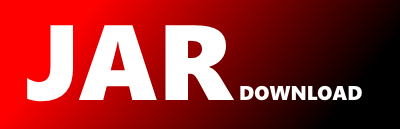
edu.isi.nlp.strings.ImmutableOffsetCalculator Maven / Gradle / Ivy
package edu.isi.nlp.strings;
import com.google.common.base.MoreObjects;
import com.google.common.base.Preconditions;
import com.google.common.collect.Lists;
import com.google.common.primitives.Booleans;
import java.util.ArrayList;
import javax.annotation.Generated;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
/**
* Immutable implementation of {@link OffsetCalculator}.
*
* Use the builder to create immutable instances:
* {@code new OffsetCalculator.Builder()}.
*/
@SuppressWarnings({"all"})
@ParametersAreNonnullByDefault
@Generated({"Immutables.generator", "OffsetCalculator"})
@Immutable
final class ImmutableOffsetCalculator extends OffsetCalculator {
private final boolean calculateEDTOffsetsByACERules;
private final boolean computeUtf8ByteOffsets;
private ImmutableOffsetCalculator(ImmutableOffsetCalculator.Builder builder) {
if (builder.calculateEDTOffsetsByACERulesIsSet()) {
initShim.calculateEDTOffsetsByACERules(builder.calculateEDTOffsetsByACERules);
}
if (builder.computeUtf8ByteOffsetsIsSet()) {
initShim.computeUtf8ByteOffsets(builder.computeUtf8ByteOffsets);
}
this.calculateEDTOffsetsByACERules = initShim.calculateEDTOffsetsByACERules();
this.computeUtf8ByteOffsets = initShim.computeUtf8ByteOffsets();
this.initShim = null;
}
private ImmutableOffsetCalculator(boolean calculateEDTOffsetsByACERules, boolean computeUtf8ByteOffsets) {
this.calculateEDTOffsetsByACERules = calculateEDTOffsetsByACERules;
this.computeUtf8ByteOffsets = computeUtf8ByteOffsets;
this.initShim = null;
}
private static final int STAGE_INITIALIZING = -1;
private static final int STAGE_UNINITIALIZED = 0;
private static final int STAGE_INITIALIZED = 1;
private transient volatile InitShim initShim = new InitShim();
private final class InitShim {
private boolean calculateEDTOffsetsByACERules;
private int calculateEDTOffsetsByACERulesStage;
boolean calculateEDTOffsetsByACERules() {
if (calculateEDTOffsetsByACERulesStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (calculateEDTOffsetsByACERulesStage == STAGE_UNINITIALIZED) {
calculateEDTOffsetsByACERulesStage = STAGE_INITIALIZING;
this.calculateEDTOffsetsByACERules = ImmutableOffsetCalculator.super.calculateEDTOffsetsByACERules();
calculateEDTOffsetsByACERulesStage = STAGE_INITIALIZED;
}
return this.calculateEDTOffsetsByACERules;
}
void calculateEDTOffsetsByACERules(boolean calculateEDTOffsetsByACERules) {
this.calculateEDTOffsetsByACERules = calculateEDTOffsetsByACERules;
calculateEDTOffsetsByACERulesStage = STAGE_INITIALIZED;
}
private boolean computeUtf8ByteOffsets;
private int computeUtf8ByteOffsetsStage;
boolean computeUtf8ByteOffsets() {
if (computeUtf8ByteOffsetsStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (computeUtf8ByteOffsetsStage == STAGE_UNINITIALIZED) {
computeUtf8ByteOffsetsStage = STAGE_INITIALIZING;
this.computeUtf8ByteOffsets = ImmutableOffsetCalculator.super.computeUtf8ByteOffsets();
computeUtf8ByteOffsetsStage = STAGE_INITIALIZED;
}
return this.computeUtf8ByteOffsets;
}
void computeUtf8ByteOffsets(boolean computeUtf8ByteOffsets) {
this.computeUtf8ByteOffsets = computeUtf8ByteOffsets;
computeUtf8ByteOffsetsStage = STAGE_INITIALIZED;
}
private String formatInitCycleMessage() {
ArrayList attributes = Lists.newArrayList();
if (calculateEDTOffsetsByACERulesStage == STAGE_INITIALIZING) attributes.add("calculateEDTOffsetsByACERules");
if (computeUtf8ByteOffsetsStage == STAGE_INITIALIZING) attributes.add("computeUtf8ByteOffsets");
return "Cannot build OffsetCalculator, attribute initializers form cycle" + attributes;
}
}
/**
* @return The value of the {@code calculateEDTOffsetsByACERules} attribute
*/
@Override
public boolean calculateEDTOffsetsByACERules() {
InitShim shim = this.initShim;
return shim != null
? shim.calculateEDTOffsetsByACERules()
: this.calculateEDTOffsetsByACERules;
}
/**
* @return The value of the {@code computeUtf8ByteOffsets} attribute
*/
@Override
public boolean computeUtf8ByteOffsets() {
InitShim shim = this.initShim;
return shim != null
? shim.computeUtf8ByteOffsets()
: this.computeUtf8ByteOffsets;
}
/**
* Copy the current immutable object by setting a value for the {@link OffsetCalculator#calculateEDTOffsetsByACERules() calculateEDTOffsetsByACERules} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for calculateEDTOffsetsByACERules
* @return A modified copy of the {@code this} object
*/
public final ImmutableOffsetCalculator withCalculateEDTOffsetsByACERules(boolean value) {
if (this.calculateEDTOffsetsByACERules == value) return this;
return validate(new ImmutableOffsetCalculator(value, this.computeUtf8ByteOffsets));
}
/**
* Copy the current immutable object by setting a value for the {@link OffsetCalculator#computeUtf8ByteOffsets() computeUtf8ByteOffsets} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for computeUtf8ByteOffsets
