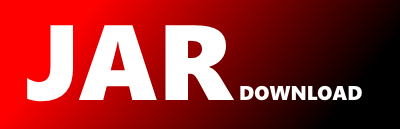
edu.isi.nlp.serialization.jackson.ImmutableMapProxy Maven / Gradle / Ivy
The newest version!
package edu.isi.nlp.serialization.jackson;
import static com.google.common.base.Preconditions.checkArgument;
import static com.google.common.base.Preconditions.checkNotNull;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.google.common.collect.ImmutableMap;
import com.google.common.collect.Lists;
import edu.isi.nlp.collections.MapUtils;
import java.util.List;
import java.util.Map;
/**
* Works around a Jackson issue in serializing maps. Jackson, frustratingly, serializes maps to JSON
* by turning the keys into Strings, which typically don't want. To get around this:
*
*
* - Don't make the map itself a {@code JsonProperty}
*
- Provide a {@code JsonProperty}-annotated package-private accessor which returns {@code
* ImmutableMapProxy.forMap(myMap)}
*
- In your {@code JsonCreator} method, take an {@code ImmutableMapProxy} as an argument and
* call {@link #toImmutableMap()}
*
*
* @deprecated Prefer {@link MapEntries}
*/
@Deprecated
public final class ImmutableMapProxy {
@JsonProperty("keys")
private final List keys;
@JsonProperty("values")
private final List values;
private ImmutableMapProxy(
@JsonProperty("keys") final List keys, @JsonProperty("values") final List values) {
this.keys = checkNotNull(keys);
this.values = checkNotNull(values);
checkArgument(keys.size() == values.size());
}
@Deprecated
@SuppressWarnings("deprecation")
public static ImmutableMapProxy forMap(Map map) {
final List keys = Lists.newArrayListWithCapacity(map.size());
final List values = Lists.newArrayListWithCapacity(map.size());
for (final Map.Entry e : map.entrySet()) {
keys.add(e.getKey());
values.add(e.getValue());
}
return new ImmutableMapProxy(keys, values);
}
public ImmutableMap toImmutableMap() {
return MapUtils.copyParallelListsToMap(keys, values);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy