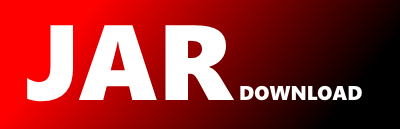
edu.isi.nlp.strings.ImmutableLocatedString Maven / Gradle / Ivy
package edu.isi.nlp.strings;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.google.common.base.Optional;
import com.google.common.base.Preconditions;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.Lists;
import com.google.common.primitives.Booleans;
import edu.isi.nlp.UnicodeFriendlyString;
import edu.isi.nlp.strings.offsets.CharOffset;
import edu.isi.nlp.strings.offsets.OffsetGroup;
import edu.isi.nlp.strings.offsets.OffsetGroupRange;
import java.util.ArrayList;
import java.util.List;
import javax.annotation.Generated;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
/**
* Immutable implementation of {@link LocatedString}.
*
* Use the builder to create immutable instances:
* {@code new LocatedString.Builder()}.
*/
@SuppressWarnings({"all"})
@ParametersAreNonnullByDefault
@Generated({"Immutables.generator", "LocatedString"})
@Immutable
final class ImmutableLocatedString extends LocatedString {
private final UnicodeFriendlyString content;
private final Optional referenceString;
private final ImmutableList characterRegions;
private final OffsetGroupRange referenceBounds;
private final boolean referenceCharOffsetsSequential;
private final int hashCode;
private ImmutableLocatedString(
UnicodeFriendlyString content,
Optional referenceString,
ImmutableList characterRegions) {
this.content = content;
this.referenceString = referenceString;
this.characterRegions = characterRegions;
this.referenceBounds = initShim.referenceBounds();
this.referenceCharOffsetsSequential = initShim.referenceCharOffsetsSequential();
this.hashCode = computeHashCode();
this.initShim = null;
}
private static final int STAGE_INITIALIZING = -1;
private static final int STAGE_UNINITIALIZED = 0;
private static final int STAGE_INITIALIZED = 1;
private transient volatile InitShim initShim = new InitShim();
private final class InitShim {
private OffsetGroupRange referenceBounds;
private int referenceBoundsStage;
OffsetGroupRange referenceBounds() {
if (referenceBoundsStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (referenceBoundsStage == STAGE_UNINITIALIZED) {
referenceBoundsStage = STAGE_INITIALIZING;
this.referenceBounds = Preconditions.checkNotNull(ImmutableLocatedString.super.referenceBounds(), "referenceBounds");
referenceBoundsStage = STAGE_INITIALIZED;
}
return this.referenceBounds;
}
private boolean referenceCharOffsetsSequential;
private int referenceCharOffsetsSequentialStage;
boolean referenceCharOffsetsSequential() {
if (referenceCharOffsetsSequentialStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (referenceCharOffsetsSequentialStage == STAGE_UNINITIALIZED) {
referenceCharOffsetsSequentialStage = STAGE_INITIALIZING;
this.referenceCharOffsetsSequential = ImmutableLocatedString.super.referenceCharOffsetsSequential();
referenceCharOffsetsSequentialStage = STAGE_INITIALIZED;
}
return this.referenceCharOffsetsSequential;
}
private String formatInitCycleMessage() {
ArrayList attributes = Lists.newArrayList();
if (referenceBoundsStage == STAGE_INITIALIZING) attributes.add("referenceBounds");
if (referenceCharOffsetsSequentialStage == STAGE_INITIALIZING) attributes.add("referenceCharOffsetsSequential");
return "Cannot build LocatedString, attribute initializers form cycle" + attributes;
}
}
/**
* @return The value of the {@code content} attribute
*/
@JsonProperty("content")
@Override
public UnicodeFriendlyString content() {
return content;
}
/**
* @return The value of the {@code referenceString} attribute
*/
@JsonProperty("referenceString")
@Override
public Optional referenceString() {
return referenceString;
}
/**
* @return The value of the {@code characterRegions} attribute
*/
@JsonProperty("characterRegions")
@Override
public ImmutableList characterRegions() {
return characterRegions;
}
/**
* @return The computed-at-construction value of the {@code referenceBounds} attribute
*/
@JsonProperty("referenceBounds")
@Override
public OffsetGroupRange referenceBounds() {
InitShim shim = this.initShim;
return shim != null
? shim.referenceBounds()
: this.referenceBounds;
}
/**
* @return The computed-at-construction value of the {@code referenceCharOffsetsSequential} attribute
*/
@JsonProperty("referenceCharOffsetsSequential")