* @return A modified copy of the {@code this} object
*/
public final ImmutableOffsetCalculator withComputeUtf8ByteOffsets(boolean value) {
if (this.computeUtf8ByteOffsets == value) return this;
return validate(new ImmutableOffsetCalculator(this.calculateEDTOffsetsByACERules, value));
}
/**
* This instance is equal to all instances of {@code ImmutableOffsetCalculator} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableOffsetCalculator
&& equalTo((ImmutableOffsetCalculator) another);
}
private boolean equalTo(ImmutableOffsetCalculator another) {
return calculateEDTOffsetsByACERules == another.calculateEDTOffsetsByACERules
&& computeUtf8ByteOffsets == another.computeUtf8ByteOffsets;
}
/**
* Computes a hash code from attributes: {@code calculateEDTOffsetsByACERules}, {@code computeUtf8ByteOffsets}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 31;
h = h * 17 + Booleans.hashCode(calculateEDTOffsetsByACERules);
h = h * 17 + Booleans.hashCode(computeUtf8ByteOffsets);
return h;
}
/**
* Prints the immutable value {@code OffsetCalculator} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return MoreObjects.toStringHelper("OffsetCalculator")
.omitNullValues()
.add("calculateEDTOffsetsByACERules", calculateEDTOffsetsByACERules)
.add("computeUtf8ByteOffsets", computeUtf8ByteOffsets)
.toString();
}
private static ImmutableOffsetCalculator validate(ImmutableOffsetCalculator instance) {
instance.check();
return instance;
}
/**
* Creates an immutable copy of a {@link OffsetCalculator} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable OffsetCalculator instance
*/
public static OffsetCalculator copyOf(OffsetCalculator instance) {
if (instance instanceof ImmutableOffsetCalculator) {
return (ImmutableOffsetCalculator) instance;
}
return new OffsetCalculator.Builder()
.from(instance)
.build();
}
/**
* Builds instances of type {@link OffsetCalculator OffsetCalculator}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@NotThreadSafe
static class Builder {
private static final long OPT_BIT_CALCULATE_E_D_T_OFFSETS_BY_A_C_E_RULES = 0x1L;
private static final long OPT_BIT_COMPUTE_UTF8_BYTE_OFFSETS = 0x2L;
private long optBits;
private boolean calculateEDTOffsetsByACERules;
private boolean computeUtf8ByteOffsets;
/**
* Creates a builder for {@link OffsetCalculator OffsetCalculator} instances.
*/
Builder() {
if (!(this instanceof OffsetCalculator.Builder)) {
throw new UnsupportedOperationException("Use: new OffsetCalculator.Builder()");
}
}
/**
* Fill a builder with attribute values from the provided {@code OffsetCalculator} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final OffsetCalculator.Builder from(OffsetCalculator instance) {
Preconditions.checkNotNull(instance, "instance");
calculateEDTOffsetsByACERules(instance.calculateEDTOffsetsByACERules());
computeUtf8ByteOffsets(instance.computeUtf8ByteOffsets());
return (OffsetCalculator.Builder) this;
}
/**
* Initializes the value for the {@link OffsetCalculator#calculateEDTOffsetsByACERules() calculateEDTOffsetsByACERules} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link OffsetCalculator#calculateEDTOffsetsByACERules() calculateEDTOffsetsByACERules}.
* @param calculateEDTOffsetsByACERules The value for calculateEDTOffsetsByACERules
* @return {@code this} builder for use in a chained invocation
*/
public final OffsetCalculator.Builder calculateEDTOffsetsByACERules(boolean calculateEDTOffsetsByACERules) {
this.calculateEDTOffsetsByACERules = calculateEDTOffsetsByACERules;
optBits |= OPT_BIT_CALCULATE_E_D_T_OFFSETS_BY_A_C_E_RULES;
return (OffsetCalculator.Builder) this;
}
/**
* Initializes the value for the {@link OffsetCalculator#computeUtf8ByteOffsets() computeUtf8ByteOffsets} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link OffsetCalculator#computeUtf8ByteOffsets() computeUtf8ByteOffsets}.
* @param computeUtf8ByteOffsets The value for computeUtf8ByteOffsets
* @return {@code this} builder for use in a chained invocation
*/
public final OffsetCalculator.Builder computeUtf8ByteOffsets(boolean computeUtf8ByteOffsets) {
this.computeUtf8ByteOffsets = computeUtf8ByteOffsets;
optBits |= OPT_BIT_COMPUTE_UTF8_BYTE_OFFSETS;
return (OffsetCalculator.Builder) this;
}
/**
* Builds a new {@link OffsetCalculator OffsetCalculator}.
* @return An immutable instance of OffsetCalculator
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public OffsetCalculator build() {
return ImmutableOffsetCalculator.validate(new ImmutableOffsetCalculator(this));
}
private boolean calculateEDTOffsetsByACERulesIsSet() {
return (optBits & OPT_BIT_CALCULATE_E_D_T_OFFSETS_BY_A_C_E_RULES) != 0;
}
private boolean computeUtf8ByteOffsetsIsSet() {
return (optBits & OPT_BIT_COMPUTE_UTF8_BYTE_OFFSETS) != 0;
}
}
}