valueListSplitter();
if (valueListSplitterOptional.isPresent()) {
valueListSplitter(valueListSplitterOptional);
}
fieldSplitter(instance.fieldSplitter());
rowInterpreter(instance.rowInterpreter());
columnInterpreter(instance.columnInterpreter());
valueInterpreter(instance.valueInterpreter());
return (MultitableLoader.Builder) this;
}
/**
* Initializes the optional value {@link MultitableLoader#valueListSplitter() valueListSplitter} to valueListSplitter.
* @param valueListSplitter The value for valueListSplitter
* @return {@code this} builder for chained invocation
*/
public final MultitableLoader.Builder valueListSplitter(Splitter valueListSplitter) {
this.valueListSplitter = Optional.of(valueListSplitter);
return (MultitableLoader.Builder) this;
}
/**
* Initializes the optional value {@link MultitableLoader#valueListSplitter() valueListSplitter} to valueListSplitter.
* @param valueListSplitter The value for valueListSplitter
* @return {@code this} builder for use in a chained invocation
*/
public final MultitableLoader.Builder valueListSplitter(Optional valueListSplitter) {
this.valueListSplitter = Preconditions.checkNotNull(valueListSplitter, "valueListSplitter");
return (MultitableLoader.Builder) this;
}
/**
* Initializes the value for the {@link MultitableLoader#fieldSplitter() fieldSplitter} attribute.
* If not set, this attribute will have a default value as returned by the initializer of {@link MultitableLoader#fieldSplitter() fieldSplitter}.
* @param fieldSplitter The value for fieldSplitter
* @return {@code this} builder for use in a chained invocation
*/
public final MultitableLoader.Builder fieldSplitter(Splitter fieldSplitter) {
this.fieldSplitter = Preconditions.checkNotNull(fieldSplitter, "fieldSplitter");
return (MultitableLoader.Builder) this;
}
/**
* Initializes the value for the {@link MultitableLoader#rowInterpreter() rowInterpreter} attribute.
* @param rowInterpreter The value for rowInterpreter
* @return {@code this} builder for use in a chained invocation
*/
public final MultitableLoader.Builder rowInterpreter(Function rowInterpreter) {
this.rowInterpreter = Preconditions.checkNotNull(rowInterpreter, "rowInterpreter");
initBits &= ~INIT_BIT_ROW_INTERPRETER;
return (MultitableLoader.Builder) this;
}
/**
* Initializes the value for the {@link MultitableLoader#columnInterpreter() columnInterpreter} attribute.
* @param columnInterpreter The value for columnInterpreter
* @return {@code this} builder for use in a chained invocation
*/
public final MultitableLoader.Builder columnInterpreter(Function columnInterpreter) {
this.columnInterpreter = Preconditions.checkNotNull(columnInterpreter, "columnInterpreter");
initBits &= ~INIT_BIT_COLUMN_INTERPRETER;
return (MultitableLoader.Builder) this;
}
/**
* Initializes the value for the {@link MultitableLoader#valueInterpreter() valueInterpreter} attribute.
* @param valueInterpreter The value for valueInterpreter
* @return {@code this} builder for use in a chained invocation
*/
public final MultitableLoader.Builder valueInterpreter(Function valueInterpreter) {
this.valueInterpreter = Preconditions.checkNotNull(valueInterpreter, "valueInterpreter");
initBits &= ~INIT_BIT_VALUE_INTERPRETER;
return (MultitableLoader.Builder) this;
}
/**
* Builds a new {@link MultitableLoader MultitableLoader}.
* @return An immutable instance of MultitableLoader
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public MultitableLoader build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return new ImmutableMultitableLoader(this);
}
private String formatRequiredAttributesMessage() {
List attributes = Lists.newArrayList();
if ((initBits & INIT_BIT_ROW_INTERPRETER) != 0) attributes.add("rowInterpreter");
if ((initBits & INIT_BIT_COLUMN_INTERPRETER) != 0) attributes.add("columnInterpreter");
if ((initBits & INIT_BIT_VALUE_INTERPRETER) != 0) attributes.add("valueInterpreter");
return "Cannot build MultitableLoader, some of required attributes are not set " + attributes;
}
}
}