@Override
protected boolean referenceCharOffsetsSequential() {
InitShim shim = this.initShim;
return shim != null
? shim.referenceCharOffsetsSequential()
: this.referenceCharOffsetsSequential;
}
/**
* Copy the current immutable object by setting a value for the {@link LocatedString#content() content} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for content
* @return A modified copy of the {@code this} object
*/
public final ImmutableLocatedString withContent(UnicodeFriendlyString value) {
if (this.content == value) return this;
UnicodeFriendlyString newValue = Preconditions.checkNotNull(value, "content");
return validate(new ImmutableLocatedString(newValue, this.referenceString, this.characterRegions));
}
/**
* Copy the current immutable object by setting a present value for the optional {@link LocatedString#referenceString() referenceString} attribute.
* @param value The value for referenceString
* @return A modified copy of {@code this} object
*/
public final ImmutableLocatedString withReferenceString(UnicodeFriendlyString value) {
Optional newValue = Optional.of(value);
if (this.referenceString.isPresent() && this.referenceString.get() == value) return this;
return validate(new ImmutableLocatedString(this.content, newValue, this.characterRegions));
}
/**
* Copy the current immutable object by setting an optional value for the {@link LocatedString#referenceString() referenceString} attribute.
* A shallow reference equality check on the optional value is used to prevent copying of the same value by returning {@code this}.
* @param optional A value for referenceString
* @return A modified copy of {@code this} object
*/
public final ImmutableLocatedString withReferenceString(Optional optional) {
Optional value = Preconditions.checkNotNull(optional, "referenceString");
if (!this.referenceString.isPresent() && !value.isPresent()) return this;
if (this.referenceString.isPresent() && value.isPresent() && this.referenceString.get() == value.get()) return this;
return validate(new ImmutableLocatedString(this.content, value, this.characterRegions));
}
/**
* Copy the current immutable object with elements that replace the content of {@link LocatedString#characterRegions() characterRegions}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableLocatedString withCharacterRegions(LocatedString.CharacterRegion... elements) {
ImmutableList newValue = ImmutableList.copyOf(elements);
return validate(new ImmutableLocatedString(this.content, this.referenceString, newValue));
}
/**
* Copy the current immutable object with elements that replace the content of {@link LocatedString#characterRegions() characterRegions}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of characterRegions elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableLocatedString withCharacterRegions(Iterable extends LocatedString.CharacterRegion> elements) {
if (this.characterRegions == elements) return this;
ImmutableList newValue = ImmutableList.copyOf(elements);
return validate(new ImmutableLocatedString(this.content, this.referenceString, newValue));
}
/**
* This instance is equal to all instances of {@code ImmutableLocatedString} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableLocatedString
&& equalTo((ImmutableLocatedString) another);
}
private boolean equalTo(ImmutableLocatedString another) {
return content.equals(another.content)
&& referenceString.equals(another.referenceString)
&& characterRegions.equals(another.characterRegions)
&& referenceBounds.equals(another.referenceBounds)
&& referenceCharOffsetsSequential == another.referenceCharOffsetsSequential;
}
/**
* Returns a precomputed-on-construction hash code from attributes: {@code content}, {@code referenceString}, {@code characterRegions}, {@code referenceBounds}, {@code referenceCharOffsetsSequential}.
* @return hashCode value
*/
@Override
public int hashCode() {
return hashCode;
}
private int computeHashCode() {
int h = 31;
h = h * 17 + content.hashCode();
h = h * 17 + referenceString.hashCode();
h = h * 17 + characterRegions.hashCode();
h = h * 17 + referenceBounds.hashCode();
h = h * 17 + Booleans.hashCode(referenceCharOffsetsSequential);
return h;
}
/**
* Utility type used to correctly read immutable object from JSON representation.
* @deprecated Do not use this type directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonDeserialize
@JsonAutoDetect(fieldVisibility = JsonAutoDetect.Visibility.NONE)
static final class Json extends LocatedString {
@Nullable UnicodeFriendlyString content;
Optional referenceString = Optional.absent();
ImmutableList characterRegions = ImmutableList.of();
@JsonProperty("content")
public void setContent(UnicodeFriendlyString content) {
this.content = content;
}
@JsonProperty("referenceString")
public void setReferenceString(Optional referenceString) {
this.referenceString = referenceString;
}
@JsonProperty("characterRegions")
public void setCharacterRegions(ImmutableList characterRegions) {
this.characterRegions = characterRegions;
}
@Override
public UnicodeFriendlyString content() { throw new UnsupportedOperationException(); }
@Override
public Optional referenceString() { throw new UnsupportedOperationException(); }
@Override
public ImmutableList characterRegions() { throw new UnsupportedOperationException(); }
@Override
public OffsetGroupRange referenceBounds() { throw new UnsupportedOperationException(); }
@Override
protected boolean referenceCharOffsetsSequential() { throw new UnsupportedOperationException(); }
}
/**
* @param json A JSON-bindable data structure
* @return An immutable value type
* @deprecated Do not use this method directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonCreator
static ImmutableLocatedString fromJson(Json json) {
LocatedString.Builder builder = new LocatedString.Builder();
if (json.content != null) {
builder.content(json.content);
}
if (json.referenceString != null) {
builder.referenceString(json.referenceString);
}
if (json.characterRegions != null) {
builder.addAllCharacterRegions(json.characterRegions);
}
return (ImmutableLocatedString) builder.build();
}
private static ImmutableLocatedString validate(ImmutableLocatedString instance) {
instance = (ImmutableLocatedString) instance.checkValidity();
return instance;
}
/**
* Creates an immutable copy of a {@link LocatedString} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable LocatedString instance
*/
public static LocatedString copyOf(LocatedString instance) {
if (instance instanceof ImmutableLocatedString) {
return (ImmutableLocatedString) instance;
}
return new LocatedString.Builder()
.from(instance)
.build();
}
/**
* Builds instances of type {@link LocatedString LocatedString}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@NotThreadSafe
static class Builder {
private static final long INIT_BIT_CONTENT = 0x1L;
private long initBits = 0x1L;
private @Nullable UnicodeFriendlyString content;
private Optional referenceString = Optional.absent();
private ImmutableList.Builder characterRegions = ImmutableList.builder();
/**
* Creates a builder for {@link LocatedString LocatedString} instances.
*/
Builder() {
if (!(this instanceof LocatedString.Builder)) {
throw new UnsupportedOperationException("Use: new LocatedString.Builder()");
}
}
/**
* Fill a builder with attribute values from the provided {@code LocatedString} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* Collection elements and entries will be added, not replaced.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final LocatedString.Builder from(LocatedString instance) {
Preconditions.checkNotNull(instance, "instance");
content(instance.content());
Optional referenceStringOptional = instance.referenceString();
if (referenceStringOptional.isPresent()) {
referenceString(referenceStringOptional);
}
addAllCharacterRegions(instance.characterRegions());
return (LocatedString.Builder) this;
}
/**
* Initializes the value for the {@link LocatedString#content() content} attribute.
* @param content The value for content
* @return {@code this} builder for use in a chained invocation
*/
public final LocatedString.Builder content(UnicodeFriendlyString content) {
this.content = Preconditions.checkNotNull(content, "content");
initBits &= ~INIT_BIT_CONTENT;
return (LocatedString.Builder) this;
}
/**
* Initializes the optional value {@link LocatedString#referenceString() referenceString} to referenceString.
* @param referenceString The value for referenceString
* @return {@code this} builder for chained invocation
*/
public final LocatedString.Builder referenceString(UnicodeFriendlyString referenceString) {
this.referenceString = Optional.of(referenceString);
return (LocatedString.Builder) this;
}
/**
* Initializes the optional value {@link LocatedString#referenceString() referenceString} to referenceString.
* @param referenceString The value for referenceString
* @return {@code this} builder for use in a chained invocation
*/
public final LocatedString.Builder referenceString(Optional referenceString) {
this.referenceString = Preconditions.checkNotNull(referenceString, "referenceString");
return (LocatedString.Builder) this;
}
/**
* Adds one element to {@link LocatedString#characterRegions() characterRegions} list.
* @param element A characterRegions element
* @return {@code this} builder for use in a chained invocation
*/
public final LocatedString.Builder addCharacterRegions(LocatedString.CharacterRegion element) {
this.characterRegions.add(element);
return (LocatedString.Builder) this;
}
/**
* Adds elements to {@link LocatedString#characterRegions() characterRegions} list.
* @param elements An array of characterRegions elements
* @return {@code this} builder for use in a chained invocation
*/
public final LocatedString.Builder addCharacterRegions(LocatedString.CharacterRegion... elements) {
this.characterRegions.add(elements);
return (LocatedString.Builder) this;
}
/**
* Sets or replaces all elements for {@link LocatedString#characterRegions() characterRegions} list.
* @param elements An iterable of characterRegions elements
* @return {@code this} builder for use in a chained invocation
*/
public final LocatedString.Builder characterRegions(Iterable extends LocatedString.CharacterRegion> elements) {
this.characterRegions = ImmutableList.builder();
return addAllCharacterRegions(elements);
}
/**
* Adds elements to {@link LocatedString#characterRegions() characterRegions} list.
* @param elements An iterable of characterRegions elements
* @return {@code this} builder for use in a chained invocation
*/
public final LocatedString.Builder addAllCharacterRegions(Iterable extends LocatedString.CharacterRegion> elements) {
this.characterRegions.addAll(elements);
return (LocatedString.Builder) this;
}
/**
* Builds a new {@link LocatedString LocatedString}.
* @return An immutable instance of LocatedString
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public LocatedString build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return ImmutableLocatedString.validate(new ImmutableLocatedString(content, referenceString, characterRegions.build()));
}
private String formatRequiredAttributesMessage() {
List attributes = Lists.newArrayList();
if ((initBits & INIT_BIT_CONTENT) != 0) attributes.add("content");
return "Cannot build LocatedString, some of required attributes are not set " + attributes;
}
}
/**
* Immutable implementation of {@link LocatedString.CharacterRegion}.
*
* Use the builder to create immutable instances:
* {@code new LocatedString.CharacterRegion.Builder()}.
*/
@Immutable
static final class CharacterRegion extends LocatedString.CharacterRegion {
private final boolean contentNonBmp;
private final CharOffset contentStartPosInclusive;
private final CharOffset contentEndPosExclusive;
private final OffsetGroup referenceStartOffsetInclusive;
private final OffsetGroup referenceEndOffsetInclusive;
private final int hashCode;
private CharacterRegion(
boolean contentNonBmp,
CharOffset contentStartPosInclusive,
CharOffset contentEndPosExclusive,
OffsetGroup referenceStartOffsetInclusive,
OffsetGroup referenceEndOffsetInclusive) {
this.contentNonBmp = contentNonBmp;
this.contentStartPosInclusive = contentStartPosInclusive;
this.contentEndPosExclusive = contentEndPosExclusive;
this.referenceStartOffsetInclusive = referenceStartOffsetInclusive;
this.referenceEndOffsetInclusive = referenceEndOffsetInclusive;
this.hashCode = computeHashCode();
}
/**
* @return The value of the {@code contentNonBmp} attribute
*/
@JsonProperty("contentNonBmp")
@Override
public boolean contentNonBmp() {
return contentNonBmp;
}
/**
* @return The value of the {@code contentStartPosInclusive} attribute
*/
@JsonProperty("contentStartPosInclusive")
@Override
public CharOffset contentStartPosInclusive() {
return contentStartPosInclusive;
}
/**
* @return The value of the {@code contentEndPosExclusive} attribute
*/
@JsonProperty("contentEndPosExclusive")
@Override
public CharOffset contentEndPosExclusive() {
return contentEndPosExclusive;
}
/**
* @return The value of the {@code referenceStartOffsetInclusive} attribute
*/
@JsonProperty("referenceStartOffsetInclusive")
@Override
public OffsetGroup referenceStartOffsetInclusive() {
return referenceStartOffsetInclusive;
}
/**
* @return The value of the {@code referenceEndOffsetInclusive} attribute
*/
@JsonProperty("referenceEndOffsetInclusive")
@Override
public OffsetGroup referenceEndOffsetInclusive() {
return referenceEndOffsetInclusive;
}
/**
* Copy the current immutable object by setting a value for the {@link LocatedString.CharacterRegion#contentNonBmp() contentNonBmp} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for contentNonBmp
* @return A modified copy of the {@code this} object
*/
public final ImmutableLocatedString.CharacterRegion withContentNonBmp(boolean value) {
if (this.contentNonBmp == value) return this;
return validate(new ImmutableLocatedString.CharacterRegion(
value,
this.contentStartPosInclusive,
this.contentEndPosExclusive,
this.referenceStartOffsetInclusive,
this.referenceEndOffsetInclusive));
}
/**
* Copy the current immutable object by setting a value for the {@link LocatedString.CharacterRegion#contentStartPosInclusive() contentStartPosInclusive} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for contentStartPosInclusive
* @return A modified copy of the {@code this} object
*/
public final ImmutableLocatedString.CharacterRegion withContentStartPosInclusive(CharOffset value) {
if (this.contentStartPosInclusive == value) return this;
CharOffset newValue = Preconditions.checkNotNull(value, "contentStartPosInclusive");
return validate(new ImmutableLocatedString.CharacterRegion(
this.contentNonBmp,
newValue,
this.contentEndPosExclusive,
this.referenceStartOffsetInclusive,
this.referenceEndOffsetInclusive));
}
/**
* Copy the current immutable object by setting a value for the {@link LocatedString.CharacterRegion#contentEndPosExclusive() contentEndPosExclusive} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for contentEndPosExclusive
* @return A modified copy of the {@code this} object
*/
public final ImmutableLocatedString.CharacterRegion withContentEndPosExclusive(CharOffset value) {
if (this.contentEndPosExclusive == value) return this;
CharOffset newValue = Preconditions.checkNotNull(value, "contentEndPosExclusive");
return validate(new ImmutableLocatedString.CharacterRegion(
this.contentNonBmp,
this.contentStartPosInclusive,
newValue,
this.referenceStartOffsetInclusive,
this.referenceEndOffsetInclusive));
}
/**
* Copy the current immutable object by setting a value for the {@link LocatedString.CharacterRegion#referenceStartOffsetInclusive() referenceStartOffsetInclusive} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for referenceStartOffsetInclusive
* @return A modified copy of the {@code this} object
*/
public final ImmutableLocatedString.CharacterRegion withReferenceStartOffsetInclusive(OffsetGroup value) {
if (this.referenceStartOffsetInclusive == value) return this;
OffsetGroup newValue = Preconditions.checkNotNull(value, "referenceStartOffsetInclusive");
return validate(new ImmutableLocatedString.CharacterRegion(
this.contentNonBmp,
this.contentStartPosInclusive,
this.contentEndPosExclusive,
newValue,
this.referenceEndOffsetInclusive));
}
/**
* Copy the current immutable object by setting a value for the {@link LocatedString.CharacterRegion#referenceEndOffsetInclusive() referenceEndOffsetInclusive} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for referenceEndOffsetInclusive
* @return A modified copy of the {@code this} object
*/
public final ImmutableLocatedString.CharacterRegion withReferenceEndOffsetInclusive(OffsetGroup value) {
if (this.referenceEndOffsetInclusive == value) return this;
OffsetGroup newValue = Preconditions.checkNotNull(value, "referenceEndOffsetInclusive");
return validate(new ImmutableLocatedString.CharacterRegion(
this.contentNonBmp,
this.contentStartPosInclusive,
this.contentEndPosExclusive,
this.referenceStartOffsetInclusive,
newValue));
}
/**
* This instance is equal to all instances of {@code CharacterRegion} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableLocatedString.CharacterRegion
&& equalTo((ImmutableLocatedString.CharacterRegion) another);
}
private boolean equalTo(ImmutableLocatedString.CharacterRegion another) {
return contentNonBmp == another.contentNonBmp
&& contentStartPosInclusive.equals(another.contentStartPosInclusive)
&& contentEndPosExclusive.equals(another.contentEndPosExclusive)
&& referenceStartOffsetInclusive.equals(another.referenceStartOffsetInclusive)
&& referenceEndOffsetInclusive.equals(another.referenceEndOffsetInclusive);
}
/**
* Returns a precomputed-on-construction hash code from attributes: {@code contentNonBmp}, {@code contentStartPosInclusive}, {@code contentEndPosExclusive}, {@code referenceStartOffsetInclusive}, {@code referenceEndOffsetInclusive}.
* @return hashCode value
*/
@Override
public int hashCode() {
return hashCode;
}
private int computeHashCode() {
int h = 31;
h = h * 17 + Booleans.hashCode(contentNonBmp);
h = h * 17 + contentStartPosInclusive.hashCode();
h = h * 17 + contentEndPosExclusive.hashCode();
h = h * 17 + referenceStartOffsetInclusive.hashCode();
h = h * 17 + referenceEndOffsetInclusive.hashCode();
return h;
}
/**
* Utility type used to correctly read immutable object from JSON representation.
* @deprecated Do not use this type directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonDeserialize
@JsonAutoDetect(fieldVisibility = JsonAutoDetect.Visibility.NONE)
static final class Json extends LocatedString.CharacterRegion {
boolean contentNonBmp;
boolean contentNonBmpIsSet;
@Nullable CharOffset contentStartPosInclusive;
@Nullable CharOffset contentEndPosExclusive;
@Nullable OffsetGroup referenceStartOffsetInclusive;
@Nullable OffsetGroup referenceEndOffsetInclusive;
@JsonProperty("contentNonBmp")
public void setContentNonBmp(boolean contentNonBmp) {
this.contentNonBmp = contentNonBmp;
this.contentNonBmpIsSet = true;
}
@JsonProperty("contentStartPosInclusive")
public void setContentStartPosInclusive(CharOffset contentStartPosInclusive) {
this.contentStartPosInclusive = contentStartPosInclusive;
}
@JsonProperty("contentEndPosExclusive")
public void setContentEndPosExclusive(CharOffset contentEndPosExclusive) {
this.contentEndPosExclusive = contentEndPosExclusive;
}
@JsonProperty("referenceStartOffsetInclusive")
public void setReferenceStartOffsetInclusive(OffsetGroup referenceStartOffsetInclusive) {
this.referenceStartOffsetInclusive = referenceStartOffsetInclusive;
}
@JsonProperty("referenceEndOffsetInclusive")
public void setReferenceEndOffsetInclusive(OffsetGroup referenceEndOffsetInclusive) {
this.referenceEndOffsetInclusive = referenceEndOffsetInclusive;
}
@Override
public boolean contentNonBmp() { throw new UnsupportedOperationException(); }
@Override
public CharOffset contentStartPosInclusive() { throw new UnsupportedOperationException(); }
@Override
public CharOffset contentEndPosExclusive() { throw new UnsupportedOperationException(); }
@Override
public OffsetGroup referenceStartOffsetInclusive() { throw new UnsupportedOperationException(); }
@Override
public OffsetGroup referenceEndOffsetInclusive() { throw new UnsupportedOperationException(); }
}
/**
* @param json A JSON-bindable data structure
* @return An immutable value type
* @deprecated Do not use this method directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonCreator
static ImmutableLocatedString.CharacterRegion fromJson(Json json) {
LocatedString.CharacterRegion.Builder builder = new LocatedString.CharacterRegion.Builder();
if (json.contentNonBmpIsSet) {
builder.contentNonBmp(json.contentNonBmp);
}
if (json.contentStartPosInclusive != null) {
builder.contentStartPosInclusive(json.contentStartPosInclusive);
}
if (json.contentEndPosExclusive != null) {
builder.contentEndPosExclusive(json.contentEndPosExclusive);
}
if (json.referenceStartOffsetInclusive != null) {
builder.referenceStartOffsetInclusive(json.referenceStartOffsetInclusive);
}
if (json.referenceEndOffsetInclusive != null) {
builder.referenceEndOffsetInclusive(json.referenceEndOffsetInclusive);
}
return (ImmutableLocatedString.CharacterRegion) builder.build();
}
private static CharacterRegion validate(ImmutableLocatedString.CharacterRegion instance) {
instance.check();
return instance;
}
/**
* Creates an immutable copy of a {@link LocatedString.CharacterRegion} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable CharacterRegion instance
*/
public static LocatedString.CharacterRegion copyOf(LocatedString.CharacterRegion instance) {
if (instance instanceof ImmutableLocatedString.CharacterRegion) {
return (ImmutableLocatedString.CharacterRegion) instance;
}
return new LocatedString.CharacterRegion.Builder()
.from(instance)
.build();
}
/**
* Builds instances of type {@link LocatedString.CharacterRegion CharacterRegion}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
*
{@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@NotThreadSafe
static class Builder {
private static final long INIT_BIT_CONTENT_NON_BMP = 0x1L;
private static final long INIT_BIT_CONTENT_START_POS_INCLUSIVE = 0x2L;
private static final long INIT_BIT_CONTENT_END_POS_EXCLUSIVE = 0x4L;
private static final long INIT_BIT_REFERENCE_START_OFFSET_INCLUSIVE = 0x8L;
private static final long INIT_BIT_REFERENCE_END_OFFSET_INCLUSIVE = 0x10L;
private long initBits = 0x1fL;
private boolean contentNonBmp;
private @Nullable CharOffset contentStartPosInclusive;
private @Nullable CharOffset contentEndPosExclusive;
private @Nullable OffsetGroup referenceStartOffsetInclusive;
private @Nullable OffsetGroup referenceEndOffsetInclusive;
/**
* Creates a builder for {@link LocatedString.CharacterRegion CharacterRegion} instances.
*/
Builder() {
if (!(this instanceof LocatedString.CharacterRegion.Builder)) {
throw new UnsupportedOperationException("Use: new LocatedString.CharacterRegion.Builder()");
}
}
/**
* Fill a builder with attribute values from the provided {@code CharacterRegion} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final LocatedString.CharacterRegion.Builder from(LocatedString.CharacterRegion instance) {
Preconditions.checkNotNull(instance, "instance");
contentNonBmp(instance.contentNonBmp());
contentStartPosInclusive(instance.contentStartPosInclusive());
contentEndPosExclusive(instance.contentEndPosExclusive());
referenceStartOffsetInclusive(instance.referenceStartOffsetInclusive());
referenceEndOffsetInclusive(instance.referenceEndOffsetInclusive());
return (LocatedString.CharacterRegion.Builder) this;
}
/**
* Initializes the value for the {@link LocatedString.CharacterRegion#contentNonBmp() contentNonBmp} attribute.
* @param contentNonBmp The value for contentNonBmp
* @return {@code this} builder for use in a chained invocation
*/
public final LocatedString.CharacterRegion.Builder contentNonBmp(boolean contentNonBmp) {
this.contentNonBmp = contentNonBmp;
initBits &= ~INIT_BIT_CONTENT_NON_BMP;
return (LocatedString.CharacterRegion.Builder) this;
}
/**
* Initializes the value for the {@link LocatedString.CharacterRegion#contentStartPosInclusive() contentStartPosInclusive} attribute.
* @param contentStartPosInclusive The value for contentStartPosInclusive
* @return {@code this} builder for use in a chained invocation
*/
public final LocatedString.CharacterRegion.Builder contentStartPosInclusive(CharOffset contentStartPosInclusive) {
this.contentStartPosInclusive = Preconditions.checkNotNull(contentStartPosInclusive, "contentStartPosInclusive");
initBits &= ~INIT_BIT_CONTENT_START_POS_INCLUSIVE;
return (LocatedString.CharacterRegion.Builder) this;
}
/**
* Initializes the value for the {@link LocatedString.CharacterRegion#contentEndPosExclusive() contentEndPosExclusive} attribute.
* @param contentEndPosExclusive The value for contentEndPosExclusive
* @return {@code this} builder for use in a chained invocation
*/
public final LocatedString.CharacterRegion.Builder contentEndPosExclusive(CharOffset contentEndPosExclusive) {
this.contentEndPosExclusive = Preconditions.checkNotNull(contentEndPosExclusive, "contentEndPosExclusive");
initBits &= ~INIT_BIT_CONTENT_END_POS_EXCLUSIVE;
return (LocatedString.CharacterRegion.Builder) this;
}
/**
* Initializes the value for the {@link LocatedString.CharacterRegion#referenceStartOffsetInclusive() referenceStartOffsetInclusive} attribute.
* @param referenceStartOffsetInclusive The value for referenceStartOffsetInclusive
* @return {@code this} builder for use in a chained invocation
*/
public final LocatedString.CharacterRegion.Builder referenceStartOffsetInclusive(OffsetGroup referenceStartOffsetInclusive) {
this.referenceStartOffsetInclusive = Preconditions.checkNotNull(referenceStartOffsetInclusive, "referenceStartOffsetInclusive");
initBits &= ~INIT_BIT_REFERENCE_START_OFFSET_INCLUSIVE;
return (LocatedString.CharacterRegion.Builder) this;
}
/**
* Initializes the value for the {@link LocatedString.CharacterRegion#referenceEndOffsetInclusive() referenceEndOffsetInclusive} attribute.
* @param referenceEndOffsetInclusive The value for referenceEndOffsetInclusive
* @return {@code this} builder for use in a chained invocation
*/
public final LocatedString.CharacterRegion.Builder referenceEndOffsetInclusive(OffsetGroup referenceEndOffsetInclusive) {
this.referenceEndOffsetInclusive = Preconditions.checkNotNull(referenceEndOffsetInclusive, "referenceEndOffsetInclusive");
initBits &= ~INIT_BIT_REFERENCE_END_OFFSET_INCLUSIVE;
return (LocatedString.CharacterRegion.Builder) this;
}
/**
* Builds a new {@link LocatedString.CharacterRegion CharacterRegion}.
* @return An immutable instance of CharacterRegion
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public LocatedString.CharacterRegion build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return ImmutableLocatedString.CharacterRegion.validate(new ImmutableLocatedString.CharacterRegion(
contentNonBmp,
contentStartPosInclusive,
contentEndPosExclusive,
referenceStartOffsetInclusive,
referenceEndOffsetInclusive));
}
private String formatRequiredAttributesMessage() {
List attributes = Lists.newArrayList();
if ((initBits & INIT_BIT_CONTENT_NON_BMP) != 0) attributes.add("contentNonBmp");
if ((initBits & INIT_BIT_CONTENT_START_POS_INCLUSIVE) != 0) attributes.add("contentStartPosInclusive");
if ((initBits & INIT_BIT_CONTENT_END_POS_EXCLUSIVE) != 0) attributes.add("contentEndPosExclusive");
if ((initBits & INIT_BIT_REFERENCE_START_OFFSET_INCLUSIVE) != 0) attributes.add("referenceStartOffsetInclusive");
if ((initBits & INIT_BIT_REFERENCE_END_OFFSET_INCLUSIVE) != 0) attributes.add("referenceEndOffsetInclusive");
return "Cannot build CharacterRegion, some of required attributes are not set " + attributes;
}
}
}
}